Task - Encode a string (C Language) Modify Project #2, Task #1 (given below) so that input characters are command line arguments. Requirements Name your program project5_encode.c. Input characters are command line arguments. There can be any number of command line arguments. Assume the total number of input characters is no more than 1000. Character handling library functions in ctype.h are allowed. The program should include the following function: void encode(char *input, char *output); The function expects input to point to a string containing the string to be encoded, output to point to a string containing the result. The program should also check if the number of arguments on the command line is greater than or equal to 2. If the number of arguments is 1, the program should display the message "Invalid input!". Examples (your program must follow this format precisely) Example #1 $ ./a.out 7 + 8 Output: 3_4 Example #2 $ ./a.out usf.edu Output: ayl_kja Example #3 $ ./a.out Invalid input! Project #2, Task #1 Task - Encode a string (C Languege) Write a program that encodes the input characters using the following algorithm. if the input character is a digit ('0' through '9'), then output the digit by adding 6 to the digit and calculate the remainder by 10. For example, if a digit is '6', then replaced digit is '2'. Subtract the digit with '0', add 6, and then calculate the remainder by 10, then add '0'. If the character is an alphabetic letter, then shift the letter by 6. For example, if the letter is ‘B’, then the letter becomes 'H'. Subtract the letter with 'a' (for lower case) or 'A' (for uppder case), add 6, and then calculate the remainder by 26, then add 'a' (for lower case) or 'A' (for uppder case). For example, 'X' shifted by 6 is 'D'. If the character is a white space, skip it. If the character is not a digit, an alphabetic letter, or a white space, output the underscore '_' character. Requirements Follow the format of the examples below. Use getchar() function to read in the input. Do not use scanf. The user input ends with the user pressing the enter key (a new line character). Character handling library functions in ctype.h are allowed. Examples (your program must follow this format precisely) Example #1 Enter input: 7 + 8 3_4 Example #2 Enter input: usf.edu ayl_kja my answer: #include #include int main() { //create a character char num; //print enter input for the user to enter their response printf("Enter input: "); while ((num = getchar()) != '\n') { //if inputs are numbers digit print add 6 to it and calculate remainder by 10. if (isdigit(num)) { printf("%c",(num-'0'+6)%10+'0'); } //if the inputs are letters then shift to the 6th letter from it and calculate the remainder by 26. else if (isalpha(num)) { //if input is in lower case letters then print the output in lower case, else if it is in upper case letters then print output in upper case. if (tolower(num)) { printf("%c",(num-'a'+6)%26+'a'); } else { printf("%c", (num-'A'+6)%26+'A'); } } //if character is white space skip else print '_' else if (isspace(num)) { continue; } else { printf("_"); } } return 0; }
Task - Encode a string (C Language)
Modify Project #2, Task #1 (given below) so that input characters are command line arguments.
Requirements
- Name your program project5_encode.c.
- Input characters are command line arguments. There can be any number of command line arguments.
- Assume the total number of input characters is no more than 1000.
- Character handling library functions in ctype.h are allowed.
- The program should include the following function:
void encode(char *input, char *output);
-
- The function expects input to point to a string containing the string to be encoded, output to point to a string containing the result.
- The program should also check if the number of arguments on the command line is greater than or equal to 2. If the number of arguments is 1, the program should display the message "Invalid input!".
Examples (your program must follow this format precisely)
Example #1
$ ./a.out 7 + 8
Output: 3_4
Example #2
$ ./a.out usf.edu
Output: ayl_kja
Example #3
$ ./a.out
Invalid input!
Project #2, Task #1
Task - Encode a string (C Languege)
Write a program that encodes the input characters using the following
- if the input character is a digit ('0' through '9'), then output the digit by adding 6 to the digit and calculate the remainder by 10. For example, if a digit is '6', then replaced digit is '2'.
- Subtract the digit with '0', add 6, and then calculate the remainder by 10, then add '0'.
- If the character is an alphabetic letter, then shift the letter by 6. For example, if the letter is ‘B’, then the letter becomes 'H'.
- Subtract the letter with 'a' (for lower case) or 'A' (for uppder case), add 6, and then calculate the remainder by 26, then add 'a' (for lower case) or 'A' (for uppder case). For example, 'X' shifted by 6 is 'D'.
- If the character is a white space, skip it.
- If the character is not a digit, an alphabetic letter, or a white space, output the underscore '_' character.
Requirements
- Follow the format of the examples below.
- Use getchar() function to read in the input. Do not use scanf.
- The user input ends with the user pressing the enter key (a new line character).
- Character handling library functions in ctype.h are allowed.
Examples (your program must follow this format precisely)
Example #1
Enter input: 7 + 8
3_4
Example #2
Enter input: usf.edu
ayl_kja
my answer:
#include <stdio.h>
#include <ctype.h>
int main()
{
//create a character
char num;
//print enter input for the user to enter their response
printf("Enter input: ");
while ((num = getchar()) != '\n')
{
//if inputs are numbers digit print add 6 to it and calculate remainder by 10.
if (isdigit(num))
{
printf("%c",(num-'0'+6)%10+'0');
}
//if the inputs are letters then shift to the 6th letter from it and calculate the remainder by 26.
else if (isalpha(num))
{
//if input is in lower case letters then print the output in lower case, else if it is in upper case letters then print output in upper case.
if (tolower(num))
{
printf("%c",(num-'a'+6)%26+'a');
}
else
{
printf("%c", (num-'A'+6)%26+'A');
}
}
//if character is white space skip else print '_'
else if (isspace(num))
{
continue;
}
else
{
printf("_");
}
}
return 0;
}

Step by step
Solved in 2 steps with 6 images

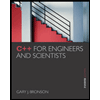
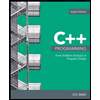
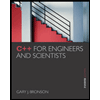
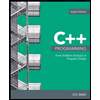