solve in python Street Class Step 1: • Create a class to store information about a street: • Instance variables should include: • the street name • the length of the street (in km) • the number of cars that travel the street per day • the condition of the street (“poor”, “fair”, or “good”). • Write a constructor (__init__), that takes four arguments corresponding to the instance variables and creates one Street instance. Street Class Step 2: • Add additional methods: • __str__ • Should return a string with the Street information neatly-formatted, as in: Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition. • compare: • This method should compare one Street to another, with the Street the method is called on being compared to a second Street passed to a parameter. • Return True or False, indicating whether the first street needs repairs more urgently than the second. • Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which need repairs more urgently than streets in “good” condition. • If the condition of two Streets is the same, the one with more traffic is given higher priority. Street Class Step 3: • Add a global constant COST_PER_KM = 1400000, to represent how much it costs to repair each kilometer of road. • Examine the format of the file streetData.txt. • Add code to read streets from file, and create a list of Street objects. • (Print a list of all streets, to verify that you have read them correctly.)
solve in python Street Class Step 1: • Create a class to store information about a street: • Instance variables should include: • the street name • the length of the street (in km) • the number of cars that travel the street per day • the condition of the street (“poor”, “fair”, or “good”). • Write a constructor (__init__), that takes four arguments corresponding to the instance variables and creates one Street instance. Street Class Step 2: • Add additional methods: • __str__ • Should return a string with the Street information neatly-formatted, as in: Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition. • compare: • This method should compare one Street to another, with the Street the method is called on being compared to a second Street passed to a parameter. • Return True or False, indicating whether the first street needs repairs more urgently than the second. • Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which need repairs more urgently than streets in “good” condition. • If the condition of two Streets is the same, the one with more traffic is given higher priority. Street Class Step 3: • Add a global constant COST_PER_KM = 1400000, to represent how much it costs to repair each kilometer of road. • Examine the format of the file streetData.txt. • Add code to read streets from file, and create a list of Street objects. • (Print a list of all streets, to verify that you have read them correctly.)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
solve in python
Street Class
Step 1:
• Create a class to store information about a street:
• Instance variables should include:
• the street name
• the length of the street (in km)
• the number of cars that travel the street per day
• the condition of the street (“poor”, “fair”, or “good”).
• Write a constructor (__init__), that takes four arguments corresponding to
the instance variables and creates one Street instance.
Step 1:
• Create a class to store information about a street:
• Instance variables should include:
• the street name
• the length of the street (in km)
• the number of cars that travel the street per day
• the condition of the street (“poor”, “fair”, or “good”).
• Write a constructor (__init__), that takes four arguments corresponding to
the instance variables and creates one Street instance.
Street Class
Step 2:
• Add additional methods:
• __str__
• Should return a string with the Street information neatly-formatted, as in:
Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.
• compare:
• This method should compare one Street to another, with the Street the method is called
on being compared to a second Street passed to a parameter.
• Return True or False, indicating whether the first street needs repairs more urgently than
the second.
• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which
need repairs more urgently than streets in “good” condition.
• If the condition of two Streets is the same, the one with more traffic is given higher priority.
Step 2:
• Add additional methods:
• __str__
• Should return a string with the Street information neatly-formatted, as in:
Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.
• compare:
• This method should compare one Street to another, with the Street the method is called
on being compared to a second Street passed to a parameter.
• Return True or False, indicating whether the first street needs repairs more urgently than
the second.
• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, which
need repairs more urgently than streets in “good” condition.
• If the condition of two Streets is the same, the one with more traffic is given higher priority.
Street Class
Step 3:
• Add a global constant COST_PER_KM = 1400000, to represent how
much it costs to repair each kilometer of road.
• Examine the format of the file streetData.txt.
• Add code to read streets from file, and create a list of Street objects.
• (Print a list of all streets, to verify that you have read them correctly.)
• Print a list of the streets in poor condition, and calculate the total cost
of repairing all of them.
Step 3:
• Add a global constant COST_PER_KM = 1400000, to represent how
much it costs to repair each kilometer of road.
• Examine the format of the file streetData.txt.
• Add code to read streets from file, and create a list of Street objects.
• (Print a list of all streets, to verify that you have read them correctly.)
• Print a list of the streets in poor condition, and calculate the total cost
of repairing all of them.
Step 4: Additional Practice
• Modify the code that constructs the Street list, so that the Streets are
ordered by priority in the list.
• Do not use a sort() function. Write your own sort, or preferably, perform an
ordered insert as you are reading streets from the txt file.
• (Make use of your compare method)
• If you have $40 million to spend on repairs, which streets could be
repaired?
• Modify the code that constructs the Street list, so that the Streets are
ordered by priority in the list.
• Do not use a sort() function. Write your own sort, or preferably, perform an
ordered insert as you are reading streets from the txt file.
• (Make use of your compare method)
• If you have $40 million to spend on repairs, which streets could be
repaired?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
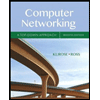
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
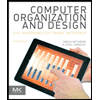
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
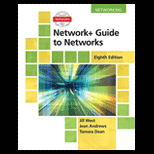
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
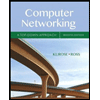
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
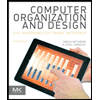
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
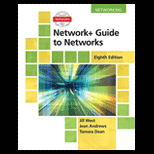
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
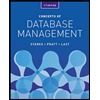
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
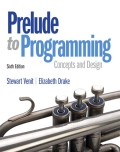
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
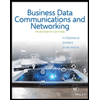
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY