Sometimes servers are down, so clients cannot connect to them. Python raises an exception of type ConnectionRefusedError in a client program when a network connection is refused. You can generate this error by running the timeclient.py file. Add exception handling code to the server logic in timeclient.py to catch and recover from this kind of exception. To recover from this exception, simply print the message Error connecting to the server and terminate the program. This lab follows a client server model. In order for the client program to connect to the server the following steps must be taken: Enter python3 timeserver.py into the first Terminal. Open a new terminal tab by clicking the '+' at the top of the terminal pane. Enter python3 timeclient.py into the second Terminal. The client code will now be able to establish a connection to the server. In order to test your error handling logic, run python3 timeclient.py into the terminal without starting the timesever. If you have already started the timeserver, use CTL+C to terminate the process or close the terminal window which is running the timeserver. """ File: timeclient.py Project 10.2 Client for obtaining the day and time. Recovers from connection errors. """ from socket import * from codecs import decode HOST = "localhost" PORT = 5000 BUFSIZE = 1024 ADDRESS = (HOST, PORT) server = socket(AF_INET, SOCK_STREAM) # Create a socket server.connect(ADDRESS) # Connect it to a host dayAndTime = decode(server.recv(BUFSIZE), "ascii") # Read a string from it print(dayAndTime) server.close()
Sometimes servers are down, so clients cannot connect to them. Python raises an exception of type ConnectionRefusedError in a client program when a network connection is refused.
You can generate this error by running the timeclient.py file.
Add exception handling code to the server logic in timeclient.py to catch and recover from this kind of exception. To recover from this exception, simply print the message Error connecting to the server and terminate the program.
This lab follows a client server model. In order for the client program to connect to the server the following steps must be taken:
- Enter python3 timeserver.py into the first Terminal.
- Open a new terminal tab by clicking the '+' at the top of the terminal pane.
- Enter python3 timeclient.py into the second Terminal.
The client code will now be able to establish a connection to the server.
In order to test your error handling logic, run python3 timeclient.py into the terminal without starting the timesever. If you have already started the timeserver, use CTL+C to terminate the process or close the terminal window which is running the timeserver.

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 5 images

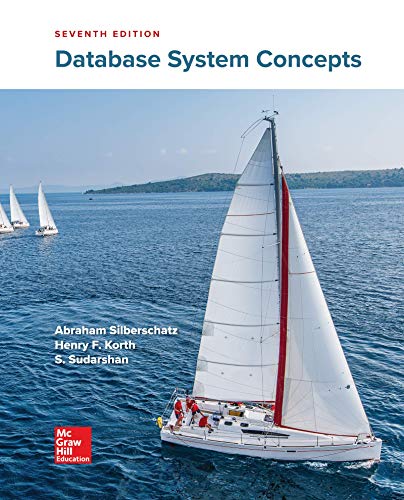
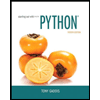
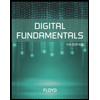
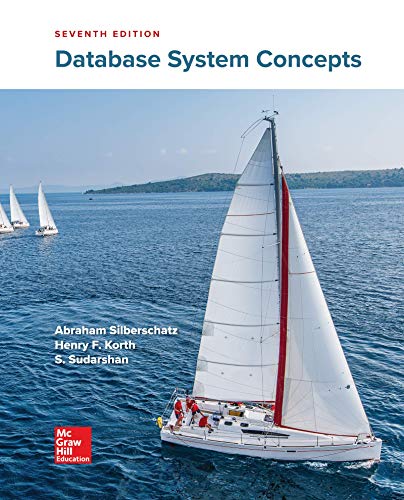
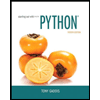
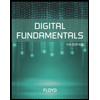
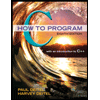
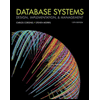
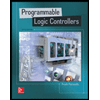