Step 3: Complete the public boolean checkWin(int[][] board) method. In order to check if a puzzle is solved or not, we need to check if the current puzzle satisfy the row rule, column rule, and grid rule. So that is to check if every row of the puzzle contains the number 1 to 9 exactly once, every column contains the number 1 to 9 exactly once, and every 3X3 subgrid contains the number 1 to 9 exactly once. The nested loops for checking rows are given to you for your reference. Read the comments to understand it. Then complete the code to check the columns and the subgrids This is step three. On this practice problem we were given an incomplete java file that is being used to solve a suduko board. Ill try to copy and paste the code I have so far down below but I dont know how well that will work. import java.util.Arrays; import java.util.Random; public class Sudoku { public static final int[][] BOARD = { {4, 3, 5, 8, 7, 6, 1, 2, 9}, {8, 7, 6, 2, 1, 9, 3, 4, 5}, {2, 1, 9, 4, 3, 5, 7, 8, 6}, {5, 2, 3, 6, 4, 7, 8, 9, 1}, {9, 8, 1, 5, 2, 3, 4, 6, 7}, {6, 4, 7, 9, 8, 1, 2, 5, 3}, {7, 5, 4, 1, 6, 8, 9, 3, 2}, {3, 9, 2, 7, 5, 4, 6, 1, 8}, {1, 6, 8, 3, 9, 2, 5, 7, 4}}; public static final int GRID_SIZE = 9; public static final int SUBGRID_SIZE = 3; public static final double EMPTYCELL_PERCENTAGE = 0.5; public static final String VALUE = "123456789"; private int[][] puzzle; private Random random = new Random(); // Create a copy of the sudoku board private int[][] copyOfBoard(int[][] originalBoard) { int n = original.length; int m = original[0].length; int[][] copy = new int[n][m]; for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { copy[i][j] = original[i][j]; } } return copy; } // provide two random number as an array with length two. // used to hide some numbers private int[] getTwoRanNum(int min, int range) { Random rd = new Random(); int x = (int) (min + Math.random() * range); int y = (int) (min + Math.random() * range); int[] arr = new int[2]; arr[0] = x; arr[1] = y; return arr; } // Hide some numbers to create puzzle. public int[][] createNewPuzzle(int[][] board) { this.puzzle = copyOfBoard(board); int numOfEmptyCell = (int) Math.floor(EMPTYCELL_PERCENTAGE * GRID_SIZE * GRID_SIZE); for (int i = 0; i < numOfEmptyCell; i++) { int[] rowcol = getTwoRanNum(0, board.length); this.puzzle[rowcol[0]][rowcol[1]] = 0; } return copyOfBoard(this.puzzle); } // Check if puzzle is solved or not public boolean checkWin(int[][] board) { boolean isWin = true; // check rows for (int i = 0; i < board.length; i++) { // copy the String object VALUE to rowValues to check the row String rowValues = VALUE; for (int j = 0; j < board.length; j++) { //for any cell, delete its value from the string rowValues rowValues = rowValues.replace("" + board[i][j], ""); } // after one row is checked, the rowValues should be an empty string // if rowValus is not empty, then the row must contain some repeated numbers // thus we set isWin to false and return it if (!rowValues.isEmpty()) { isWin = false; return isWin; } } // step 3 // check columns // step 3 //check sub grids return isWin; } // check if the puzzle is completed or not public boolean isComplete(int [][] puzzle) { // step 4 // check if the current puzzle is completed or not } public void printArray(int[][] a) { System.out.println(" 0 1 2 3 4 5 6 7 8"); for (int i = 0; i < a.length; i++) { System.out.println(" -------------------------------------"); System.out.print("row " + i + ": "); for (int j = 0; j < a[i].length; j++) { if(a[i][j] != 0) System.out.print("| " + a[i][j] + " "); else System.out.print("| "); } System.out.println("|"); } System.out.println(" -------------------------------------"); } } }
Step 3: Complete the public boolean checkWin(int[][] board) method. In order to check if a puzzle
is solved or not, we need to check if the current puzzle satisfy the row rule, column rule,
and grid rule. So that is to check if every row of the puzzle contains the number 1 to 9
exactly once, every column contains the number 1 to 9 exactly once, and every 3X3 subgrid
contains the number 1 to 9 exactly once. The nested loops for checking rows are given to
you for your reference. Read the comments to understand it. Then complete the code to
check the columns and the subgrids
This is step three. On this practice problem we were given an incomplete java file that is being used to solve a suduko board.
Ill try to copy and paste the code I have so far down below but I dont know how well that will work.
import java.util.Arrays;
import java.util.Random;
public class Sudoku {
public static final int[][] BOARD = {
{4, 3, 5, 8, 7, 6, 1, 2, 9},
{8, 7, 6, 2, 1, 9, 3, 4, 5},
{2, 1, 9, 4, 3, 5, 7, 8, 6},
{5, 2, 3, 6, 4, 7, 8, 9, 1},
{9, 8, 1, 5, 2, 3, 4, 6, 7},
{6, 4, 7, 9, 8, 1, 2, 5, 3},
{7, 5, 4, 1, 6, 8, 9, 3, 2},
{3, 9, 2, 7, 5, 4, 6, 1, 8},
{1, 6, 8, 3, 9, 2, 5, 7, 4}};
public static final int GRID_SIZE = 9;
public static final int SUBGRID_SIZE = 3;
public static final double EMPTYCELL_PERCENTAGE = 0.5;
public static final String VALUE = "123456789";
private int[][] puzzle;
private Random random = new Random();
// Create a copy of the sudoku board
private int[][] copyOfBoard(int[][] originalBoard) {
int n = original.length;
int m = original[0].length;
int[][] copy = new int[n][m];
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
copy[i][j] = original[i][j];
}
}
return copy;
}
// provide two random number as an array with length two.
// used to hide some numbers
private int[] getTwoRanNum(int min, int range) {
Random rd = new Random();
int x = (int) (min + Math.random() * range);
int y = (int) (min + Math.random() * range);
int[] arr = new int[2];
arr[0] = x;
arr[1] = y;
return arr;
}
// Hide some numbers to create puzzle.
public int[][] createNewPuzzle(int[][] board) {
this.puzzle = copyOfBoard(board);
int numOfEmptyCell = (int) Math.floor(EMPTYCELL_PERCENTAGE * GRID_SIZE * GRID_SIZE);
for (int i = 0; i < numOfEmptyCell; i++) {
int[] rowcol = getTwoRanNum(0, board.length);
this.puzzle[rowcol[0]][rowcol[1]] = 0;
}
return copyOfBoard(this.puzzle);
}
// Check if puzzle is solved or not
public boolean checkWin(int[][] board) {
boolean isWin = true;
// check rows
for (int i = 0; i < board.length; i++) {
// copy the String object VALUE to rowValues to check the row
String rowValues = VALUE;
for (int j = 0; j < board.length; j++) {
//for any cell, delete its value from the string rowValues
rowValues = rowValues.replace("" + board[i][j], "");
}
// after one row is checked, the rowValues should be an empty string
// if rowValus is not empty, then the row must contain some repeated numbers
// thus we set isWin to false and return it
if (!rowValues.isEmpty()) {
isWin = false;
return isWin;
}
}
// step 3
// check columns
// step 3
//check sub grids
return isWin;
}
// check if the puzzle is completed or not
public boolean isComplete(int [][] puzzle) {
// step 4
// check if the current puzzle is completed or not
}
public void printArray(int[][] a) {
System.out.println(" 0 1 2 3 4 5 6 7 8");
for (int i = 0; i < a.length; i++) {
System.out.println(" -------------------------------------");
System.out.print("row " + i + ": ");
for (int j = 0; j < a[i].length; j++) {
if(a[i][j] != 0)
System.out.print("| " + a[i][j] + " ");
else
System.out.print("| ");
}
System.out.println("|");
}
System.out.println(" -------------------------------------");
}
}
}



Step by step
Solved in 5 steps with 2 images

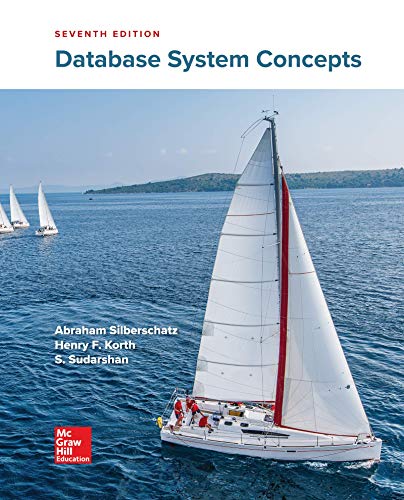
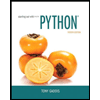
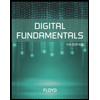
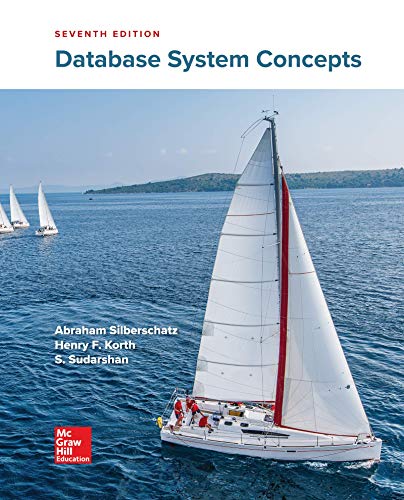
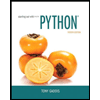
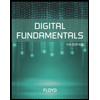
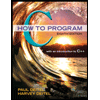
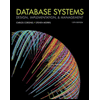
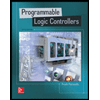