show: Lists the items that the wizard is currently carrying along with the index for each item and the weight of that item. See sample runs below. As shown in the sample this function also prints the total weight of the items along with the max weight limit of 100 lbs. Hint: You can use the sum function in Python to calculate the sum of weights. E.g. sum(weights) will return the sum of all the items in the weights list. grab_item: Add a new item while enforcing the following policy: There is a limit of 4 on the number of items the wizard can carry and limit of 100 lbs on the total weight the wizard can carry. So specifically, if the wizard is already carrying 4 items, print message that “You can't carry anymore items. Drop something first.” And return out of the function. Otherwise prompt the user for the name and the weight of the new item. Next check whether with the addition of the new item, the total weight will exceed the limit of 100 lbs. If so, print a message saying weight limit will be exceeded, so cannot add the new item and return out of the function. Otherwise add the new name and weight to the two lists. See Sample runs below. Hint: You can use the sum function in Python to calculate the sum of weights. E.g. sum(weights) will return the sum of all the items in the weights list. edit_item: Allow the user to update (only) the name of one of the items. Prompt the user for the index of the item to be edited. Validate that the index is between 1 and current count of items. If invalid, print a message to that effect and exit. Otherwise prompt the user for the new name and update the name of the item. Print that the item was updated successfully. See Sample runs below. drop_item: Prompt the user for the index of the item to be dropped. Validate that the index is between 1 and current count of items. Remove the item from both the lists. Print that the item was removed successfully. - Program that keeps track of the inventory of items a wizard is - carrying. The program allows the user to show/add/remove/ - edit the items the wizard is carrying. WEIGHT_LIMIT = 100 COUNT_LIMIT = 4 def display_title(): print("The Wizard Inventory program") print() def display_menu(): print("COMMAND MENU") print("show - Show all items") print("grab - Grab an item") print("edit - Edit an item") print("drop - Drop an item") print("exit - Exit program") print() def show(names, weights): ''' Function to show the items listed in names and weights list in the form: index name (weight lbs) At the end print the current total weight of the items and the weight limit of 100 lbs.''' pass # To be implemented def grab_item(names, weights): ''' Function to add an item to the items list. Check if the lists already have 4 items, then print a message saying cannot add more and exit. otherwise prompt the user for the name and the weight of the new item Check if with the addition of the new item the weight limit of 100 lbs will be exceeded. If so, print a message saying weight limit will be exceeded, cannot add item and exit Add the new name and weight to the two lists. ''' pass # To be implemented def edit_item(names, weights): ''' Function to edit name of an item from the two lists Prompt the user for the index of the item to be edited. Validate that the index is between 1 and count of items. If invalid index, print a message stating the index is invalid else prompt the user for the new name and update the name of the correct item. ''' pass # To be implemented def drop_item(names, weights): ''' Function to drop an item from the two lists Prompt the user for the index of the item to be dropped. Validate that the index is between 1 and count of items. Remove the item at the given index from the list. ''' pass # To be implemented def main(): display_title() display_menu() # all players start with these 3 items inventory_names = ["wooden staff", "wizard hat","cloth shoes"] inventory_weights = [30.0, 1.5, 5.3] while True: command = input("Command: ") if command == "show": show(inventory_names, inventory_weights) elif command == "grab": grab_item(inventory_names, inventory_weights) elif command == "edit": edit_item(inventory_names, inventory_weights) elif command == "drop": drop_item(inventory_names, inventory_weights) elif command == "exit": break else: print("Not a valid command. Please try again.\n") print("Bye!") if __name__ == "__main__": main()
show: Lists the items that the wizard is currently carrying along with the index for each
item and the weight of that item. See sample runs below. As shown in the sample this
function also prints the total weight of the items along with the max weight limit of 100
lbs. Hint: You can use the sum function in Python to calculate the sum of weights. E.g.
sum(weights) will return the sum of all the items in the weights list.
grab_item: Add a new item while enforcing the following policy: There is a limit of 4
on the number of items the wizard can carry and limit of 100 lbs on the total weight the
wizard can carry. So specifically, if the wizard is already carrying 4 items, print message
that “You can't carry anymore items. Drop something first.” And return out of the
function. Otherwise prompt the user for the name and the weight of the new item. Next
check whether with the addition of the new item, the total weight will exceed the limit of
100 lbs. If so, print a message saying weight limit will be exceeded, so cannot add the
new item and return out of the function. Otherwise add the new name and weight to the
two lists. See Sample runs below. Hint: You can use the sum function in Python to
calculate the sum of weights. E.g. sum(weights) will return the sum of all the items in the
weights list.
for the index of the item to be edited. Validate that the index is between 1 and current
count of items. If invalid, print a message to that effect and exit. Otherwise prompt the
user for the new name and update the name of the item. Print that the item was updated
successfully. See Sample runs below.
drop_item: Prompt the user for the index of the item to be dropped. Validate that the
index is between 1 and current count of items. Remove the item from both the lists. Print
that the item was removed successfully.
- carrying. The program allows the user to show/add/remove/
- edit the items the wizard is carrying.
WEIGHT_LIMIT = 100
COUNT_LIMIT = 4
def display_title():
print("The Wizard Inventory program")
print()
def display_menu():
print("COMMAND MENU")
print("show - Show all items")
print("grab - Grab an item")
print("edit - Edit an item")
print("drop - Drop an item")
print("exit - Exit program")
print()
def show(names, weights):
'''
Function to show the items listed in names and weights list in the form:
index name (weight lbs) At the end print the current total weight
of the items and the weight limit of 100 lbs.'''
pass # To be implemented
def grab_item(names, weights):
''' Function to add an item to the items list.
Check if the lists already have 4 items, then print
a message saying cannot add more and exit.
otherwise prompt the user for the name and the weight of the new item
Check if with the addition of the new item the weight limit
of 100 lbs will be exceeded. If so, print a message saying weight limit
will be exceeded,
cannot add item and exit
Add the new name and weight to the two lists.
'''
pass # To be implemented
def edit_item(names, weights):
''' Function to edit name of an item from the two lists
Prompt the user for the index of the item to be edited.
Validate that the index is between 1 and count of items.
If invalid index, print a message stating the index is invalid
else prompt the user for the new name and update the name of the correct
item.
'''
pass # To be implemented
def drop_item(names, weights):
''' Function to drop an item from the two lists
Prompt the user for the index of the item to be dropped.
Validate that the index is between 1 and count of items.
Remove the item at the given index from the list.
'''
pass # To be implemented
display_title()
display_menu()
# all players start with these 3 items
inventory_names = ["wooden staff", "wizard hat","cloth shoes"]
inventory_weights = [30.0, 1.5, 5.3]
while True:
command = input("Command: ")
if command == "show":
show(inventory_names, inventory_weights)
elif command == "grab":
grab_item(inventory_names, inventory_weights)
elif command == "edit":
edit_item(inventory_names, inventory_weights)
elif command == "drop":
drop_item(inventory_names, inventory_weights)
elif command == "exit":
break
else:
print("Not a valid command. Please try again.\n")
print("Bye!")
if __name__ == "__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

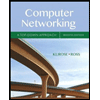
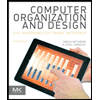
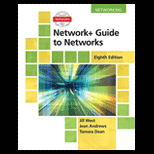
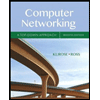
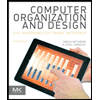
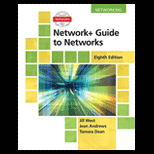
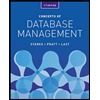
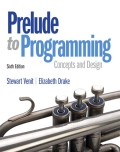
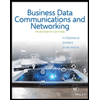