Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an argument. Complete the recursive function BinarySearch() with the following specifications: 1. Parameters: o a target integer o a vector of integers o lower and upper bounds within which the recursive call will search 2. Return value: o the index within the vector where the target is located -1 if target is not found The template provides the main program and a helper function that reads a vector from input. The algorithm begins by choosing an index midway between the lower and upper bounds. 1. If target == integers.at (index) return index 2. If lower == upper, return lower if target == integers.at (lower) else-1 to indicate not found 3. Otherwise call the function recursively on half the vector parameter: o If integers.at (index) < target, search the vector from index to upper • If integers.at (index) > target, search the vector from lower to index The vector must be ordered, but duplicates are allowed. Once the search algorithm works correctly, add the following to BinarySearch():
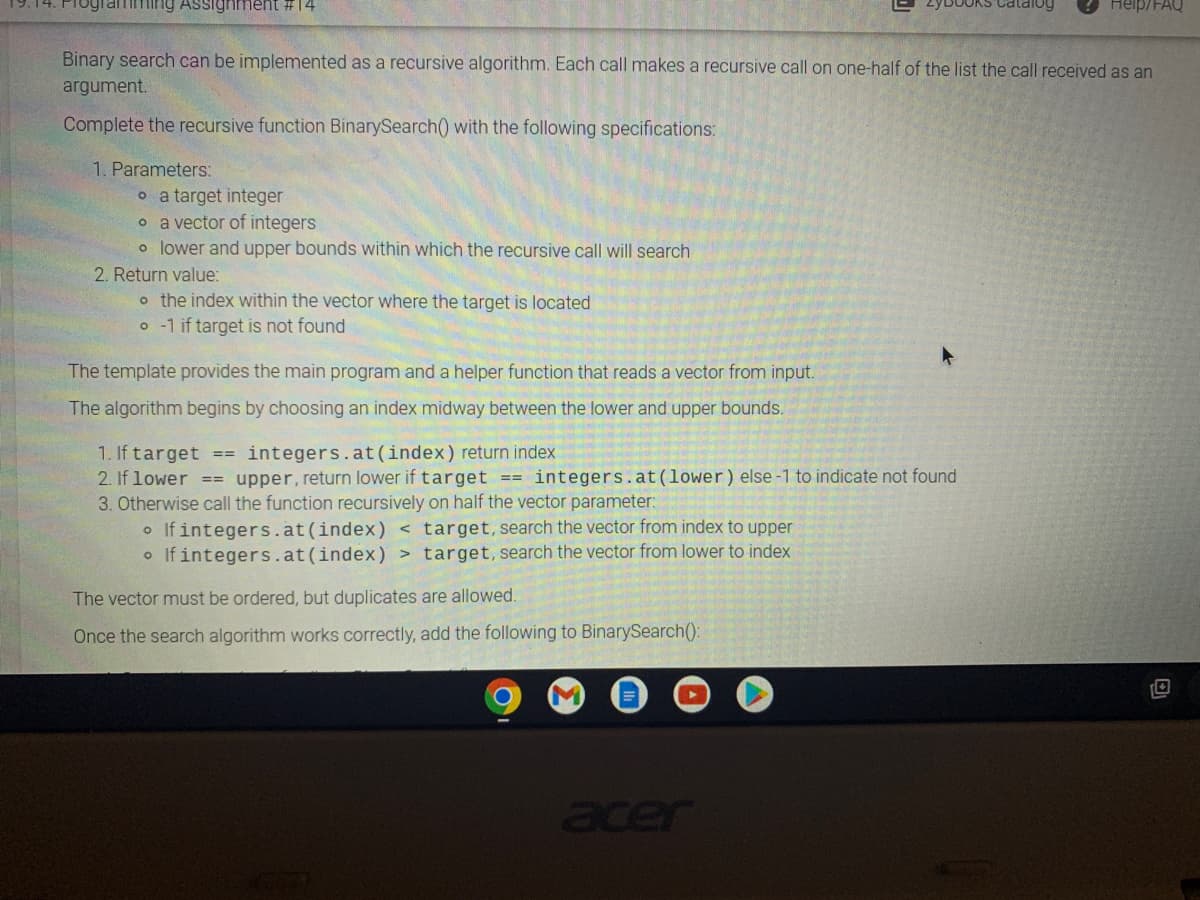

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

ok That solved the comiler issue. Now I am having an issue where it is failing certain tests but not others.
I used the code above but here it is again. I am not sure if I missed something when I corrected the code that maybe I am not seeing. also I attached a screen shot of th efailed tests.
#include <iostream>
#include <
#include <algorithm>
using namespace std;
vector<int> Readintegers() {
int size; cin >> size;
vector<int> integers(size);
for (int i = 0; i < size; ++i) {
cin>>integers[i];
}
sort(integers.begin(), integers.end()); return integers;
}
int recursions = 1;
int comparisons = 1;
int BinarySearch(int target, vector<int> integers, int lower, int upper) {
if(lower>upper)
return -1;
int index = lower + (upper - lower) / 2;
comparisons+=1;
if(integers[index]==target){
return index;
}
else if (integers[index]>target) {
recursions++;
return BinarySearch(target,integers, lower, index-1);//fixed this line
//coma is missing between target and integers
}
else{
recursions++;
return BinarySearch(target,integers, index+1,upper);
}
}
int main(int argc, char* argv[]) {
int target;
int index;
vector<int> integers = Readintegers();
cin >> target;
index = BinarySearch(target, integers, 0, integers.size() - 1);
printf("index: %d, recursions: %d, comparisons: %d\n",
index, recursions, comparisons);
return 0;
}
so. I input:
#include <iostream>
#include <
#include <algorithm>
using namespace std;
vector<int> Readintegers() {
int size; cin >> size;
vector<int> integers(size);
for (int i = 0; i < size; ++i) {
}
sort(integers.begin(), integers.end()); return integers;
}
int recursions = 1;
int comparisons = 1;
int BinarySearch(int target, vector<int> integers, int lower, int upper) {
if(lower-upper)return -1;
int index = lower + (upper - lower) / 2;
comparisons+=1;
if(integers[index]==target){
return index;
}
else if (integers[index]>target) {
recursions++;
return BinarySearch(target integers, lower, index-1);
}
else{
recursions++;
return BinarySearch(target,integers, index+1,upper);
}
}
int main(int argc, char* argv[]) {
int target;
int index;
vector<int> integers = Readintegers();
cin >> target;
index = BinarySearch(target, integers, 0, integers.size() - 1);
printf("index: %d, recursions: %d, comparisons: %d\n",
index, recursions, comparisons);
return 0;
}
I am getting one error which I added as a screenshot
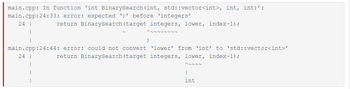
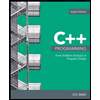
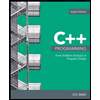