Test 9 > run Enter x and y coordinates of the 1st circle: 1.5 0.25 Enter radius of the 1st circle: 1.2 Enter x and y coordinates of the square: 0.3 -2.5 Enter width of the square: 5 Enter x and y coordinates of the 2nd circle: 1.6 -1.1 Enter radius of the 2nd circle: 0.5 Enter x and y coordinates of the query point: 1.6 -1.55 2nd circle and square contain point (1.6, -1.55). Test 10 > run Enter x and y coordinates of the 1st circle: 1.5 0.25 Enter radius of the 1st circle: 1.2 Enter x and y coordinates of the square: 0.3 2.5 Enter width of the square: 5 Enter x and y coordinates of the 2nd circle: 1.6 -1.1 Enter radius of the 2nd circle: 0.5 Enter x and y coordinates of the query point: 1.55 -0.85 Both circles contain point (1.55, -0.85). TASK 1: Study the lecture notes (Powerpoint slides and pre-recorded lectures) and assigned readings on Selection before you start. TASK 2: Replace "??" with your name, creation date, and a description of the program (synopsis). NOTE: DO NOT delete nor change the code already given to you in the code template, except for the comment /* INSERT YOUR CODE HERE */. You will insert your solution by replacing /* INSERT YOUR CODE HERE */. TASK 3: Write C++ code to prompt and read in the x and y coordinates of the first circle. Compile, run, and test this solution before continuing to the next task. TASK 4: Write C++ code to prompt and read in the radius of the first circle. Compile, run, and test this solution before continuing to the next task. TASK 5: Write C++ code to prompt and read in the x and y coordinates of the bottom left corner of the square. Compile, run, and test this solution before continuing to the next task. Continue to do this after EACH of the following tasks. TASK 6: Write C++ code to prompt and read in the width of the square. This will give you the length and height of the square. TASK 7: Write C++ code to prompt and read in the x and y coordinates of the second circle. TASK 8: Write C++ code to prompt and read in the radius of the second circle. TASK 9: Write C++ code to prompt and read in the x and y coordinates of the query point. Task 10: Write C++ code to determine which shapes contain the query point and output the correct message. i. Use the Pythagorean Theorem (see examples in the lecture notes) to determine whether a circle contains the query point with the equation square root{(x - cx)^2 + (y - cy)^2} <= r, where (x, y) is the query point, (cx, cy) is the circle center, r is the circle radius, and ^ means to take a value to a power. Though, the ^ is NOT a valid operator in C++. ii. Use two checks to determine whether the square contains the query point. Check that the x coordinate of the query point is between the x locations of the left and right sides of the square. Also, check that the y coordinate of the query point is between the y locations of the bottom and top sides of the square. Let (rx, ry) be the coordinates for the bottom left corner of the square, l be the length, and h be the height. The x location of the left side is rx. The x location of the right side is rx + l. The y location of the bottom side is ry. The y location of the top side is ry + h. iii. Output one of eight possible messages: All shapes contain point, Both circles contain point, 1st circle and square contain point, 1st circle contains point, 2nd circle and square contain point, 2nd circle contains point, Square contains point, or No shape contains point.
Test 9 > run Enter x and y coordinates of the 1st circle: 1.5 0.25 Enter radius of the 1st circle: 1.2 Enter x and y coordinates of the square: 0.3 -2.5 Enter width of the square: 5 Enter x and y coordinates of the 2nd circle: 1.6 -1.1 Enter radius of the 2nd circle: 0.5 Enter x and y coordinates of the query point: 1.6 -1.55 2nd circle and square contain point (1.6, -1.55).
Test 10 > run Enter x and y coordinates of the 1st circle: 1.5 0.25 Enter radius of the 1st circle: 1.2 Enter x and y coordinates of the square: 0.3 2.5 Enter width of the square: 5 Enter x and y coordinates of the 2nd circle: 1.6 -1.1 Enter radius of the 2nd circle: 0.5 Enter x and y coordinates of the query point: 1.55 -0.85 Both circles contain point (1.55, -0.85).
TASK 1: Study the lecture notes (Powerpoint slides and pre-recorded lectures) and assigned readings on Selection before you start.
TASK 2: Replace "??" with your name, creation date, and a description of the program (synopsis).
NOTE: DO NOT delete nor change the code already given to you in the code template, except for the comment /* INSERT YOUR CODE HERE */. You will insert your solution by replacing /* INSERT YOUR CODE HERE */.
TASK 3: Write C++ code to prompt and read in the x and y coordinates of the first circle. Compile, run, and test this solution before continuing to the next task.
TASK 4: Write C++ code to prompt and read in the radius of the first circle. Compile, run, and test this solution before continuing to the next task.
TASK 5: Write C++ code to prompt and read in the x and y coordinates of the bottom left corner of the square. Compile, run, and test this solution before continuing to the next task. Continue to do this after EACH of the following tasks.
TASK 6: Write C++ code to prompt and read in the width of the square. This will give you the length and height of the square.
TASK 7: Write C++ code to prompt and read in the x and y coordinates of the second circle.
TASK 8: Write C++ code to prompt and read in the radius of the second circle.
