ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() method Build the ShoppingCart class with the following specifications. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to "January 1, 2016" ArrayList cartItems Default constructor Parameterized constructor which takes the customer name and date as parameters Public member methods getCustomerName() accessor getDate() accessor addItem() Adds an item to cartItems array. Has a parameter of type ItemToPurchase. Does not return anything. removeItem() Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anything. If item name cannot be found, output a message: Item not found in cart. Nothing removed. modifyItem() Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything. If item can be found (by name) in cart, check if parameter has default values for description, price, and quantity. If not, modify item in cart. If item cannot be found (by name) in cart, output a message: Item not found in cart. Nothing modified. getNumItemsInCart() Returns quantity of all items in cart. Has no parameters. getCostOfCart() Determines and returns the total cost of items in cart. Has no parameters. printTotal() Outputs total of objects in cart. If cart is empty, output a message: SHOPPING CART IS EMPTY printDescriptions() Outputs each item's description. If cart is empty, output a message: SHOPPING CART IS EMPTY Step 3: In main(), prompt the user for a customer's name and today's date. Output the name and date. Create an object of type ShoppingCart. Step 4: Implement the following menu functions in the ShoppingCartManager class printMenu() Prints the following menu of options to manipulate the shopping cart. MENU a - Add item to cart d - Remove item from cart c - Change item quantity i - Output items' descriptions o - Output shopping cart q - Quit executeMenu() Takes 3 parameters: a character representing the user's choice, a shopping cart, and a Scanner object. Performs the menu options described below in step 5, according to the user's choice. Step 5: Implement the menu options Step 5a: In main(), call printMenu() and prompt for the user's choice of menu options. Each option is represented by a single character. If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling executeMenu(). Then, print the menu and prompt for a new option. Continue until the user enters 'q'. Hint: Implement Quit before implementing other options. Step 5b: Implement "Output shopping cart" menu option in executeMenu(). Step 5c: Implement "Output items' descriptions" menu option in executeMenu(). Step 5d: Implement "Add item to cart" menu option in executeMenu(). Ex: ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: Volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2 Step 5e: Implement "Remove item from cart" menu option in executeMenu(). Ex: REMOVE ITEM FROM CART Enter name of item to remove: Chocolate Chips Step 5f: Implement "Change item quantity" menu option in executeMenu(). Hint: Make new ItemToPurchase object and use ItemToPurchase modifiers before using modifyItem() method.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
- ShoppingCart.java - Class definition
- ShoppingCartManager.java - Contains main() method
Build the ShoppingCart class with the following specifications.
- Private fields
- String customerName - Initialized in default constructor to "none"
- String currentDate - Initialized in default constructor to "January 1, 2016"
- ArrayList cartItems
- Default constructor
- Parameterized constructor which takes the customer name and date as parameters
- Public member methods
- getCustomerName() accessor
- getDate() accessor
- addItem()
- Adds an item to cartItems array. Has a parameter of type ItemToPurchase. Does not return anything.
- removeItem()
- Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anything.
- If item name cannot be found, output a message: Item not found in cart. Nothing removed.
- modifyItem()
- Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything.
- If item can be found (by name) in cart, check if parameter has default values for description, price, and quantity. If not, modify item in cart.
- If item cannot be found (by name) in cart, output a message: Item not found in cart. Nothing modified.
- getNumItemsInCart()
- Returns quantity of all items in cart. Has no parameters.
- getCostOfCart()
- Determines and returns the total cost of items in cart. Has no parameters.
- printTotal()
- Outputs total of objects in cart.
- If cart is empty, output a message: SHOPPING CART IS EMPTY
- printDescriptions()
- Outputs each item's description.
- If cart is empty, output a message: SHOPPING CART IS EMPTY
Step 3: In main(), prompt the user for a customer's name and today's date. Output the name and date. Create an object of type ShoppingCart.
Step 4: Implement the following menu functions in the ShoppingCartManager class
- printMenu()
- Prints the following menu of options to manipulate the shopping cart.
- executeMenu()
- Takes 3 parameters: a character representing the user's choice, a shopping cart, and a Scanner object. Performs the menu options described below in step 5, according to the user's choice.
Step 5: Implement the menu options
Step 5a: In main(), call printMenu() and prompt for the user's choice of menu options. Each option is represented by a single character.
If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling executeMenu(). Then, print the menu and prompt for a new option. Continue until the user enters 'q'.
Hint: Implement Quit before implementing other options.
Step 5b: Implement "Output shopping cart" menu option in executeMenu().
Step 5c: Implement "Output items' descriptions" menu option in executeMenu().
Step 5d: Implement "Add item to cart" menu option in executeMenu().
Ex:
ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: Volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2Step 5e: Implement "Remove item from cart" menu option in executeMenu().
Ex:
REMOVE ITEM FROM CART Enter name of item to remove: Chocolate ChipsStep 5f: Implement "Change item quantity" menu option in executeMenu().
Hint: Make new ItemToPurchase object and use ItemToPurchase modifiers before using modifyItem() method.
- printMenu()
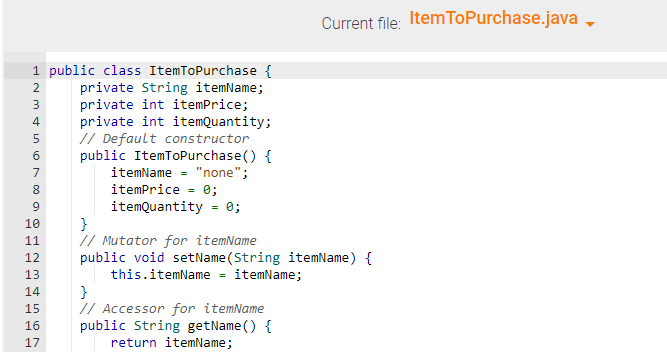
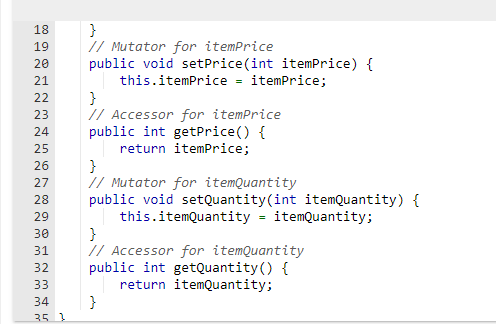

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

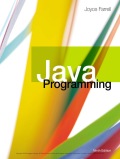
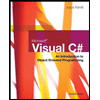
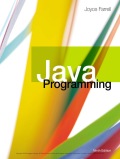
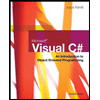