Sudoku gives the player a 9 by 9 grid and requires them to fill all elements with the numbers 1 through 9. You cannot place duplicate values in the same row, column or 3x3 section. Input Format 9 rows of 9 space separated integers between 0 and 9. If the value is 0, that means the space is to be filled in with a value between 1 and 9. If the value is already between 1 and 9, those elements cannot be changed. Constraints values are between 0 and 9 Output Format output the results of the completed sudoku puzzle in nine rows. Each element separated by a space. If no solution exists, output "No solution exists" Note: you might want to create a global flag (boolean) that would indicate whether or not the solution has been found. Initialize it to false, when it comes time to call validSolution(), you could set it to true. This way, when you return to main, you could check the flag and determine whether or not a solution was found. Sample Input 0 0 0 0 0 4 0 0 0 0 9 0 0 0 0 1 2 7 0 3 0 5 2 6 0 0 0 0 00005030 2 0 0 2 4 0 8 700 70 60 20 000 0003 908 0 4 0 8 3 0 0 2 0 0 1 0 0 0 0 1 0 0 0 0 Sample Output 0 8 2 7 9 4 9 6 4 5 8 1 3 1 5 2 6 7 489 1 4 8 7 5 6 39 2 5 9 2 4 3 8 7 1 6 29548 7 3 6 1 6 7 1 3 4 8 3 6 2 5 9 8 9 5 8 24 7 2 9 5 1 1 4 6 3 7 Explanation 0 Print the solution with a space on either side of the numbers. This will give you two spaces between each number. If you think of the solution as an 81 digit number, with the top left number being the most significant digit and the lower right as the least significant digit, then in the cases of multiple solutions, print the one with the smallest value. 3 1 6 5 27 3 1 import java.io.*; 2 import java.util. *; 3 import java.text.*; 4 import java.math. *; 5 import java.util.regex.*; 6 public class Solution { 7 8 9▾ 10 11 ▼ 12▾ 13▾ 14▾ 15 16 17 18 } public static void main(String[] args) { Scanner in = new Scanner (System.in); int[][] a = new int [9] [9]; for (int a_i=0; a_i<9; a_i++) { } for (int a_j=0; a_j < 9; a_j++) { a[a_i] [a_j] = in.nextInt (); } Java 8
Sudoku gives the player a 9 by 9 grid and requires them to fill all elements with the numbers 1 through 9. You cannot place duplicate values in the same row, column or 3x3 section. Input Format 9 rows of 9 space separated integers between 0 and 9. If the value is 0, that means the space is to be filled in with a value between 1 and 9. If the value is already between 1 and 9, those elements cannot be changed. Constraints values are between 0 and 9 Output Format output the results of the completed sudoku puzzle in nine rows. Each element separated by a space. If no solution exists, output "No solution exists" Note: you might want to create a global flag (boolean) that would indicate whether or not the solution has been found. Initialize it to false, when it comes time to call validSolution(), you could set it to true. This way, when you return to main, you could check the flag and determine whether or not a solution was found. Sample Input 0 0 0 0 0 4 0 0 0 0 9 0 0 0 0 1 2 7 0 3 0 5 2 6 0 0 0 0 00005030 2 0 0 2 4 0 8 700 70 60 20 000 0003 908 0 4 0 8 3 0 0 2 0 0 1 0 0 0 0 1 0 0 0 0 Sample Output 0 8 2 7 9 4 9 6 4 5 8 1 3 1 5 2 6 7 489 1 4 8 7 5 6 39 2 5 9 2 4 3 8 7 1 6 29548 7 3 6 1 6 7 1 3 4 8 3 6 2 5 9 8 9 5 8 24 7 2 9 5 1 1 4 6 3 7 Explanation 0 Print the solution with a space on either side of the numbers. This will give you two spaces between each number. If you think of the solution as an 81 digit number, with the top left number being the most significant digit and the lower right as the least significant digit, then in the cases of multiple solutions, print the one with the smallest value. 3 1 6 5 27 3 1 import java.io.*; 2 import java.util. *; 3 import java.text.*; 4 import java.math. *; 5 import java.util.regex.*; 6 public class Solution { 7 8 9▾ 10 11 ▼ 12▾ 13▾ 14▾ 15 16 17 18 } public static void main(String[] args) { Scanner in = new Scanner (System.in); int[][] a = new int [9] [9]; for (int a_i=0; a_i<9; a_i++) { } for (int a_j=0; a_j < 9; a_j++) { a[a_i] [a_j] = in.nextInt (); } Java 8
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Hi I need help in writing a java program that takes in a matrix 9 by 9. Where values input as zeros should be filled following the rules of the Sudoku puzzle. The solution should be printed out as a matrix of 9 by 9. Attached is the code for the input matrix from the scanner and additional details for the program. Thanks
![Sudoku gives the player a 9 by 9 grid and requires them to fill all elements with the
numbers 1 through 9.
You cannot place duplicate values in the same row, column or 3x3 section.
Input Format
9 rows of 9 space separated integers between 0 and 9.