TASK 9: Write C++ code to prompt and read in the x and y coordinates of the query point.
Task 10: Write C++ code to determine which shapes contain the query point and output the correct message.
i. Use the Pythagorean Theorem (see examples in the lecture notes) to determine whether a circle contains the query point with the equation square root{(x - cx)^2 + (y - cy)^2} <= r, where (x, y) is the query point, (cx, cy) is the circle center, r is the circle radius, and ^ means to take a value to a power. Though, the ^ is NOT a valid operator in C++.
ii. Use two checks to determine whether the square contains the query point. Check that the x coordinate of the query point is between the x locations of the left and right sides of the square. Also, check that the y coordinate of the query point is between the y locations of the bottom and top sides of the square. Let (rx, ry) be the coordinates for the bottom left corner of the square, l be the length, and h be the height. The x location of the left side is rx. The x location of the right side is rx + l. The y location of the bottom side is ry. The y location of the top side is ry + h.
iii. Output one of eight possible messages: All shapes contain point, Both circles contain point, 1st circle and square contain point, 1st circle contains point, 2nd circle and square contain point, 2nd circle contains point, Square contains point, or No shape contains point.
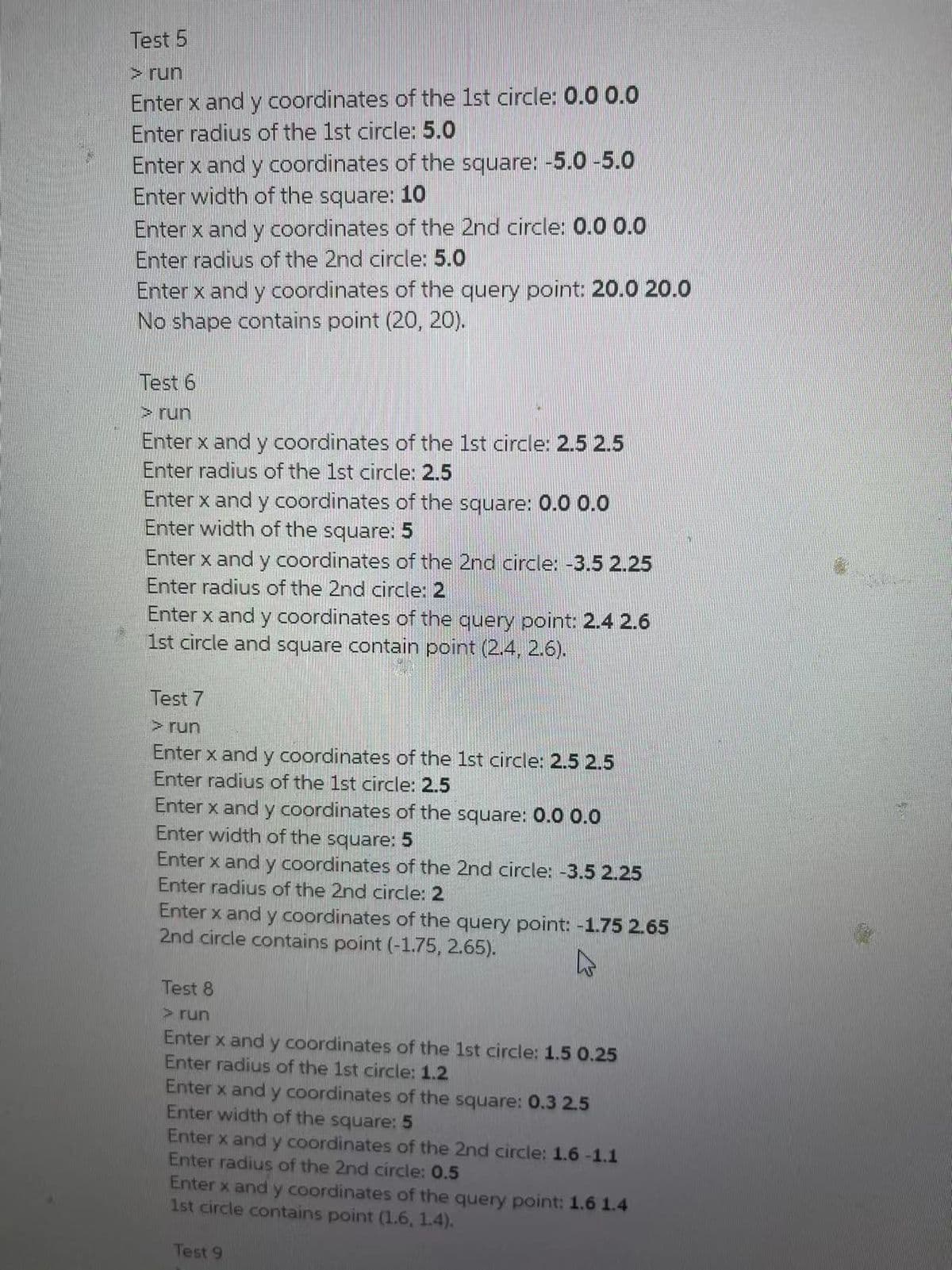
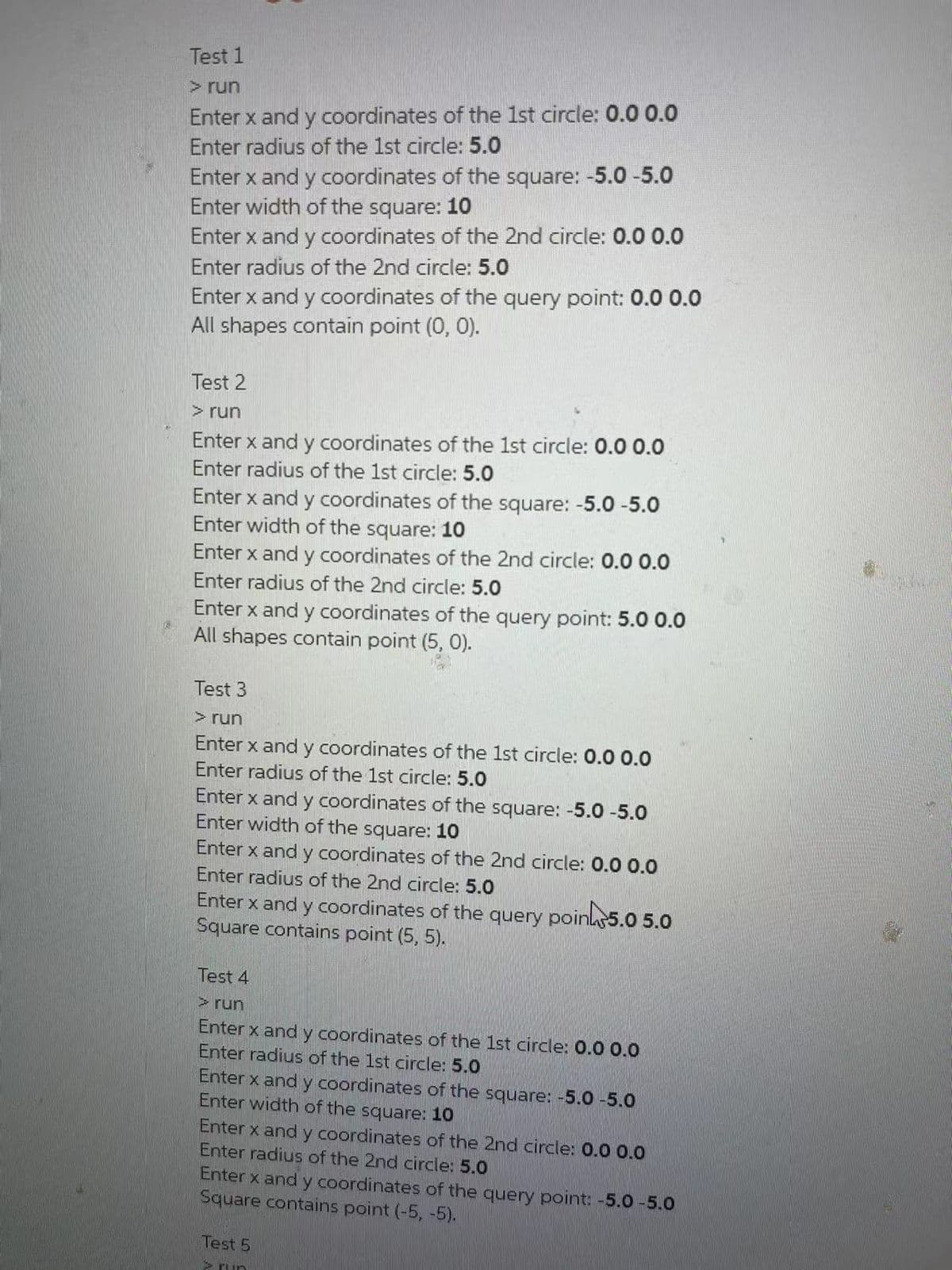

Given
Answer:
To check if a point P(x,) lies inside a circle a circle centered at point z(x1,y1) with radius r,just put the point P(x,y) in standard equation of circle and compare value with R.H.S.
if(x-x1)2+(y-y1)2 <=r2,point P lies inside the circle,else point P lies outside the circle
To check if a point P(x,y) lies inside a rectangle whose bottom left coordinate is (x1,y1),length l and height h:
check following 4 conditions:
1.x>=x1
2.x<=x+l
3.y>=y1
4.y<=y1+h
If the above 4 conditions are true,point P will lie inside the rectangle else point P will lie outside the rectangle
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

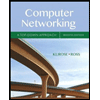
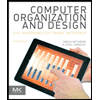
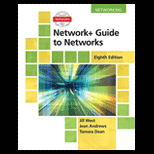
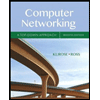
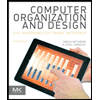
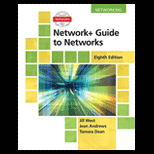
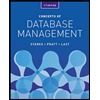
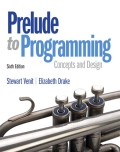
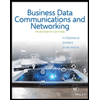