return
5. Write a function which will find the average of all elements of an array of integers. Pass an array and a dimension (the number of elements), and return the value of the average as a float ( the return value). Test this function in the main

A function which will find the average of all elements of an array of integers.
float average(int array[], int dimension)
{
//declare local variable avg of float type
float avg;
//declare integer type variable sum
int sum=0;
//for loop run until dimension
for(int i=0; i<dimension; i++)
{
//compute sum
sum=sum+array[i];
}
//compute average
avg=sum/dimension;
//retrun avg
return avg;
}
The following program is written in the C++ programming language.
Program:
//included header file
#include <iostream>
//included namespace
using namespace std;
//function which will find the average
float average(int array[], int dimension)
{
//declare local variable avg of float type
float avg;
//declare integer type variable sum
int sum=0;
//for loop run until dimension
for(int i=0; i<dimension; i++)
{
//compute sum
sum=sum+array[i];
}
//compute average
avg=sum/dimension;
//retrun avg
return avg;
}
//main function
int main ()
{
//declared array with given elements
int array[]={5, 7, 3, 5, 6, 4};
//declared flaot type variable avg
float avg;
//declared variable dimension of integer type
int dimension=6;
//calling function average
avg=average(array, dimension);
//print average of all elements of an array of integers
cout<<"The average of all elements of an array of integers is:"<<avg;
return 0;
}
Step by step
Solved in 3 steps with 1 images

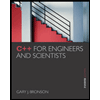
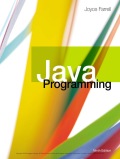
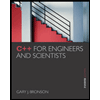
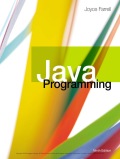