If the value is 0, that means the space is to be filled in with a value between 1 and 9. If the
value is already between 1 and 9, those elements cannot be changed.
Constraints
values are between 0 and 9
Output Format
output the results of the completed sudoku puzzle in nine rows. Each element separated
by a space.
If no solution exists, output "No solution exists"
Note: you might want to create a global flag (boolean) that would indicate whether or not
the solution has been found. Initialize it to false, when it comes time to call validSolution(),
you could set it to true. This way, when you return to main, you could check the flag and
determine whether or not a solution was found.
Sample Input 0
0 0 0 0 4 0 0 0 0
9 0 0 0 0 1 2 7 0
3 0 5 2 600 00
000050302
0 0 2 4 0 8 700
70 60 20 000
0003 908 0 4
0 8 3 0 0 2 0 0 1
0 0 0 0 1 0 0 0 0
Sample Output 0
8
2
7 94
3 1 6 5
9 6 4 5 8
12 7 3
3 1 5 2 6 7 489
1 4 8 7 5 6 39 2
5 9 2 4
3 8 7 1 6
29548
7 3 6 1
1
4 8 3 6
6 7
3
9 5 8 24
7 2 9 5 1
1 4 6 3 7
2 5 9 8
Explanation 0
Print the solution with a space on either side of the numbers. This will give you two spaces
between each number.
If you think of the solution as an 81 digit number, with the top left number being the most
significant digit and the lower right as the least significant digit, then in the cases of
multiple solutions, print the one with the smallest value.
1 import java.io.*;
2 import java.util.*;
3 import java.text.*;
4 import java.math. *;
5 import java.util.regex.*;
6
7▾ public class Solution {
8
9▾
10
11▾
12▾
13▾
14▾
15
16
17
18 }
public static void main(String[] args) {
Scanner in = new Scanner (System.in);
int[][] a = new int [9] [9];
}
for (int a_i=0; a_i<9; a_i++) {
}
for (int a_j=0; a_j < 9; a_j++) {
a[a_i] [a_j] = in.nextInt ();
}
Java 8](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2F0d957949-097d-4652-9e12-2b9f50f3cfe7%2Fsaampcq9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Sudoku gives the player a 9 by 9 grid and requires them to fill all elements with the
numbers 1 through 9.
You cannot place duplicate values in the same row, column or 3x3 section.
Input Format
9 rows of 9 space separated integers between 0 and 9.
If the value is 0, that means the space is to be filled in with a value between 1 and 9. If the
value is already between 1 and 9, those elements cannot be changed.
Constraints
values are between 0 and 9
Output Format
output the results of the completed sudoku puzzle in nine rows. Each element separated
by a space.
If no solution exists, output "No solution exists"
Note: you might want to create a global flag (boolean) that would indicate whether or not
the solution has been found. Initialize it to false, when it comes time to call validSolution(),
you could set it to true. This way, when you return to main, you could check the flag and
determine whether or not a solution was found.
Sample Input 0
0 0 0 0 4 0 0 0 0
9 0 0 0 0 1 2 7 0
3 0 5 2 600 00
000050302
0 0 2 4 0 8 700
70 60 20 000
0003 908 0 4
0 8 3 0 0 2 0 0 1
0 0 0 0 1 0 0 0 0
Sample Output 0
8
2
7 94
3 1 6 5
9 6 4 5 8
12 7 3
3 1 5 2 6 7 489
1 4 8 7 5 6 39 2
5 9 2 4
3 8 7 1 6
29548
7 3 6 1
1
4 8 3 6
6 7
3
9 5 8 24
7 2 9 5 1
1 4 6 3 7
2 5 9 8
Explanation 0
Print the solution with a space on either side of the numbers. This will give you two spaces
between each number.
If you think of the solution as an 81 digit number, with the top left number being the most
significant digit and the lower right as the least significant digit, then in the cases of
multiple solutions, print the one with the smallest value.
1 import java.io.*;
2 import java.util.*;
3 import java.text.*;
4 import java.math. *;
5 import java.util.regex.*;
6
7▾ public class Solution {
8
9▾
10
11▾
12▾
13▾
14▾
15
16
17
18 }
public static void main(String[] args) {
Scanner in = new Scanner (System.in);
int[][] a = new int [9] [9];
}
for (int a_i=0; a_i<9; a_i++) {
}
for (int a_j=0; a_j < 9; a_j++) {
a[a_i] [a_j] = in.nextInt ();
}
Java 8
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
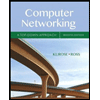
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
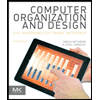
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
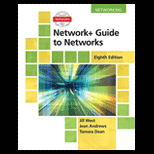
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
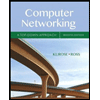
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
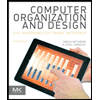
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
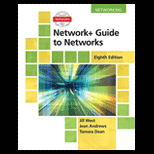
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
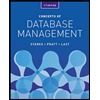
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
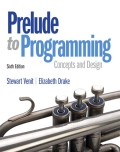
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
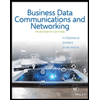
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY