Solution Description Create a file CarWash1331.java that will simulate ordering car wash services. The Kiosk will prompt the user for a car wash service they would like to order. Your program will keep track of the car wash services the user wants, then calculate discount, tip and total. CarWash1331.java The program should work as follows. 1. Print a welcome message with menu items as illustrated below: Welcome to Car Wash 1331! Sedan/Coupe: $13.31 Truck: $21.10 SUV/Minivan: $35.10 2. Prompt the user to "Select the type of vehicle:" a. User should be able to enter a valid car type (ignoring lower/upper cases) b. If the user enters an invalid car type (ignoring lower/upper cases), terminate the program 3. Ask the user "Would you like to add Wax for $3.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the wax to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 4. Ask the user "Would you like to add Tire Polish for $5.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the tire polish to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 5. Ask the user "Would you like to add an Interior Vacuum for $7.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the interior vacuum cleaning to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 6. Ask the user, "Do you have a discount code/coupon?" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) b. If the user selects "yes": i. Prompt the user "Please enter the discount code:"
Solution Description Create a file CarWash1331.java that will simulate ordering car wash services. The Kiosk will prompt the user for a car wash service they would like to order. Your program will keep track of the car wash services the user wants, then calculate discount, tip and total. CarWash1331.java The program should work as follows. 1. Print a welcome message with menu items as illustrated below: Welcome to Car Wash 1331! Sedan/Coupe: $13.31 Truck: $21.10 SUV/Minivan: $35.10 2. Prompt the user to "Select the type of vehicle:" a. User should be able to enter a valid car type (ignoring lower/upper cases) b. If the user enters an invalid car type (ignoring lower/upper cases), terminate the program 3. Ask the user "Would you like to add Wax for $3.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the wax to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 4. Ask the user "Would you like to add Tire Polish for $5.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the tire polish to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 5. Ask the user "Would you like to add an Interior Vacuum for $7.00:" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) i. If the user selects "yes", add the interior vacuum cleaning to the subtotal ii. If the user selects "no", continue with the program b. The program will be tested against only valid inputs 6. Ask the user, "Do you have a discount code/coupon?" a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases) b. If the user selects "yes": i. Prompt the user "Please enter the discount code:"
Chapter5: Making Decisions
Section: Chapter Questions
Problem 5PE
Related questions
Question
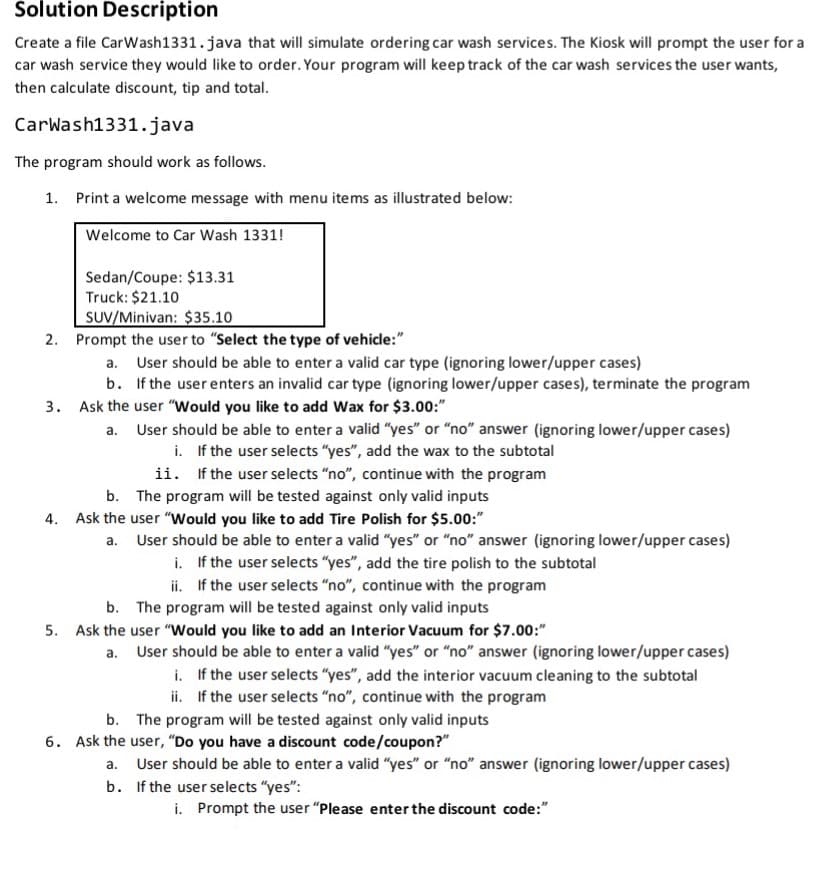
Transcribed Image Text:Solution Description
Create a file CarWash1331.java that will simulate ordering car wash services. The Kiosk will prompt the user for a
car wash service they would like to order. Your program will keep track of the car wash services the user wants,
then calculate discount, tip and total.
