Students in this college pay $236.45 per credit hour in addition to a $52 administrative fee. Your code should generate a tuition invoice (a method within the class Student). Note that students get a 25% off total payment if their gpa is greater or equal to 3.85. Both classes Faculty and Student inherit from the abstract class Person. The abstract class Person has what's common to both Faculty and Student, and it is left to you (the programmer) to come out with an abstract method that you implement in your classes Faculty and Student, say void printinfo(); You may change the class Person to an interface to be implemented by Faculty and Student. Either way is fine! Your code should handle any Input Mismatch Exceptions. In addition, you code must have a user-defined exception called IdException that handles the faculty and student ids entered by the user. Note that any id has to be of the form LetterLetterDigitDigitDigitDigit like el7894. See sample run below. Test your code with one array of size 100 (or ArrayList) of type Person. The sample run below should give you a clear idea about how your code should run. The user's entry is marked in bold so you can tell what your code should display to the screen and what the user enters. Please note well that: 1) Your code should run exactly as shown on the sample run below (However, the TA will not deduct points because you skipped two lines instead of three or your tuition invoice has 56 hyphens instead of 63! ). 2) When asked to enter the enter the faulty department, matheMatics and MathematiCs are considered to be the same, and your program should display mathematics if faculty information is to be displayed to the screen. However, if the user enters Mathematics department, then this is an invalid entry. 3) The university ID has no required form so you may choose to enter anything to be the ID. This project is similar to the previous one. It involves implementing a simple university personnel management program. The program contains two different kinds of objects: students and faculty. For each object, the program stores relevant information such as university ID, name, etc. Different information is stored depending on the type of the object. For example, a student has a GPA, while a faculty has a title and department (professor, mathematics). For each of any class data member, your program must include the getters and the setters, and each class must include at least two constructors. The goal of this Project is to demonstrate the use of inheritance, abstract classes or interfaces, abstract methods and method overriding.
This is how the program should look like :
Welcome to my Personal Management Program
Choose one of the options:
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 2
Enter the student’s info:
Name of Student: Julia Alvarez
ID: ju1254
Gpa: 3.26
Credit hours: 7
Thanks!
Choose one of the options:
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 2
Enter the student’s info:
Name of Student: Matt Jones
ID: ma0258
Gpa: 2.78
Credit hours: 0
Thanks!
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: a
Invalid entry- please try again
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 1
Enter the faculty’s info:
Name of the faculty: John Miller
ID: jo7894
Rank: Instructor
Sorry entered rank (Instructor) is invalid
Rank: Professor
Department: Engineering
Thanks!
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 3
