Rewrite the program with a little bit of changes in the codes... #include int READING (); int ADDING (int NUM1, int NUM2); int SUBSTRACT (int NUM1, int NUM2); int MULTIPLY (int NUM1, int NUM2); float DIVIDE (int NUM1, int NUM2); void PRINT (int NUM1, int NUM2, int TOTAL, int SUBS, int MUL, float DIV); int main() { int NUM1, NUM2, TOTAL, SUBS, MUL; float DIV; NUM1 = READING (); NUM2 = READING (); TOTAL = ADDING (NUM1, NUM2); SUBS = SUBSTRACT (NUM1, NUM2); MUL = MULTIPLY (NUM1, NUM2); DIV = DIVIDE (NUM1, NUM2); PRINT (NUM1, NUM2, TOTAL, SUBS, MUL, DIV); return 0; } int READING () { int NUM; printf("Please enter a number "); scanf("%d", &NUM); return NUM; } int ADDING (int NUMBER1, int NUMBER2) { int TOT; TOT = NUMBER1 + NUMBER2; return TOT; } int SUBSTRACT (int NUMBER1, int NUMBER2) { int SUB; SUB = NUMBER1 - NUMBER2; return SUB; } int MULTIPLY (int NUMBER1, int NUMBER2) { int MUL; MUL = NUMBER1 * NUMBER2; return MUL; } float DIVIDE (int NUMBER1, int NUMBER2) { float DIV; DIV = float(NUMBER1) / float(NUMBER2); return DIV; } void PRINT (int NUM1, int NUM2, int TOTAL, int SUBS, int MUL, float DIV) { printf("\n%d + %d = %d", NUM1, NUM2, TOTAL); printf("\n%d - %d = %d", NUM1, NUM2, SUBS); printf("\n%d * %d = %d", NUM1, NUM2, MUL); printf("\n%d / %d = %f", NUM1, NUM2, DIV); return; }
Rewrite the program with a little bit of changes in the codes...
#include <stdio.h>
int READING ();
int ADDING (int NUM1, int NUM2);
int SUBSTRACT (int NUM1, int NUM2);
int MULTIPLY (int NUM1, int NUM2);
float DIVIDE (int NUM1, int NUM2);
void PRINT (int NUM1, int NUM2, int TOTAL,
int SUBS, int MUL, float DIV);
int main()
{
int NUM1, NUM2, TOTAL, SUBS, MUL;
float DIV;
NUM1 = READING ();
NUM2 = READING ();
TOTAL = ADDING (NUM1, NUM2);
SUBS = SUBSTRACT (NUM1, NUM2);
MUL = MULTIPLY (NUM1, NUM2);
DIV = DIVIDE (NUM1, NUM2);
PRINT (NUM1, NUM2, TOTAL, SUBS, MUL, DIV);
return 0;
}
int READING ()
{
int NUM;
printf("Please enter a number ");
scanf("%d", &NUM);
return NUM;
}
int ADDING (int NUMBER1, int NUMBER2)
{
int TOT;
TOT = NUMBER1 + NUMBER2;
return TOT;
}
int SUBSTRACT (int NUMBER1, int NUMBER2)
{
int SUB;
SUB = NUMBER1 - NUMBER2;
return SUB;
}
int MULTIPLY (int NUMBER1, int NUMBER2)
{
int MUL;
MUL = NUMBER1 * NUMBER2;
return MUL;
}
float DIVIDE (int NUMBER1, int NUMBER2)
{
float DIV;
DIV = float(NUMBER1) / float(NUMBER2);
return DIV;
}
void PRINT (int NUM1, int NUM2, int TOTAL,
int SUBS, int MUL, float DIV)
{
printf("\n%d + %d = %d", NUM1, NUM2, TOTAL);
printf("\n%d - %d = %d", NUM1, NUM2, SUBS);
printf("\n%d * %d = %d", NUM1, NUM2, MUL);
printf("\n%d / %d = %f", NUM1, NUM2, DIV);
return;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

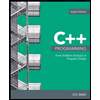
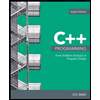