task 13.1 Follow these steps: ● Modify the existing Animal.java file for this task. ● Using the Lion class template, as shown in this pdf file, expand the class to have features specific to a lion: ○ Add a field for lion type (cub, male, female). ○ Add a method in this class which sets the lion type based on its weight (note that the weight is a derived field from the superclass). ○ If the weight is less than 80kg, it’s type should be a cub. If less than 120kg, it should be female. If greater than 120kg, it is a male. ○ Include a method that will print out a description of a lion object. ● Compile, save and run your file. Compulsory Task 2 Follow these steps: ● Modify the existing Animal.java file for this task. ● Create a class called ‘Cheetah’ that: ○ Inherits from the Animal class. ○ Makes use of at least one static field which needs to have a static setter and getter. ○ Contains a constructor. ○ Contains a toString() method. ○ Has an array as one of its fields. ● Create an application class. Within the main method, create a Cheetah object and print out (to the screen) the details that describe this object. ● Compile,
task 13.1
Follow these steps:
● Modify the existing Animal.java file for this task.
● Using the Lion class template, as shown in this pdf file, expand the class to
have features specific to a lion:
○ Add a field for lion type (cub, male, female).
○ Add a method in this class which sets the lion type based on its
weight (note that the weight is a derived field from the superclass).
○ If the weight is less than 80kg, it’s type should be a cub. If less than
120kg, it should be female. If greater than 120kg, it is a male.
○ Include a method that will print out a description of a lion object.
● Compile, save and run your file.
Compulsory Task 2
Follow these steps:
● Modify the existing Animal.java file for this task.
● Create a class called ‘Cheetah’ that:
○ Inherits from the Animal class.
○ Makes use of at least one static field which needs to have a static
setter and getter.
○ Contains a constructor.
○ Contains a toString() method.
○ Has an array as one of its fields.
● Create an application class. Within the main method, create a Cheetah
object and print out (to the screen) the details that describe this object.
● Compile,
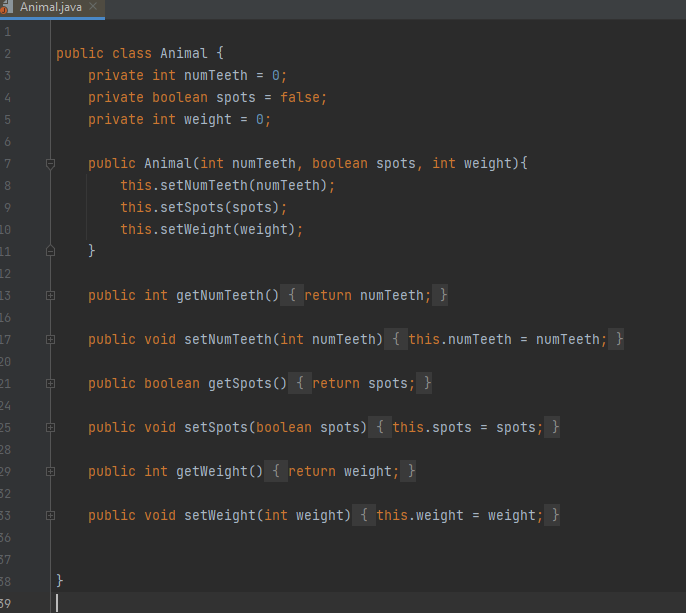
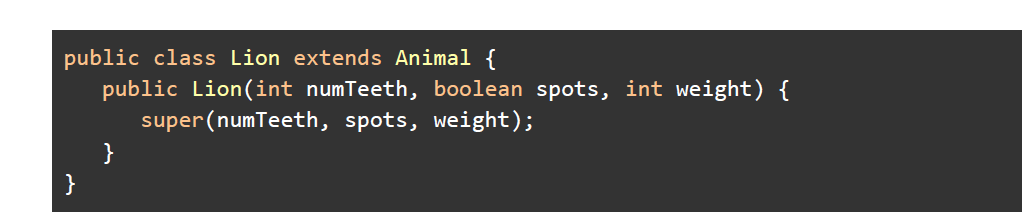

Step by step
Solved in 4 steps with 6 images

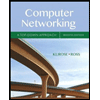
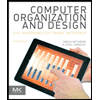
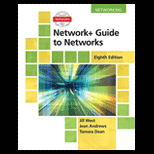
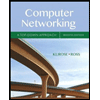
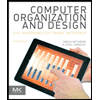
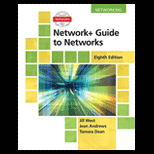
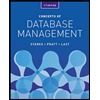
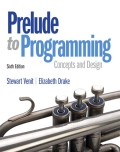
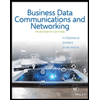