Enter the student’s id: ju1254
Here is the tuition invoice for Julia Alvarez :
---------------------------------------------------------------------------
Julia Alvarez ju1254
Credit Hours:7 ($236.45/credit hour)
Fees: $52
Total payment: $1,707.15 ($0 discount applied)
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 3
Enter the student’s id: eri856
Sorry-student not found!
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 4
Enter the faculty’s id: jo8578
Sorry jo8578 doesn’t exist
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 4
Enter the faculty’s id: JO7894
Faculty found:
---------------------------------------------------------------------------
John Miller
Engineering Department, Professor
---------------------------------------------------------------------------
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 1
Enter the faculty’s info:
Name of the faculty: Erika J. Jones
ID: e7894
Sorry Invalid id format-It has to be LetterLetterDigitDigitDigitDigit
- Add a new Faculty member
- Add a new Student
- Print tuition invoice for a student
- Print information of a faculty
- Exit Program
Enter your selection: 5
Goodbye!
This is one part of my code :
class Faculty extends Person{
public String Department;
public String Rank;
public void add() {
// TODO Auto-generated method stub
}
public String getDepartment() {
return Department;
}
public void setDepartment(String department) {
Department = department;
}
public String getRank() {
return Rank;
}
public void setRank(String rank) {
Rank = rank;
}
}
class Student extends Person {
public double Gpa;
public int numberOfCreditHoursCurrentlyTaken;
public double getGpa() {
return Gpa;
}
public void setGpa(double gpa) {
Gpa = gpa;
}
public int getNumberOfCreditHoursCurrentlyTaken() {
return numberOfCreditHoursCurrentlyTaken;
}
public void setNumberOfCreditHoursCurrentlyTaken(int numberOfCreditHoursCurrentlyTaken) {
this.numberOfCreditHoursCurrentlyTaken = numberOfCreditHoursCurrentlyTaken;
}
public void add() {}
public void generateTuituonInvoice(Student stnt)
{
System.out.println("Here is the tuition invoice for" +stnt.fullName+":");
System.out.println("--------------------------------------------------");
System.out.println(stnt.fullName+" "+ stnt.ID);
System.out.println("Credit Hours:"+stnt.numberOfCreditHoursCurrentlyTaken+"($236.45/credit hour)");
System.out.println("Fees: $52");
if(Gpa>=3.85)
{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.75;
double dis= (52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45))*0.25;
System.out.println("Total payment: $" +payment+"($"+dis+" discount applied)");
}
else{
double payment=(52+(stnt.numberOfCreditHoursCurrentlyTaken*236.45));
System.out.println("Total payment: $" +payment+"($0 discount applied)");
}
System.out.println("--------------------------------------------------");
}
}
abstract class Person {
public String fullName;
public String ID;
public abstract void add();
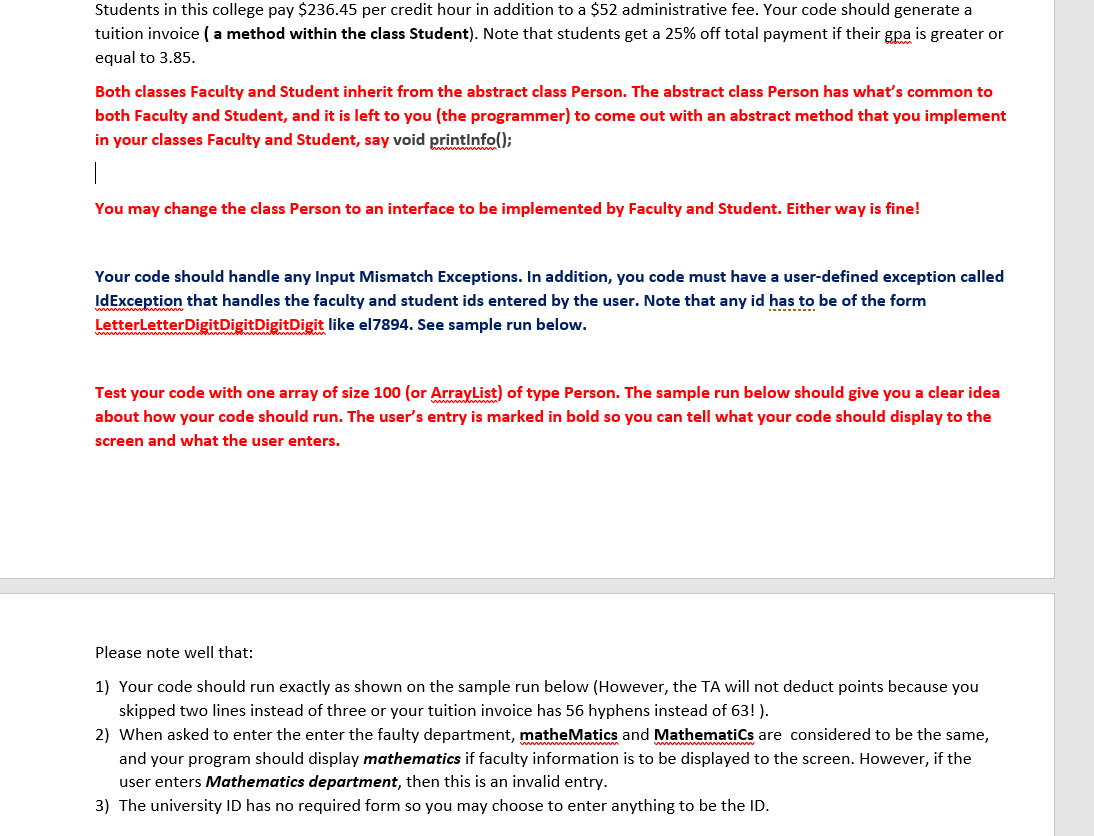
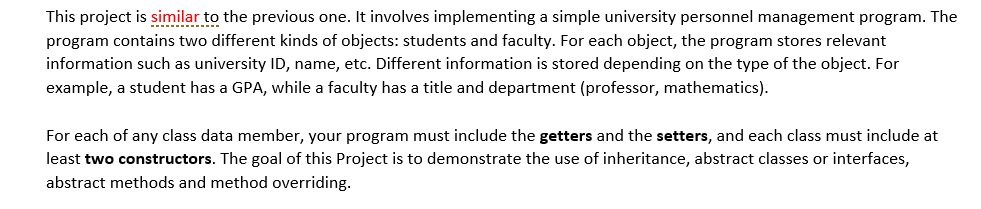

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 3 images

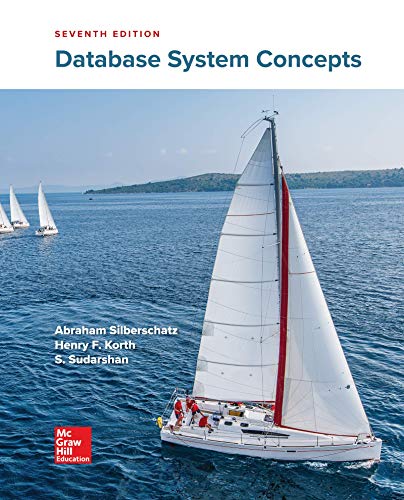
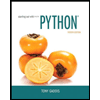
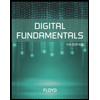
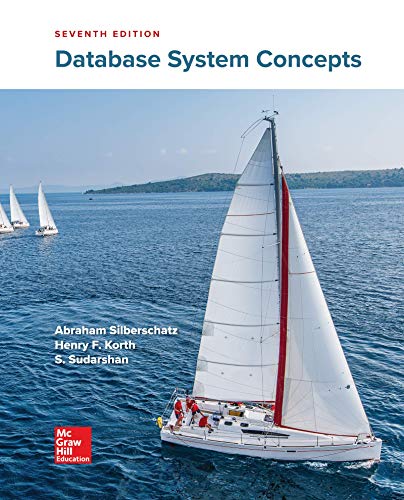
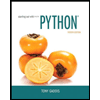
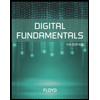
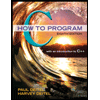
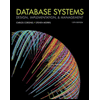
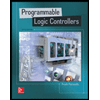