Task #3 Writing Output to a File 1. Copy the files StatsDemo.java (see Code Listing 4.2) and Numbers.txt from the Student Files or as directed by your instructor. 2. First we will write output to a file: a. Create a FileWriter object passing it the filename Results.txt (Don’t forget the needed import statement). b. Create a PrintWriter object passing it the FileWriter object. c. Since you are using a FileWriter object, add a throws clause to the main method header. d. Print the mean and standard deviation to the output file using a three decimal format, labeling each. e. Close the output file. 3. Compile, debug, and run. You will need to type in the filename Numbers.txt. You should get no output to the console, but running the program will create a file called Results.txt with your output. The output you should get at this point is: mean = 0.000, standard deviation = 0.000. This is not the correct mean or standard deviation for the data, but we will fix this in the next tasks. Task #4 Calculating the Mean 1. Now we need to add lines to allow us to read from the input file and calculate the mean. a. Create a FileReader object passing it the filename. b. Create a BufferedReader object passing it the FileReader object. 2. Write a priming read to read the first line of the file. 3. Write a loop that continues until you are at the end of the file. 4. The body of the loop will: a. convert the line into a double value and add the value to the accumulator b. increment the counter c. read a new line from the file 5. When the program exits the loop close the input file. 6. Calculate and store the mean. The mean is calculated by dividing the accumulator by the counter. 7. Compile, debug, and run. You should now get a mean of 77.444, but the standard deviation will still be 0.000. Task #5 Calculating the Standard Deviation 1. We need to reconnect to the file so we can start reading from the top again. a. Create a FileReader object passing it the filename. b. Create a BufferedReader object passing it the FileReader object. 2. Reinitialize the sum and count to 0. 3. Write a priming read to read the first line of the file. 4. Write a loop that continues until you are at the end of the file. 5. The body of the loop will: a. convert the line into a double value and subtract the mean, store the result in difference b. add the square of the difference to the accumulator c. increment the counter d. read a newline from the file. 6. When the program exits the loop close the input file. 7. The variance is calculated by dividing the accumulator (sum of the squares of the difference) by the counter. Calculate the standard deviation by taking the square root of the variance (Use the Math.sqrt method to take the square root). 8. Compile, debug, and run. You should get a mean of 77.444 and standard deviation of 10.021. Code Listing 4.2 (StatsDemo.java) import java.util.Scanner; // TASK #3 Add the file I/O import statement here /** This class reads numbers from a file, calculates the mean and standard deviation, and writes the results to a file. */ public class StatsDemo { // TASK #3 Add the throws clause public static void main(String[] args) { double sum = 0; // The sum of the numbers int count = 0; // The number of numbers added double mean = 0; // The average of the numbers double stdDev = 0; // The standard deviation String line; // To hold a line from the file double difference; // The value and mean difference // Create an object of type Scanner Scanner keyboard = new Scanner (System.in); String filename; // The user input file name // Prompt the user and read in the file name System.out.println("This program calculates " + "statistics on a file " + "containing a series of numbers"); System.out.print("Enter the file name: "); filename = keyboard.nextLine(); // ADD LINES FOR TASK #4 HERE // Create a FileReader object passing it the filename // Create a BufferedReader object passing FileReader // object // Perform a priming read to read the first line of // the file // Loop until you are at the end of the file // Convert the line to a double value and add the // value to sum // Increment the counter // Read a new line from the file // Close the input file // Store the calculated mean // ADD LINES FOR TASK #5 HERE // Reconnect FileReader object passing it the // filename // Reconnect BufferedReader object passing // FileReader object // Reinitialize the sum of the numbers // Reinitialize the number of numbers added // Perform a priming read to read the first line of // the file // Loop until you are at the end of the file // Convert the line into a double value and // subtract the mean // Add the square of the difference to the sum // Increment the counter // Read a new line from the file // Close the input file // Store the calculated standard deviation // ADD LINES FOR TASK #3 HERE // Create a FileWriter object using "Results.txt" // Create a PrintWriter object passing the // FileWriter object // Print the results to the output file // Close the output file } }
