Sample Input 0 Sample Output 0 Enter the number of students: Average weight of all students is 49600.0 g Average energy of all students is 50.0% Average happiness of all students is 65.0%
Sample Input 0 Sample Output 0 Enter the number of students: Average weight of all students is 49600.0 g Average energy of all students is 50.0% Average happiness of all students is 65.0%
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Kindly refer to the pictures for the instructions
Beginner Level Code:
class Student:
def __init__(self, student_number: int, name: str, weight: float):
self.student_number = student_number
self.name = name
self.weight = weight # in kg
def eat(self, food: str):
# TODO: insert appropriate code here
self.weight += 0.50
print(f"{self.name} ate {food}.")
def exercise(self, exercise: str):
# TODO: insert appropriate code here
self.weight -= 0.50
print(f"{self.name} did {exercise}.")
def get_weight_in_grams(self):
# TODO: insert appropriate code here
return (self.weight * 1000)
# TODO: once done, remove line of code below
#pass
class EEE111Student(Student):
energy_level = 50
happiness_level = 50
def code_python(self):
# TODO: insert appropriate code here
self.energy_level -= 10
self.happiness_level += 10
# TODO: once done, remove line of code below
#pass
def eat(self, food: str):
# TODO: insert appropriate code here
Student.eat(self, food)
self.energy_level += 10
self.happiness_level += 10
# TODO: once done, remove line of code below
#pass
def exercise(self, food: str):
# TODO: insert appropriate code here
Student.exercise(self, food)
self.energy_level -= 10
self.happiness_level += 10
# TODO: once done, remove line of code below
#pass
def display_state(self):
print(
f"Displaying stats for {self.name}, student number: {self.student_number}."
)
print(
f"Energy level: {self.energy_level}, happiness level: {self.happiness_level}, weight: {self.weight}"
)
if __name__ == "__main__":
other_student = Student(student_number=1, name="Juan", weight=50)
eee_student = EEE111Student(student_number=1, name="Joe", weight=50)
other_student.eat("popcorn")
eee_student.eat("rice")
eee_student.eat("adobo")
other_student.exercise("badminton")
eee_student.exercise("volleyball")
eee_student.code_python()
print(f"{other_student.name} weight: {other_student.get_weight_in_grams()} grams")
print(f"{eee_student.name} weight: {eee_student.get_weight_in_grams()} grams")
eee_student.display_state()
![Using the code in the Beginner Level, modify the Student class to remove the print statements inside the
eat and exercise methods. This is to remove clutter as you implement the requirements for this level.
In the previous level, all students had a fixed amount of energy/happiness/weight added regardless of the
type of food they eat. However, that's not what happens in reality. To represent eating more accurately, let us
create a Food class.
Part 1:
To create the Food class, you must follow the specifications below:
• Upon initialization, the object will have the following attributes (as specified by the user)
weight (weight of the food in kg)
energy_add (amount of energy it will add once consumed)
• happiness_add (amount of happiness it will add once consumed)
• Example usage: Food (weight=1, energy_add=1, happiness_add=1)
Part 2:
To make the eating behavior closer to reality, you should modify the Student and the EEE11iStudent
classes such that:
• Instead of accepting a string (as food) in the eat method, the method should accept a Food object (e.g.,
student.eat(food=Food (...)))
Once a Student/EEE111Student object eats a Food object,
• The added weight should be the Food object's weight
• The added energy should be the Food object's energy_add
• The added happiness should be the Food object's happiness_add
Part 3:
After updating the student and EEE 111 student behavior, let's try to simulate a small group of EEE 111
students. Modify the main code to do the following:
• Accept a positive integer from the user (assume the input is correct, so no need to do input validation) that
determines the number of students in the class
• Create a number of EEE 111 students specified in the input.
For each student:
student_number should be from 1 to num_students (inclusive) where each student should have a
unique student_number
name should be "StudentN" where N is the student number
• weight should be 50 + 0.5 * (student number-1) in kilograms
• The code should look something like:
num_students = int(input("Enter the number of students: "))
students = []
for idx in range (num_students):
# TODO: create new_student here
students.append (new_student)
Part 4:
As the teacher, you have given tasks to the students.
Add the following lines in your code (assuming Food class was properly created):
food_choices = {
"papaya": Food (weight=0.10, energy_add=10, happiness_add=5),
"corn": Food (weight=0.20, energy_add=5, happiness_add=6),
• For students with even student numbers, they should do the following:
• Eat "corn" in the given food_choices
• Code python
• For students with odd student numbers, they should do the following:
• Eat "papaya" in the given food_choices
• Exercise with "run" as input
• For students with student numbers divisible by 7, they should do the following:
• Eat “papaya" in the given food_choices
• Eat "corn" in the given food_choices
• For students with student numbers divisible by 5, they should do the following:
• Code python
• Exercise with “run" as input
• After the students have finished their respective tasks, you should get the following
• The average weight of all students in grams
• The average energy of all students
• The average happiness of all students](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd2677216-7cf7-42e8-b32b-5f76635a2e9c%2F8ebcedb9-6acf-4985-abfb-803151592169%2Fk0cwovf_processed.png&w=3840&q=75)
Transcribed Image Text:Using the code in the Beginner Level, modify the Student class to remove the print statements inside the
eat and exercise methods. This is to remove clutter as you implement the requirements for this level.
In the previous level, all students had a fixed amount of energy/happiness/weight added regardless of the
type of food they eat. However, that's not what happens in reality. To represent eating more accurately, let us
create a Food class.
Part 1:
To create the Food class, you must follow the specifications below:
• Upon initialization, the object will have the following attributes (as specified by the user)
weight (weight of the food in kg)
energy_add (amount of energy it will add once consumed)
• happiness_add (amount of happiness it will add once consumed)
• Example usage: Food (weight=1, energy_add=1, happiness_add=1)
Part 2:
To make the eating behavior closer to reality, you should modify the Student and the EEE11iStudent
classes such that:
• Instead of accepting a string (as food) in the eat method, the method should accept a Food object (e.g.,
student.eat(food=Food (...)))
Once a Student/EEE111Student object eats a Food object,
• The added weight should be the Food object's weight
• The added energy should be the Food object's energy_add
• The added happiness should be the Food object's happiness_add
Part 3:
After updating the student and EEE 111 student behavior, let's try to simulate a small group of EEE 111
students. Modify the main code to do the following:
• Accept a positive integer from the user (assume the input is correct, so no need to do input validation) that
determines the number of students in the class
• Create a number of EEE 111 students specified in the input.
For each student:
student_number should be from 1 to num_students (inclusive) where each student should have a
unique student_number
name should be "StudentN" where N is the student number
• weight should be 50 + 0.5 * (student number-1) in kilograms
• The code should look something like:
num_students = int(input("Enter the number of students: "))
students = []
for idx in range (num_students):
# TODO: create new_student here
students.append (new_student)
Part 4:
As the teacher, you have given tasks to the students.
Add the following lines in your code (assuming Food class was properly created):
food_choices = {
"papaya": Food (weight=0.10, energy_add=10, happiness_add=5),
"corn": Food (weight=0.20, energy_add=5, happiness_add=6),
• For students with even student numbers, they should do the following:
• Eat "corn" in the given food_choices
• Code python
• For students with odd student numbers, they should do the following:
• Eat "papaya" in the given food_choices
• Exercise with "run" as input
• For students with student numbers divisible by 7, they should do the following:
• Eat “papaya" in the given food_choices
• Eat "corn" in the given food_choices
• For students with student numbers divisible by 5, they should do the following:
• Code python
• Exercise with “run" as input
• After the students have finished their respective tasks, you should get the following
• The average weight of all students in grams
• The average energy of all students
• The average happiness of all students
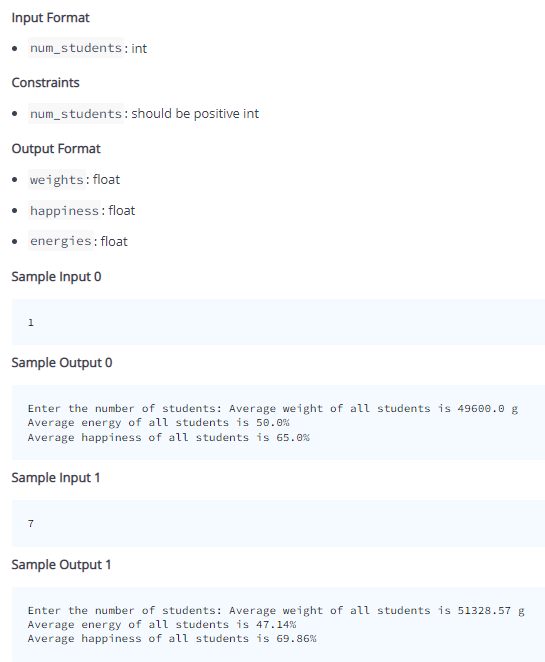
Transcribed Image Text:Input Format
• num_students: int
Constraints
• num_students: should be positive int
Output Format
weights: float
• happiness: float
• energies: float
Sample Input 0
1
Sample Output 0
Enter the number of students: Average weight of all students is 49600.0 g
Average energy of all students is 50.0%
Average happiness of all students is
Sample Input 1
7
Sample Output 1
Enter the number of students: Average weight of all students is 51328.57 g
Average energy of all students is 47.14%
Average happiness of all students is 69.86%
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
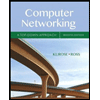
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
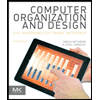
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
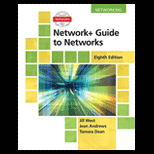
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
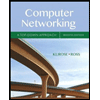
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
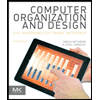
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
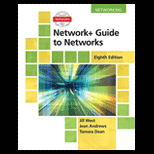
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
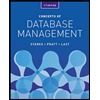
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
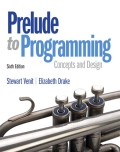
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
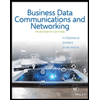
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY