ShoppingList Class import java.util.Scanner; import java.util.LinkedList; public class ShoppingList { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); // TODO: Declare a LinkedList called shoppingList of type ListItem LinkedList shoppingList = new LinkedList(); String item; // TODO: Scan inputs (items) and add them to the shoppingList LinkedList // Read inputs until a -1 is input item = scnr.nextLine(); while (!item.equals("-1")) { shoppingList.add(item); item = scnr.nextLine(); } for (int i = 0; i < shoppingList.size(); ++i) { System.out.println(shoppingList.get(i)); } // TODO: Print the shoppingList LinkedList using the printNodeData() method //shoppingList.printNodeData(); } } ListItem Class public class ListItem { private String item; public ListItem() { item = ""; } public ListItem(String itemInit) { this.item = itemInit; } // Print this node public void printNodeData() { System.out.println(this.item); } }
Need help solving this issue. I'm not sure how to add the items in a LinkedList
ShoppingList Class
import java.util.Scanner;
import java.util.LinkedList;
public class ShoppingList {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
// TODO: Declare a LinkedList called shoppingList of type ListItem
LinkedList<ListItem> shoppingList = new LinkedList<ListItem>();
String item;
// TODO: Scan inputs (items) and add them to the shoppingList LinkedList
// Read inputs until a -1 is input
item = scnr.nextLine();
while (!item.equals("-1")) {
shoppingList.add(item);
item = scnr.nextLine();
}
for (int i = 0; i < shoppingList.size(); ++i) {
System.out.println(shoppingList.get(i));
}
// TODO: Print the shoppingList LinkedList using the printNodeData() method
//shoppingList.printNodeData();
}
}
ListItem Class
public class ListItem {
private String item;
public ListItem() {
item = "";
}
public ListItem(String itemInit) {
this.item = itemInit;
}
// Print this node
public void printNodeData() {
System.out.println(this.item);
}
}
Error Message
ShoppingList.java:18: error: incompatible types: String cannot be converted to ListItem shoppingList.add(item);

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

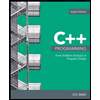
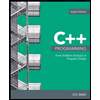