Step 1: Add code to prompt the user to enter the name of the room that they are entering information for. Validate the input of the name of the room so that an error is shown if the user does not enter a name for the room. The user must be given an unlimited amount of attempts to enter a name for the room. Step 2: Add Input Validation to the code using the following rules: The length of the room cannot be less than 5 feet The width of the room cannot be less than 5 feet The user's menu selection for the amount of shade should be 1, 2, or 3. If the user's input is invalid, the program should display an appropriate error message and prompt the user to re-enter their input. The user must be given an unlimited amount of attempts to enter valid input. Step 3: Add code to have the program ask the user if they would like to enter information about another room after the information for a previous room has been processed and displayed. The user should be able to enter a "y" or "Y" to indicate that they want to enter information about another room. See the Sample Input and Output for how to format this. Step 4: After the user has indicated that they do not wish to enter information about another room, the program should output the number of rooms that have been entered and then stop executing. this is my project 1 #include #include using namespace std; int main() { // declare variables double length, width, area, capacity; int menuChoice; string shade; string title = "Air Conditioning Window Unit Cooling Capacity"; // input of the length of the room cout << "Please enter the length of the room (in feet): "; cin >> length; // validate the input of the length if(length < 0) { cout << "Error: invalid length entered. Program will terminate."; return 0; } // input of the width of the room cout << "Please enter the width of the room (in feet): "; cin >> width; // validate the input of the width if(width < 0) { cout << "Error: invalid width entered. Program will terminate."; return 0; } // calculate the area of the room area = length * width; // display a menu that asks the user how much shade the room gets cout << "What is the amount of shade that this room recieves? \n"; cout << "\n\t 1. Little Shade"; cout << "\n\t 2. Moderate Shade"; cout << "\n\t 3. Abundant Shade"; cout << "\n\n"; cout << "Please select from the options above: "; cin >> menuChoice; // validate the menu choice if(menuChoice < 1 or menuChoice > 3) { cout << "Error: Invalid menu choice entered. Program will terminate."; return 0; } // determine the capacity if(area < 250) capacity = 5500; else if(area >= 250 and area <= 500) capacity = 10000; else if(area > 500 and area <= 1000) capacity = 17500; else capacity = 24000; // apply the shade if(menuChoice == 1) { capacity = capacity + (capacity * (0.15)); // little shade shade = "Little"; } else if(menuChoice == 2) { // moderate shade shade = "Moderate"; } else { // abundant shade capacity = capacity - (capacity * (0.10)); shade = "Abundant"; } // output cout << setprecision(2) << showpoint << fixed; cout << endl << title << endl << endl; cout << "Room Area (in square feet):" << area << endl; cout << "Amount of Shade: " << shade << endl; cout << "BTU's Per Hour needed: " << capacity << endl; return; }
I need help to my project 2 programming C++. Please
Project 2 is a continuation of Project 1. Modify the code from Project 1 in the following ways:
Step 1: Add code to prompt the user to enter the name of the room that they are entering information for. Validate the input of the name of the room so that an error is shown if the user does not enter a name for the room. The user must be given an unlimited amount of attempts to enter a name for the room.
Step 2: Add Input Validation to the code using the following rules:
- The length of the room cannot be less than 5 feet
- The width of the room cannot be less than 5 feet
- The user's menu selection for the amount of shade should be 1, 2, or 3.
If the user's input is invalid, the program should display an appropriate error message and prompt the user to re-enter their input. The user must be given an unlimited amount of attempts to enter valid input.
Step 3: Add code to have the program ask the user if they would like to enter information about another room after the information for a previous room has been processed and displayed. The user should be able to enter a "y" or "Y" to indicate that they want to enter information about another room. See the Sample Input and Output for how to format this.
Step 4: After the user has indicated that they do not wish to enter information about another room, the program should output the number of rooms that have been entered and then stop executing.
this is my project 1
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
// declare variables
double length, width, area, capacity;
int menuChoice;
string shade;
string title = "Air Conditioning
Window Unit Cooling Capacity";
// input of the length of the room
cout << "Please enter the length of the room (in feet): ";
cin >> length;
// validate the input of the length if(length < 0)
{
cout << "Error: invalid length entered. Program will terminate.";
return 0;
}
// input of the width of the room
cout << "Please enter the width of the room (in feet): ";
cin >> width;
// validate the input of the width if(width < 0)
{
cout << "Error: invalid width entered. Program will terminate.";
return 0;
}
// calculate the area of the room area = length * width;
// display a menu that asks the user how much shade the room gets
cout << "What is the amount of shade that this room recieves? \n";
cout << "\n\t 1. Little Shade";
cout << "\n\t 2. Moderate Shade";
cout << "\n\t 3. Abundant Shade";
cout << "\n\n";
cout << "Please select from the options above: ";
cin >> menuChoice; // validate the menu choice if(menuChoice < 1 or menuChoice > 3)
{
cout << "Error: Invalid menu choice entered. Program will terminate.";
return 0;
}
// determine the capacity
if(area < 250)
capacity = 5500;
else if(area >= 250 and area <= 500)
capacity = 10000;
else if(area > 500 and area <= 1000) capacity = 17500;
else
capacity = 24000;
// apply the shade
if(menuChoice == 1)
{
capacity = capacity +
(capacity * (0.15)); // little shade
shade = "Little";
}
else if(menuChoice == 2)
{
// moderate shade
shade = "Moderate";
}
else
{
// abundant shade
capacity = capacity -
(capacity * (0.10)); shade = "Abundant";
}
// output
cout << setprecision(2) << showpoint << fixed;
cout << endl << title << endl << endl;
cout << "Room Area (in square feet):"
<< area << endl;
cout << "Amount of Shade: " << shade << endl; cout << "BTU's Per Hour needed: " << capacity << endl;
return;
}
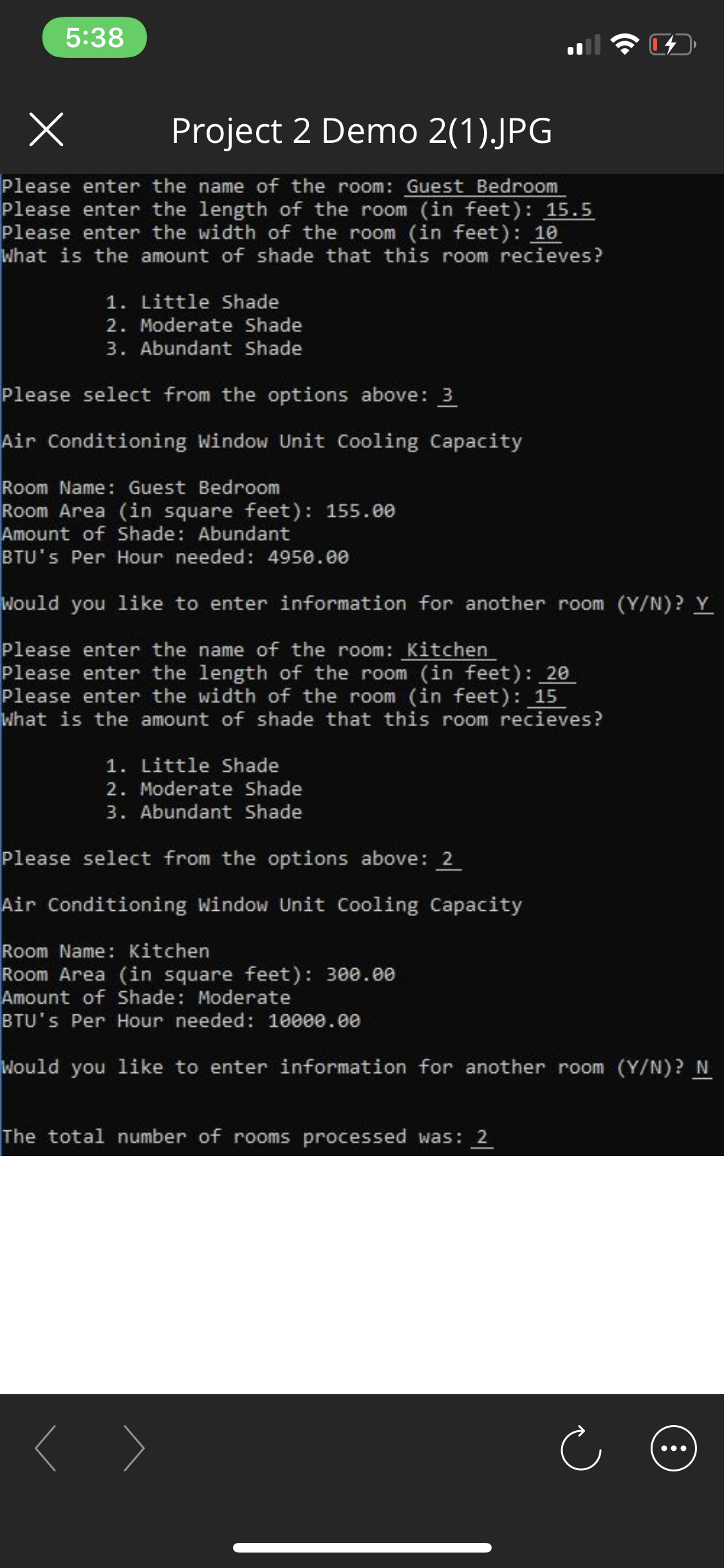
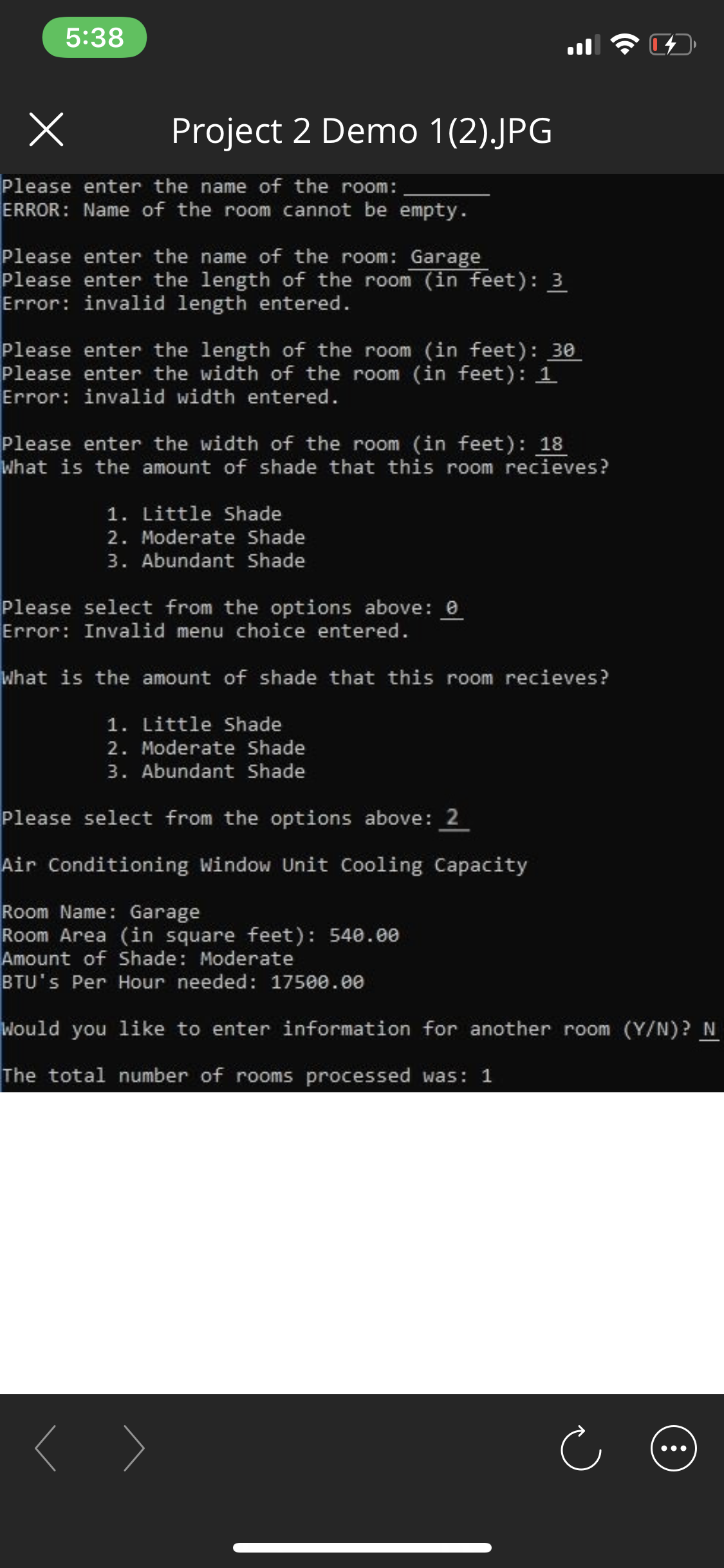

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

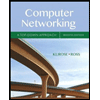
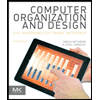
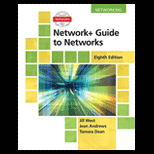
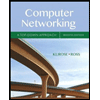
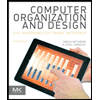
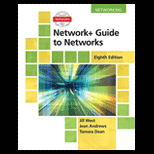
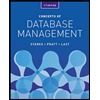
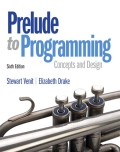
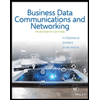