Task #3 Writing Output to a File 1. Copy the files StatsDemo.java (see Code Listing 4.2) and Numbers.txt from the Student Files or as directed by your instructor. 2. First we will write output to a file: a. Create a FileWriter object passing it the filename Results.txt (Don’t forget the needed import statement). b. Create a PrintWriter object passing it the FileWriter object. c. Since you are using a FileWriter object, add a throws clause to the main method header. d. Print the mean and standard deviation to the output file using a three decimal format, labeling each. e. Close the output file. 3. Compile, debug, and run. You will need to type in the filename Numbers.txt. You should get no output to the console, but running the program will create a file called Results.txt with your output. The output you should get at this point is: mean = 0.000, standard deviation = 0.000. This is not the correct mean or standard deviation for the data, but we will fix this in the next tasks. Task #4 Calculating the Mean 1. Now we need to add lines to allow us to read from the input file and calculate the mean. a. Create a FileReader object passing it the filename. b. Create a BufferedReader object passing it the FileReader object. 2. Write a priming read to read the first line of the file. 3. Write a loop that continues until you are at the end of the file. 4. The body of the loop will: a. convert the line into a double value and add the value to the accumulator b. increment the counter c. read a new line from the file 5. When the program exits the loop close the input file. 6. Calculate and store the mean. The mean is calculated by dividing the accumulator by the counter. 7. Compile, debug, and run. You should now get a mean of 77.444, but the standard deviation will still be 0.000. Task #5 Calculating the Standard Deviation 1. We need to reconnect to the file so we can start reading from the top again. a. Create a FileReader object passing it the filename. b. Create a BufferedReader object passing it the FileReader object. 2. Reinitialize the sum and count to 0. 3. Write a priming read to read the first line of the file. 4. Write a loop that continues until you are at the end of the file. 5. The body of the loop will: a. convert the line into a double value and subtract the mean, store the result in difference b. add the square of the difference to the accumulator c. increment the counter d. read a newline from the file. 6. When the program exits the loop close the input file. 7. The variance is calculated by dividing the accumulator (sum of the squares of the difference) by the counter. Calculate the standard deviation by taking the square root of the variance (Use the Math.sqrt method to take the square root). 8. Compile, debug, and run. You should get a mean of 77.444 and standard deviation of 10.021. Code Listing 4.2 (StatsDemo.java) import java.util.Scanner; // TASK #3 Add the file I/O import statement here /** This class reads numbers from a file, calculates the mean and standard deviation, and writes the results to a file. */ public class StatsDemo { // TASK #3 Add the throws clause public static void main(String[] args) { double sum = 0; // The sum of the numbers int count = 0; // The number of numbers added double mean = 0; // The average of the numbers double stdDev = 0; // The standard deviation String line; // To hold a line from the file double difference; // The value and mean difference // Create an object of type Scanner Scanner keyboard = new Scanner (System.in); String filename; // The user input file name // Prompt the user and read in the file name System.out.println("This program calculates " + "statistics on a file " + "containing a series of numbers"); System.out.print("Enter the file name: "); filename = keyboard.nextLine(); // ADD LINES FOR TASK #4 HERE // Create a FileReader object passing it the filename // Create a BufferedReader object passing FileReader // object // Perform a priming read to read the first line of // the file // Loop until you are at the end of the file // Convert the line to a double value and add the // value to sum // Increment the counter // Read a new line from the file // Close the input file // Store the calculated mean // ADD LINES FOR TASK #5 HERE // Reconnect FileReader object passing it the // filename // Reconnect BufferedReader object passing // FileReader object // Reinitialize the sum of the numbers // Reinitialize the number of numbers added // Perform a priming read to read the first line of // the file // Loop until you are at the end of the file // Convert the line into a double value and // subtract the mean // Add the square of the difference to the sum // Increment the counter // Read a new line from the file // Close the input file // Store the calculated standard deviation // ADD LINES FOR TASK #3 HERE // Create a FileWriter object using "Results.txt" // Create a PrintWriter object passing the // FileWriter object // Print the results to the output file // Close the output file } }
Chapter14: Files And Streams
Section: Chapter Questions
Problem 2E: Create a program named FileComparison that compares two files. First, use a text editor such as...
Related questions
Question
Task #3 Writing Output to a File
1. Copy the files StatsDemo.java (see Code Listing 4.2) and Numbers.txt from the
Student Files or as directed by your instructor.
2. First we will write output to a file:
a. Create a FileWriter object passing it the filename Results.txt (Don’t
forget the needed import statement).
b. Create a PrintWriter object passing it the FileWriter object.
c. Since you are using a FileWriter object, add a throws clause to the
main method header.
d. Print the mean and standard deviation to the output file using a three
decimal format, labeling each.
e. Close the output file.
3. Compile, debug, and run. You will need to type in the filename Numbers.txt. You
should get no output to the console, but running the program will create a file
called Results.txt with your output. The output you should get at this point is:
mean = 0.000, standard deviation = 0.000. This is not the correct mean or
standard deviation for the data, but we will fix this in the next tasks.
Task #4 Calculating the Mean
1. Now we need to add lines to allow us to read from the input file and calculate the
mean.
a. Create a FileReader object passing it the filename.
b. Create a BufferedReader object passing it the FileReader object.
2. Write a priming read to read the first line of the file.
3. Write a loop that continues until you are at the end of the file.
4. The body of the loop will:
a. convert the line into a double value and add the value to the accumulator
b. increment the counter
c. read a new line from the file
5. When the program exits the loop close the input file.
6. Calculate and store the mean. The mean is calculated by dividing the accumulator
by the counter.
7. Compile, debug, and run. You should now get a mean of 77.444, but the standard
deviation will still be 0.000.
Task #5 Calculating the Standard Deviation
1. We need to reconnect to the file so we can start reading from the top again.
a. Create a FileReader object passing it the filename.
b. Create a BufferedReader object passing it the FileReader object.
2. Reinitialize the sum and count to 0.
3. Write a priming read to read the first line of the file.
1. Copy the files StatsDemo.java (see Code Listing 4.2) and Numbers.txt from the
Student Files or as directed by your instructor.
2. First we will write output to a file:
a. Create a FileWriter object passing it the filename Results.txt (Don’t
forget the needed import statement).
b. Create a PrintWriter object passing it the FileWriter object.
c. Since you are using a FileWriter object, add a throws clause to the
main method header.
d. Print the mean and standard deviation to the output file using a three
decimal format, labeling each.
e. Close the output file.
3. Compile, debug, and run. You will need to type in the filename Numbers.txt. You
should get no output to the console, but running the program will create a file
called Results.txt with your output. The output you should get at this point is:
mean = 0.000, standard deviation = 0.000. This is not the correct mean or
standard deviation for the data, but we will fix this in the next tasks.
Task #4 Calculating the Mean
1. Now we need to add lines to allow us to read from the input file and calculate the
mean.
a. Create a FileReader object passing it the filename.
b. Create a BufferedReader object passing it the FileReader object.
2. Write a priming read to read the first line of the file.
3. Write a loop that continues until you are at the end of the file.
4. The body of the loop will:
a. convert the line into a double value and add the value to the accumulator
b. increment the counter
c. read a new line from the file
5. When the program exits the loop close the input file.
6. Calculate and store the mean. The mean is calculated by dividing the accumulator
by the counter.
7. Compile, debug, and run. You should now get a mean of 77.444, but the standard
deviation will still be 0.000.
Task #5 Calculating the Standard Deviation
1. We need to reconnect to the file so we can start reading from the top again.
a. Create a FileReader object passing it the filename.
b. Create a BufferedReader object passing it the FileReader object.
2. Reinitialize the sum and count to 0.
3. Write a priming read to read the first line of the file.
4. Write a loop that continues until you are at the end of the file.
5. The body of the loop will:
a. convert the line into a double value and subtract the mean, store the result
in difference
b. add the square of the difference to the accumulator
c. increment the counter
d. read a newline from the file.
6. When the program exits the loop close the input file.
7. The variance is calculated by dividing the accumulator (sum of the squares of the
difference) by the counter. Calculate the standard deviation by taking the square
root of the variance (Use the Math.sqrt method to take the square root).
8. Compile, debug, and run. You should get a mean of 77.444 and standard
deviation of 10.021.
Code Listing 4.2 (StatsDemo.java)
import java.util.Scanner;
// TASK #3 Add the file I/O import statement here
/**
This class reads numbers from a file, calculates the
mean and standard deviation, and writes the results
to a file.
