“server.c” with the following functionalities:
Complete the attached template program “server.c” with the following functionalities:
- Parent process creates 5 children processes.
- Each child process has a pipe set up for sending a string to the parent.
- Parent is monitoring data availability in any of the pipes. If it finds available pipes, it will read strings from the pipes into memory locations pointed by buf[] array elements. Parent needs to store the bytes from pipe, pip[i], into a memory pointed by buf[i].
- Once the parent finds out that all its children processes are terminated, it will convert each string (stored in memory pointed by buf[i]) into an integer and add them up to find the total sum into a variable, sum.
- Variable sum will be returned at the end of the parent().
- Parent also monitors any keyboard stroke. If a user types in ‘q’ character in the keyboard, parent needs to print a message “Quit!” and return 0 immediately. Other characters will be simply ignored by the parent, and it will continue its execution.
- You may refer to the example program in the textbook p. 166-167.
The output from your program should be:
180690
* server -- creates five children and then add numbers sent by them and print the sum */
#include <sys/time.h>
#include <sys/wait.h>
#include <unistd.h>
#include <stdlib.h>
#include <stdio.h>
#define MSGSIZE 6 char msg[5][MSGSIZE] = {"12345", "45689", "15689", "34592", "72375"};
int parent(int [5][2]);
int child(int [2], int);
int main() { int pip[5][2];
int i; /* create five communication pipes, and spawn five children */ for(i = 0; i < 5; i++) {
if(pipe(pip[i]) == -1) perror("pipe call");
switch (fork() ) { case -1: /* error */ perror("fork call");
case 0: /* child */ child(pip[i], i);
}
}
int sum = parent(pip);
printf("%d", sum);
exit(0); } /* parent sits listening on all five pipes, once it hears from all 5 pipes it will convert each string, add them up, and return its sum as an integer */
int parent(int p[5][2]) /* code for parent */ { //// buf[5][] is used to 5 strings to be received from 5 children processes!
char buf[5][MSGSIZE], ch;
fd_set set, master;
int i; int sum=0; /* close all unwanted write file descriptors */
//// Fill up this part! /* set the bit masks for the select system call */
//// Fill up this part! ////
//// /* Select is called with no timeout, it will block until an event occurs */
while(set = master, select( /* Fill up this part! */ ) > 0) {
/* we mustn't forget information,Information on standard input, * i.e. fd=0 */ /*** when a user presses 'q' character, server needs to print a message "Quit!" and return 0 immediately if a user presses other character, server needs to ignore it! ***
/ //// //// Fill up this part! ////
for(i = 0; i < 5; i++) {
//// check which pipes have data bytes available for reading //// then, read the bytes into proper target buf[] array //// bytes sent by a child (connected through pip[i]) should //// be stored into buf[i][] //// If read() fails, then use perror to print an error message and return -1 ////
//// Fill up this part! ////
} /* The server will return the sum of the numbers it reaceived from children to the main program if all its children have died */
if (waitpid (-1, NULL, WNOHANG) == -1) {
int i=0; for(i=0; i<5; i++) sum += atoi(buf[i]); return sum; } } } /* id - child identifier - from 0 through 4 */
int child(int p[2], int id) {
int count;
close(p[0]); write(p[1], msg[id], MSGSIZE); sleep(getpid() % 4); exit(0);
}

Step by step
Solved in 2 steps

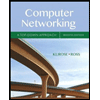
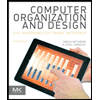
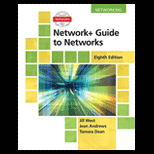
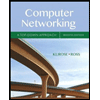
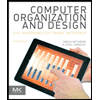
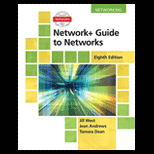
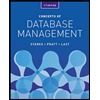
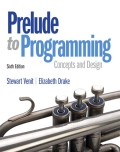
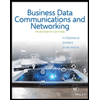