CarWash1331.java
The program should work as follows.
1. Print a welcome message with menu items as illustrated below:
Welcome to Car Wash 1331!
Sedan/Coupe: $13.31
Truck: $21.10
SUV/Minivan: $35.10
2. Prompt the user to "Select the type of vehicle:"
a. User should be able to enter a valid car type (ignoring lower/upper cases)
b. If the user enters an invalid car type (ignoring lower/upper cases), terminate the program
3. Ask the user "Would you like to add Wax for $3.00:"
a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases)
i. If the user selects "yes", add the wax to the subtotal
ii. If the user selects "no", continue with the program
b. The program will be tested against only valid inputs
4. Ask the user "Would you like to add Tire Polish for $5.00:"
a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases)
i. If the user selects "yes", add the tire polish to the subtotal
ii. If the user selects "no", continue with the program
b. The program will be tested against only valid inputs
5. Ask the user "Would you like to add an Interior Vacuum for $7.00:"
a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases)
i. If the user selects "yes", add the interior vacuum cleaning to the subtotal
ii. If the user selects "no", continue with the program
b. The program will be tested against only valid inputs
6. Ask the user, "Do you have a discount code/coupon?"
a. User should be able to enter a valid "yes" or "no" answer (ignoring lower/upper cases)
b. If the user selects "yes":
i. Prompt the user "Please enter the discount code:"
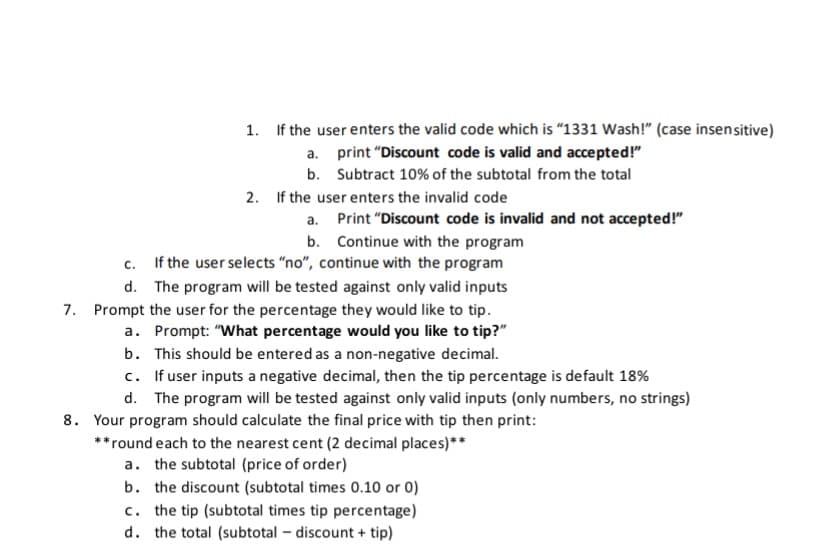
Transcribed Image Text:1. If the user enters the valid code which is "1331 Wash!" (case insensitive)
a. print "Discount code is valid and accepted!"
b. Subtract 10% of the subtotal from the total
2. If the user enters the invalid code
a. Print "Discount code is invalid and not accepted!"
b. Continue with the program
If the user selects “no", continue with the program
C.
d. The program will be tested against only valid inputs
7. Prompt the user for the percentage they would like to tip.
a. Prompt: "What percentage would you like to tip?"
b. This should be entered as a non-negative decimal.
c. If user inputs a negative decimal, then the tip percentage is default 18%
d. The program will be tested against only valid inputs (only numbers, no strings)
8. Your program should calculate the final price with tip then print:
**round each to the nearest cent (2 decimal places)**
a. the subtotal (price of order)
b. the discount (subtotal times 0.10 or 0)
c. the tip (subtotal times tip percentage)
d. the total (subtotal – discount + tip)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
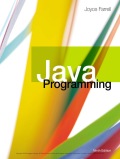
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
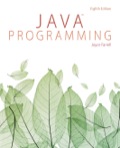
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
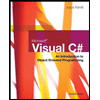
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
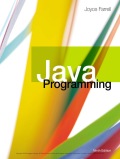
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
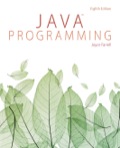
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
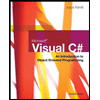
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,