Sentence.java (interface) /** * This is a Interface for Sentence. */ public interface Sentence { /** * Returns number of Words. * * @return - an int. */ int getNumberOfWords(); /** * Returns Longest Word. * * @return - a String. */ String longestWord(); /** * Returns a String that represents a sentence. * * @return - a String. */ String toString(); /** * clones a sentence. * * @return - a Sentence. */ Sentence clone(); /** * merges a sentence with another. * * @return - a Sentence. */ Sentence merge(Sentence secondSentenc
For all methods in each class (labeled), how do I write the JUnit 4 test(s) to test all methods? Full code is shown below:
PunctuationNode.java (class)
public class PunctuationNode implements Sentence {
private String punctuation;
private Sentence rest;
/**
* This is a constructor for class PunctuationNode.
*
* @param punctuation - a String.
* @param rest - a String.
*/
public PunctuationNode(String punctuation, Sentence rest) {
this.punctuation = punctuation;
this.rest = rest;
}
/**
* A method to get number of words.
*
* @return - a integer.
*/
@Override
public int getNumberOfWords() {
return this.rest.getNumberOfWords();
}
/**
* A method to get longest word.
*
* @return - a integer.
*/
@Override
public String longestWord() {
return this.rest.longestWord();
}
/**
* Returns a String that represents a sentence.
*
* @return - a Sentence.
*/
@Override
public String toString() {
return this.punctuation + this.rest;
}
/**
* clones a sentence.
*
* @return - a Sentence.
*/
@Override
public Sentence clone() {
return new PunctuationNode(this.punctuation, this.rest.clone());
}
/**
* merges a sentence with another.
*
* @return - a Sentence.
*/
@Override
public Sentence merge(Sentence other) {
return new PunctuationNode(this.punctuation, this.rest.merge(other));
}
}
WordNode.java (class)
/**
* This is a class for Sentence.
*/
public class WordNode implements Sentence {
private String word;
private Sentence rest;
/**
* This is a constructor for the Sentence Class.
*
* @param word - string.
* @param rest - a Sentence.
*/
public WordNode(String word, Sentence rest) {
this.word = word;
this.rest = rest;
}
/**
* Does: Calculates the total number of words in a Sentence.
*
* @return - The total number of words from "current" + the rest of the words.
*/
@Override
public int getNumberOfWords() {
return 1 + this.rest.getNumberOfWords();
}
/**
* Does: Determines the longest word in the sentence.
*
* @return - A string of the longest word.
*/
@Override
public String longestWord() {
if (word.length() >= this.rest.longestWord().length()) {
return this.word;
}
return this.rest.longestWord();
}
/**
* Does: Adds a period to the end of the string if there none.
*
* @return - a String.
*/
@Override
public String toString() {
if (this.rest.toString().equals("")) {
// If we are at the end of the word nodes
// and check for last node is Empty Node
return this.word + ".";
}
if (rest instanceof PunctuationNode) {
return this.word + this.rest.toString();
}
return this.word + " " + this.rest.toString();
}
/**
* Does: clones a sentence.
*
* @return - a String.
*/
@Override
public Sentence clone() {
return new WordNode(this.word, this.rest.clone());
}
/**
* Does: merges a sentence.
*
* @return - a String.
*/
@Override
public Sentence merge(Sentence secondSentence) {
return new WordNode(this.word, this.rest.merge(secondSentence));
}
}
EmptyNode.java (class)
/**
* This a class for EmptyNode.
*/
public class EmptyNode implements Sentence {
/**
* Returns 0.
*
* @return - 0 words at the start.
*/
@Override
public int getNumberOfWords() {
return 0;
}
/**
* Returns empty string.
*
* @return - 0 words at the start.
*/
@Override
public String longestWord() {
return "";
}
/**
* Returns empty string.
*
* @return - 0 words at the start.
*/
@Override
public String toString() {
return "";
}
/**
* Returns sentence to merge.
*
* @return - other sentence.
*/
@Override
public Sentence merge(Sentence other) {
return other.clone();
}
/**
* Returns empty Node.
*
* @return - 0 words at start.
*/
@Override
public Sentence clone() {
return new EmptyNode();
}
}
Sentence.java (interface)
/**
* This is a Interface for Sentence.
*/
public interface Sentence {
/**
* Returns number of Words.
*
* @return - an int.
*/
int getNumberOfWords();
/**
* Returns Longest Word.
*
* @return - a String.
*/
String longestWord();
/**
* Returns a String that represents a sentence.
*
* @return - a String.
*/
String toString();
/**
* clones a sentence.
*
* @return - a Sentence.
*/
Sentence clone();
/**
* merges a sentence with another.
*
* @return - a Sentence.
*/
Sentence merge(Sentence secondSentence);
}
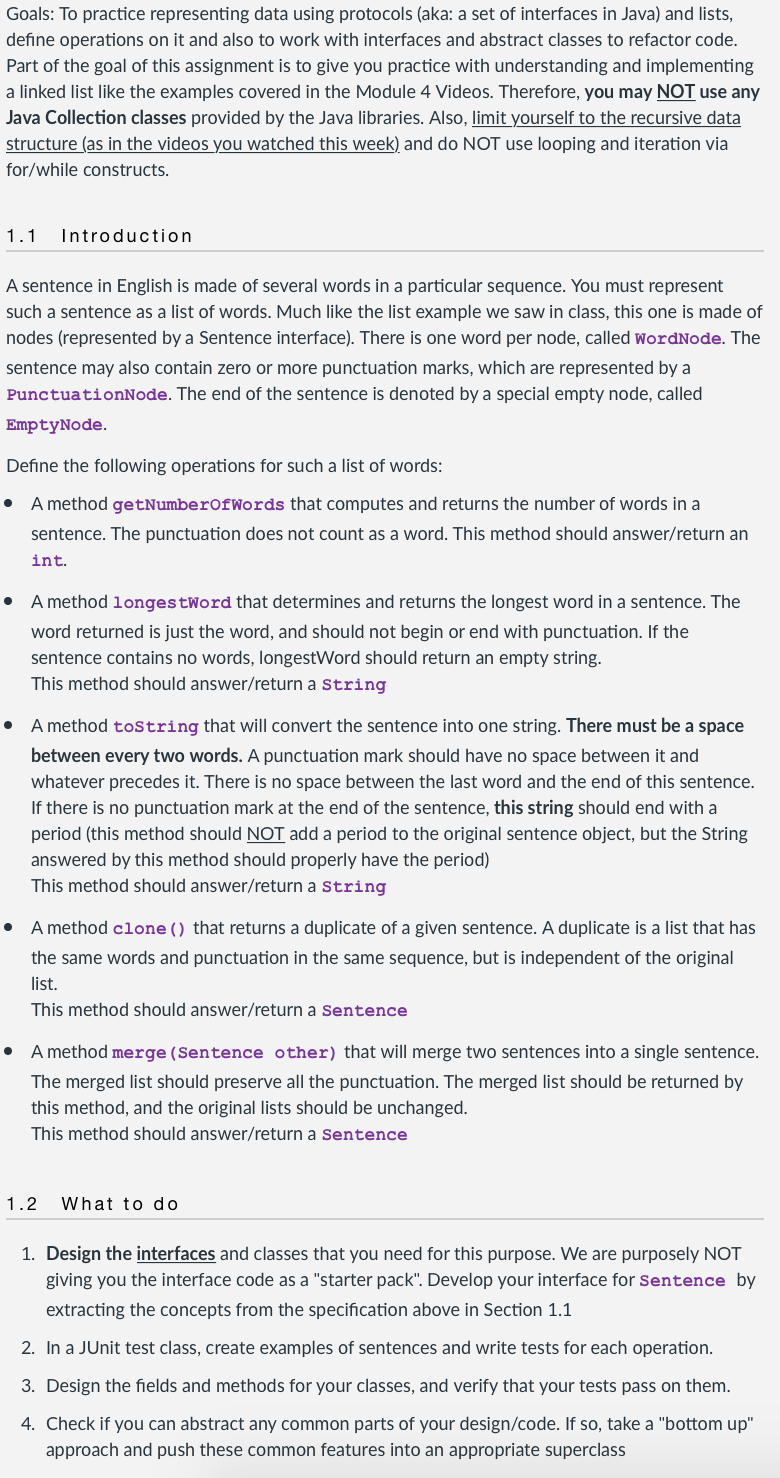

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

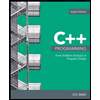
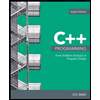