Stack,
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++ Programming
Topic: Stack, Ques and Deques
Below is the initial program of the main file, only modify the main file, sllstack file also provided. See attached photo for instructions.
main.cpp
#include <iostream>
#include <cstring>
#include "sllstack.h"
using namespace std;
int main(int argc, char** argv) {
SLLStack* stack = new SLLStack();
int test;
string str;
cin >> test;
switch (test) {
case 0:
getline(cin, str);
// PERFORM SOLUTION TO BRACKETS PROBLEM HERE
// FYI: Place your variable declarations, if any, before switch.
break;
case 1:
stack->push('a');
stack->push('b');
stack->push('c');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
case 2:
stack->push('5');
stack->push('3');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('7');
stack->push('9');
stack->push('4');
cout << stack->pop() << endl;
stack->push('6');
stack->push('8');
cout << stack->pop() << endl;
cout << stack->size() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
case 3:
stack->push('a');
stack->push('b');
stack->push('c');
cout << stack->pop() << endl;
stack->push('d');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('e');
stack->push('f');
cout << stack->size() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
case 4:
stack->push('C');
cout << stack->pop() << endl;
stack->push('L');
stack->push('K');
stack->push('N');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('O');
stack->push('V');
stack->push('W');
cout << stack->pop() << endl;
stack->push('E');
cout << stack->size() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
case 5:
stack->push('B');
cout << stack->pop() << endl;
stack->push('K');
stack->push('H');
stack->push('V');
stack->push('O');
stack->push('C');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('P');
stack->push('E');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->size() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
case 6:
stack->push('g');
stack->push('h');
stack->push('i');
stack->push('j');
stack->push('k');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('l');
cout << stack->pop() << endl;
cout << stack->pop() << endl;
stack->push('m');
stack->push('n');
cout << stack->size() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->pop() << endl;
cout << stack->isEmpty() << endl;
break;
}
return 0;
}
sllstack.h
#include "stack.h"
#include "linkedlist.h"
// SLLStack means Singly Linked List (SLL) Stack
class SLLStack : public Stack {
LinkedList* list;
public:
SLLStack() {
list = new LinkedList();
}
void push(char e) {
list->add(e);
return;
}
char pop() {
char elem;
elem = list->removeTail();
return elem;
}
char top() {
char elem;
elem = list->get(size());
return elem;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
};
![Additionally, you are also going to solve the Brackets Problem in
the case O of the main.cpp file where you have to identify
whether a given string that contains brackets is valid or not.
Brackets may be parentheses ( and ), curly brackets { and },
and square brackets [ and ]. Each opening symbol must match
its corresponding closing symbol. For example, a left bracket,
"L" must match a corresponding right bracket, ")," as in the
following expression: [0]
Take note of the three considerations that will make the string
invalid: a mismatch where the closing bracket did not match
what was in the top of the stack (e.g. "(1)"), an excess opening
where we have completed checking the string where there
should have been more closing bracket/s (e.g. "(0"), and an
excess closing where we found a closing bracket but there's no
opening bracket to match it with (e.g. "0)").
Using the methods of a stack and your SLL implementation,
create a separate program that will print accepted if a given
string is valid exclusively in terms of brace pairing and
otherwise, print not accepted.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2cb11186-80ff-4474-bc75-6eff69002743%2F6ef32611-b6cd-459c-9679-fa927c045875%2F23572lk_processed.png&w=3840&q=75)
Transcribed Image Text:Additionally, you are also going to solve the Brackets Problem in
the case O of the main.cpp file where you have to identify
whether a given string that contains brackets is valid or not.
Brackets may be parentheses ( and ), curly brackets { and },
and square brackets [ and ]. Each opening symbol must match
its corresponding closing symbol. For example, a left bracket,
"L" must match a corresponding right bracket, ")," as in the
following expression: [0]
Take note of the three considerations that will make the string
invalid: a mismatch where the closing bracket did not match
what was in the top of the stack (e.g. "(1)"), an excess opening
where we have completed checking the string where there
should have been more closing bracket/s (e.g. "(0"), and an
excess closing where we found a closing bracket but there's no
opening bracket to match it with (e.g. "0)").
Using the methods of a stack and your SLL implementation,
create a separate program that will print accepted if a given
string is valid exclusively in terms of brace pairing and
otherwise, print not accepted.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
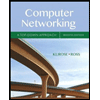
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
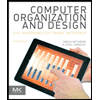
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
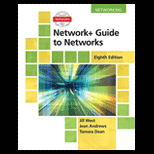
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
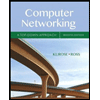
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
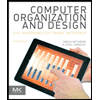
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
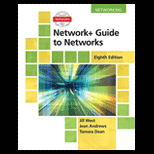
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
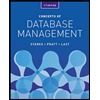
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
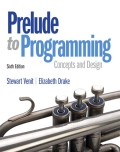
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
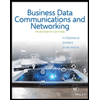
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY