The bank will store a record for each account consisting of a customer number (an integer) representing the owner of the account, an account type (a single char: C for chequing, S for savings, and M for mutual fund), and the balance of the account (a double). These are kept in three arrays-an array of customer numbers, an array of types, and an array of balances. A sample of what this might look like appears below: Customer Array 102241 992944 992944 Type Array C S C Balance Array 1.29 22.50 1.24 The entries in the array correspond to one another-for example, the first slot in all three arrays represent the fact that customer number 102241 has a chequing account with $1.29 in it. As is shown, the same customer number can appear several times, as customers can have more than one account. These are called parallel arrays, because the slots in each correspond, and the really important thing about parallel arrays is that if you shuffle around anything in one array, you have to perform the same shuffles to the others. For example, say we delete one particular account. If we remove the entry from the customer array, but not the others, nothing matches any more and the data is worthless. Similarly, if we sort by balance, every data movement in the balance array must be duplicated in the others precisely, or once again the correspondence is lost. Since the array entries correspond to one another, we also need only keep track of the size (the number of entries used) once, since there will be the same number of entries in all three arrays. Your banking system will not do terribly much (by banking system standards!). When you start the program, it will look for a text file called bank.txt, open it, and load the three arrays with the data contained there. You can assume that bank.txt will contain one entry per line- the first customer number, then the account type on the next line, and the balance on the next line, then the next customer number, and so on. The data will not be in any particular order, but you can assume all data for each account will be present (that is, there’ll be no unmatching balances, etc.), and that the data will be error-free. Your bank will hold a maximum of 200 accounts, but you do NOT know how many will be in the file (you’ll have to count them as you read and keep track of the sizes of arrays). Once you have the data read in, you will prompt the user on the screen to enter commands (single characters). We will ignore the usual database update commands (creating, deleting accounts, etc), and concentrate only on selecting and viewing data. The valid commands your system will support are S (Search for an account), P (Print Total Balances), and Q (quit). You should error check these to ensure that other letters are not accepted (just print an error message in such cases and prompt for the command again). Your system will accept S and P commands until eventually the user enters a Quit command, causing the program to terminate. The P and S commands must operate as follows: When processing the Print Total Balances command, you will need to first ensure that the arrays representing the accounts are sorted by customer number so that all accounts for a particular customer appear together. Print Total Balances should thus begin by calling a Selection Sort method that you must write. You will have to slightly alter the one you saw in class, because you are using parallel arrays here. Remember, you have to keep the correspondence between slots: this means that while you’re sorting the customer numbers, you will have to perform identical swaps in all three arrays. After the array has been sorted, Print Total Balances will print ONE entry for each customer showing the TOTAL of ALL the accounts that customer has. You must only go through the arrays ONCE to do this, which shouldn’t at all be a problem once the data’s sorted. A Search command will allow the user to search for an account by account number, type, or both. The routine to process a search command will prompt the user for a customer number and account type. The user can either enter a valid value for these, or enter 0 for an account number or X for an account type. An account number of 0 or an account type of X will be considered a “don’t care” on the user’s part, allowing the search routine to display multiple results
The bank will store a record for each account consisting of a customer number (an integer) representing the owner of the account, an account type (a single char: C for chequing, S for savings, and M for mutual fund), and the balance of the account (a double). These are kept in three arrays-an array of customer numbers, an array of types, and an array of balances. A sample of what this might look like appears below:
Customer Array
102241 992944 992944Type Array
C S C
Balance Array
1.29 22.50 1.24
The entries in the array correspond to one another-for example, the first slot in all three arrays represent the fact that customer number 102241 has a chequing account with $1.29 in it. As is shown, the same customer number can appear several times, as customers can have more than one account. These are called parallel arrays, because the slots in each correspond, and the really important thing about parallel arrays is that if you shuffle around anything in one array, you have to perform the same shuffles to the others. For example, say we delete one particular account. If we remove the entry from the customer array, but not the others, nothing matches any more and the data is worthless. Similarly,
if we sort by balance, every data movement in the balance array must be duplicated in the others precisely, or once again the correspondence is lost. Since the array entries correspond to one another, we also need only keep track of the size (the number of entries used) once, since there will be the same number of entries in all three arrays.
Your banking system will not do terribly much (by banking system standards!). When you start the program, it will look for a text file called bank.txt, open it, and load the three arrays with the data contained there. You can assume that bank.txt will contain one entry per line- the first customer number, then the account type on the next line, and the balance on the next line, then the next customer number, and so on. The data will not be in any particular order, but you can assume all data for each account will be present (that is, there’ll be no unmatching balances, etc.), and that the data will be error-free. Your bank will hold a maximum of 200 accounts, but you do NOT know how many will be in the file (you’ll have to count them as you read and keep track of the sizes of arrays).
Once you have the data read in, you will prompt the user on the screen to enter commands (single characters). We will ignore the usual
When processing the Print Total Balances command, you will need to first ensure that the arrays representing the accounts are sorted by customer number so that all accounts for a particular customer appear together. Print Total Balances should thus begin by calling a Selection Sort method that you must write. You will have to slightly alter the one you saw in class, because you are using parallel arrays here. Remember, you have to keep the correspondence between slots: this means that while you’re sorting the customer numbers, you will have to perform identical swaps in all three arrays. After the array has been sorted, Print Total Balances will print ONE entry for each customer showing the TOTAL of ALL the accounts that customer has. You must only go through the arrays ONCE to do this, which shouldn’t at all be a problem once the data’s sorted.
A Search command will allow the user to search for an account by account number, type, or both. The routine to process a search command will prompt the user for a customer number and account type. The user can either enter a valid value for these, or enter 0 for an account number or X for an account type. An account number of 0 or an account type of X will be considered a “don’t care” on the user’s part, allowing the search routine to display multiple results.

Step by step
Solved in 2 steps with 4 images

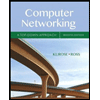
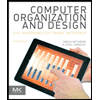
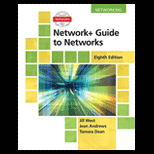
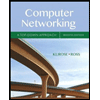
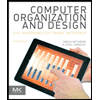
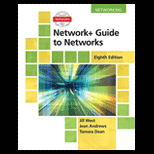
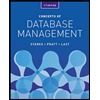
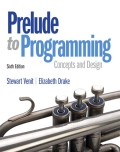
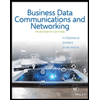