*/
public class StatsDemo
{
// TASK #3 Add the throws clause
public static void main(String[] args)
{
double sum = 0; // The sum of the numbers
int count = 0; // The number of numbers added
double mean = 0; // The average of the numbers
double stdDev = 0; // The standard deviation
String line; // To hold a line from the file
double difference; // The value and mean difference
5. The body of the loop will:
a. convert the line into a double value and subtract the mean, store the result
in difference
b. add the square of the difference to the accumulator
c. increment the counter
d. read a newline from the file.
6. When the program exits the loop close the input file.
7. The variance is calculated by dividing the accumulator (sum of the squares of the
difference) by the counter. Calculate the standard deviation by taking the square
root of the variance (Use the Math.sqrt method to take the square root).
8. Compile, debug, and run. You should get a mean of 77.444 and standard
deviation of 10.021.
Code Listing 4.2 (StatsDemo.java)
import java.util.Scanner;
// TASK #3 Add the file I/O import statement here
/**
This class reads numbers from a file, calculates the
mean and standard deviation, and writes the results
to a file.
*/
public class StatsDemo
{
// TASK #3 Add the throws clause
public static void main(String[] args)
{
double sum = 0; // The sum of the numbers
int count = 0; // The number of numbers added
double mean = 0; // The average of the numbers
double stdDev = 0; // The standard deviation
String line; // To hold a line from the file
double difference; // The value and mean difference
// Create an object of type Scanner
Scanner keyboard = new Scanner (System.in);
String filename; // The user input file name
// Prompt the user and read in the file name
System.out.println("This program calculates " +
"statistics on a file " +
"containing a series of numbers");
System.out.print("Enter the file name: ");
filename = keyboard.nextLine();
// ADD LINES FOR TASK #4 HERE
// Create a FileReader object passing it the filename
// Create a BufferedReader object passing FileReader
// object
// Perform a priming read to read the first line of
// the file
// Loop until you are at the end of the file
// Convert the line to a double value and add the
// value to sum
// Increment the counter
// Read a new line from the file
// Close the input file
// Store the calculated mean
// ADD LINES FOR TASK #5 HERE
// Reconnect FileReader object passing it the
// filename
// Reconnect BufferedReader object passing
// FileReader object
// Reinitialize the sum of the numbers
// Reinitialize the number of numbers added
// Perform a priming read to read the first line of
// the file
// Loop until you are at the end of the file
// Convert the line into a double value and
// subtract the mean
// Add the square of the difference to the sum
// Increment the counter
// Read a new line from the file
// Close the input file
// Store the calculated standard deviation
// ADD LINES FOR TASK #3 HERE
// Create a FileWriter object using "Results.txt"
// Create a PrintWriter object passing the
Scanner keyboard = new Scanner (System.in);
String filename; // The user input file name
// Prompt the user and read in the file name
System.out.println("This program calculates " +
"statistics on a file " +
"containing a series of numbers");
System.out.print("Enter the file name: ");
filename = keyboard.nextLine();
// ADD LINES FOR TASK #4 HERE
// Create a FileReader object passing it the filename
// Create a BufferedReader object passing FileReader
// object
// Perform a priming read to read the first line of
// the file
// Loop until you are at the end of the file
// Convert the line to a double value and add the
// value to sum
// Increment the counter
// Read a new line from the file
// Close the input file
// Store the calculated mean
// ADD LINES FOR TASK #5 HERE
// Reconnect FileReader object passing it the
// filename
// Reconnect BufferedReader object passing
// FileReader object
// Reinitialize the sum of the numbers
// Reinitialize the number of numbers added
// Perform a priming read to read the first line of
// the file
// Loop until you are at the end of the file
// Convert the line into a double value and
// subtract the mean
// Add the square of the difference to the sum
// Increment the counter
// Read a new line from the file
// Close the input file
// Store the calculated standard deviation
// ADD LINES FOR TASK #3 HERE
// Create a FileWriter object using "Results.txt"
// Create a PrintWriter object passing the
// FileWriter object
// Print the results to the output file
// Close the output file
}
}
// Print the results to the output file
// Close the output file
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
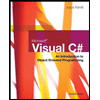
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
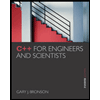
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
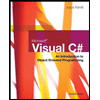
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
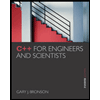
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr