matches.txt file data bellow. ``` Charlie Bradbury F 42 65 N Green 5558675309 Bobby Singer M 70 69 Y Brown 5558675309 Dean Winchester M 43 72 N Brown 5558675309 Sam Winchester M 39 75 N Brown 5558675309 Jody Mills F 51 65 N Brown 5558675309 Bela Talbot F 39 69 Y Blue 5558675309 James Novak M 46 71 Y Blue 5558675309 ``` code. #include #include #include #include using namespace std; int main() { char user_gender, user_smoker; string user_eyecolor; int user_minAge, user_maxAge, user_minHeight, user_maxHeight; cout << "What is the gender of your ideal match(M, F, N) ? "; cin >> user_gender; cout << "What is the minimum age? "; cin >> user_minAge; cout << "What is the maximum age? "; cin >> user_maxAge; cout << "What is the minimum height (in inches)? "; cin >> user_minHeight; cout << "What is the maximum height (in inches)? "; cin >> user_maxHeight; cout << "Smoker (Y/N)? "; cin >> user_smoker; cout << "What is the eyecolor (Blue, Green, Grey, Brown)? "; cin >> user_eyecolor; cout << endl << endl; //Variables to check against the conditions int countGender = 0; int partialMatch = 0; int fullMatch = 0; cout << endl << left << setw(1) << " Name" << fixed << right << setw(22) << "Age" << fixed << right << setw(12) << "Height" << fixed << right << setw(12) << "Smoker" << fixed << right << setw(15) << "Eye Color" << fixed << right << setw(22) << "Phone" << endl; cout << "-----------------------------------------------------------------------------------------------------------------" << endl; //Now read the file data. ifstream fin("matches.txt"); if (fin.is_open()) { while (!fin.eof()) { string firstname, lastname, eyecolor, phoneno; char gender, smoker; int age, height; fin >> firstname >> lastname >> gender >> age >> height >> smoker >> eyecolor >> phoneno; if (gender == user_gender) { countGender++; //Now check to see if the age and height are between the maximum and minum preferences. if ((age >= user_minAge && age <= user_maxAge) && (height >= user_minHeight && height <= user_maxHeight)) { //Then check to see if the smoking preference and eye color are also a match. if (user_smoker == smoker && user_eyecolor == eyecolor) { fullMatch++; cout << "* " << firstname << " " << lastname << setw(25) << age << setw(11) << height << setw(11) << smoker << setw(11) << eyecolor << setw(11) << phoneno << endl; } else if (eyecolor == user_eyecolor) { partialMatch++; cout << " " << firstname << " " << lastname << setw(24) << age << setw(11) << height << setw(11) << smoker << setw(11) << eyecolor<< setw(11) << phoneno << endl; } } } } cout << "-----------------------------------------------------------------------------" << endl; cout << "There were " << fullMatch << " matches and " << partialMatch << " partial matches out of " << countGender << " records." << endl; cout << "-----------------------------------------------------------------------------" << endl; fin.close(); } else { cout << "File did not open"; } return 0; }
matches.txt file data bellow.
```
Charlie Bradbury F 42 65 N Green 5558675309
Bobby Singer M 70 69 Y Brown 5558675309
Dean Winchester M 43 72 N Brown 5558675309
Sam Winchester M 39 75 N Brown 5558675309
Jody Mills F 51 65 N Brown 5558675309
Bela Talbot F 39 69 Y Blue 5558675309
James Novak M 46 71 Y Blue 5558675309
```
code.
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
{
char user_gender, user_smoker;
string user_eyecolor;
int user_minAge, user_maxAge, user_minHeight, user_maxHeight;
cout << "What is the gender of your ideal match(M, F, N) ? ";
cin >> user_gender;
cout << "What is the minimum age? ";
cin >> user_minAge;
cout << "What is the maximum age? ";
cin >> user_maxAge;
cout << "What is the minimum height (in inches)? ";
cin >> user_minHeight;
cout << "What is the maximum height (in inches)? ";
cin >> user_maxHeight;
cout << "Smoker (Y/N)? ";
cin >> user_smoker;
cout << "What is the eyecolor (Blue, Green, Grey, Brown)? ";
cin >> user_eyecolor;
cout << endl << endl;
//Variables to check against the conditions
int countGender = 0;
int partialMatch = 0;
int fullMatch = 0;
cout << endl << left << setw(1) << " Name" << fixed << right << setw(22) << "Age" << fixed << right << setw(12) << "Height" << fixed << right << setw(12) << "Smoker" << fixed << right << setw(15) << "Eye Color" << fixed << right << setw(22) << "Phone" << endl;
cout << "-----------------------------------------------------------------------------------------------------------------" << endl;
//Now read the file data.
ifstream fin("matches.txt");
if (fin.is_open())
{
while (!fin.eof())
{
string firstname, lastname, eyecolor, phoneno;
char gender, smoker;
int age, height;
fin >> firstname >> lastname >> gender >> age >> height >> smoker >> eyecolor >> phoneno;
if (gender == user_gender)
{
countGender++;
//Now check to see if the age and height are between the maximum and minum preferences.
if ((age >= user_minAge && age <= user_maxAge) && (height >= user_minHeight && height <= user_maxHeight))
{
//Then check to see if the smoking preference and eye color are also a match.
if (user_smoker == smoker && user_eyecolor == eyecolor)
{
fullMatch++;
cout << "* " << firstname << " " << lastname << setw(25) << age << setw(11) << height << setw(11) << smoker << setw(11) << eyecolor << setw(11) << phoneno << endl;
}
else if (eyecolor == user_eyecolor)
{
partialMatch++;
cout << " " << firstname << " " << lastname << setw(24) << age << setw(11) << height << setw(11) << smoker << setw(11) << eyecolor<< setw(11) << phoneno << endl;
}
}
}
}
cout << "-----------------------------------------------------------------------------" << endl;
cout << "There were " << fullMatch << " matches and " << partialMatch << " partial matches out of " << countGender << " records." << endl;
cout << "-----------------------------------------------------------------------------" << endl;
fin.close();
}
else {
cout << "File did not open";
}
return 0;
}
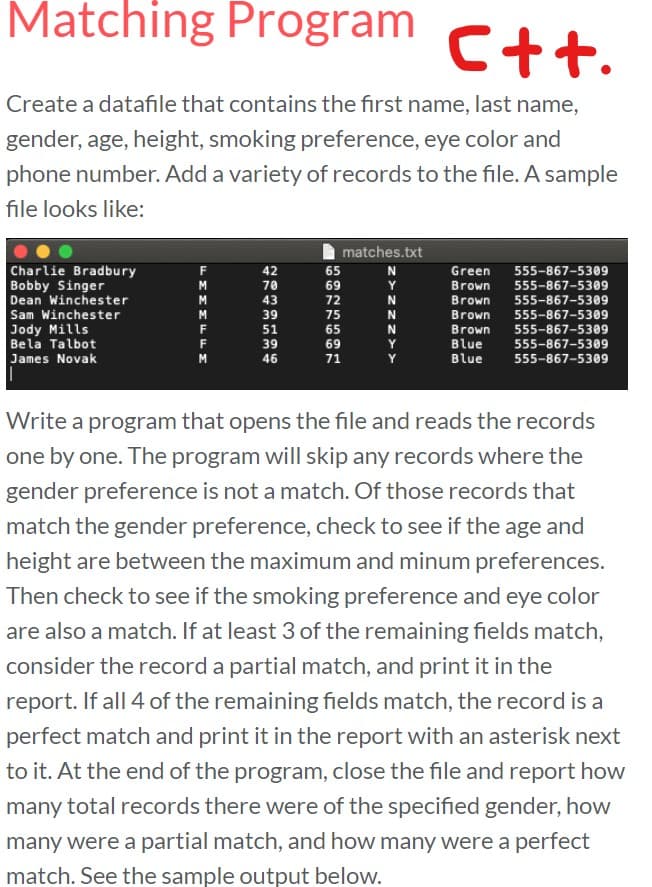
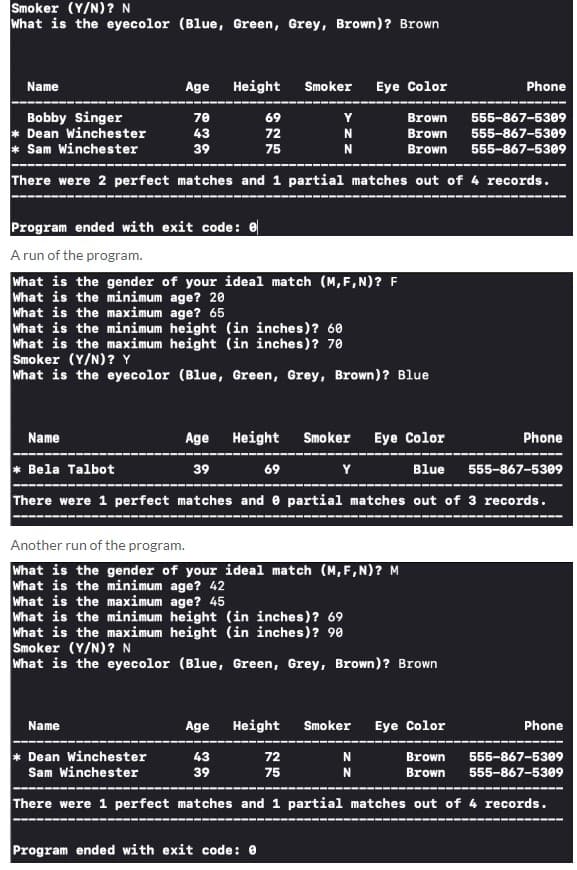

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Still not working for other user inputs as shown in the sreenshot.
Kindly, would you please check for all the three sample output as well? Thank you.
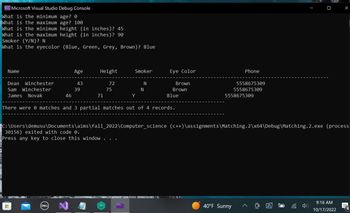
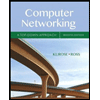
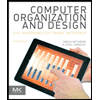
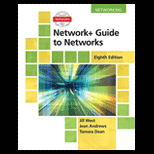
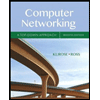
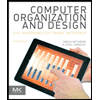
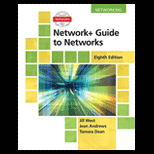
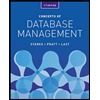
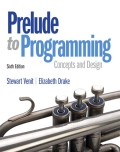
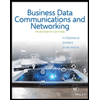