The Course class) Revise the Course class as follows: Revise the getStudents () method to return an array whose length is the same as the number of students in the course. (Hint: create a new array and copy students to it.) The array size is fixed in Listing 10.6. Revise the addStudent method to automatically increase the array size it there is no room to add more students. This is done by creating a new larger array and copying the contents of the current array to it. Implement the dropstudent method. Add a new method named clear ) that removes all students from the course. Class File public class CourseNew{ private String courseName; private String[] students = new String[100]; private int numberOfStudents; public Course(String courseName) { this.courseName = courseName; numberOfStudents = 0; } public void addStudent(String student) { if (numberOfStudents < 100) { students[numberOfStudents] = student; numberOfStudents++; } } public String[] getStudents() { String student[] = new String[numberOfStudents]; for (int index = 0; index < numberOfStudents; index++) { student[index] = this.students[index]; } return student; } public int getNumberOfStudents() { return numberOfStudents; } public String getCourseName() { return courseName; } public void dropStudent(String student) { if (numberOfStudents == 0 || numberOfStudents == 1) { students[0] = null; numberOfStudents = 0; return; } else { for (int index = 0; index < numberOfStudents; index++) { if (students[index].equals(student)) { students[index] = students[numberOfStudents - 1]; students[numberOfStudents - 1] = null; numberOfStudents--; } } } }
I need help with a Introduction to Java 11th edition book question with some modifications. The only difference is I have a test file to run the class file with I just need a class file that will run my test file. Below is my class file and test file I need them combined. Here's the question.
** 10.9 (The Course class) Revise the Course class as follows: Revise the getStudents () method to return an array whose length is the same as the number of students in the course. (Hint: create a new array and copy students to it.) The array size is fixed in Listing 10.6. Revise the addStudent method to automatically increase the array size it there is no room to add more students. This is done by creating a new larger array and copying the contents of the current array to it. Implement the dropstudent method. Add a new method named clear ) that removes all students from the course.
Class File
public class CourseNew{
private String courseName;
private String[] students = new String[100];
private int numberOfStudents;
public Course(String courseName) {
this.courseName = courseName;
numberOfStudents = 0;
}
public void addStudent(String student) {
if (numberOfStudents < 100) {
students[numberOfStudents] = student;
numberOfStudents++;
}
}
public String[] getStudents() {
String student[] = new String[numberOfStudents];
for (int index = 0; index < numberOfStudents; index++) {
student[index] = this.students[index];
}
return student;
}
public int getNumberOfStudents() {
return numberOfStudents;
}
public String getCourseName() {
return courseName;
}
public void dropStudent(String student) {
if (numberOfStudents == 0 || numberOfStudents == 1) {
students[0] = null;
numberOfStudents = 0;
return;
} else {
for (int index = 0; index < numberOfStudents; index++) {
if (students[index].equals(student)) {
students[index] = students[numberOfStudents - 1];
students[numberOfStudents - 1] = null;
numberOfStudents--;
}
}
}
}
Test File.
public class Exercise10_09 {
public static void main(String[] args) {
/** Load Courses and Students */
CourseNew course1 = new CourseNew("Data Structures");
CourseNew course2 = new CourseNew("
System.out.println(" ... Adding Students to Courses");
course1.addStudent("Peter Jones");
course1.addStudent("Brian Smith");
course1.addStudent("Anne Kennedy");
course1.addStudent("Susan Kennedy");
course1.addStudent("John Kennedy");
course1.addStudent("Kim Johnson");
course1.addStudent("Mary Brown");
course1.addStudent("Ken Greene");
course1.addStudent("Bennett Johnson");
course1.addStudent("Mike Wagner");
course1.addStudent("Cindy Shay");
course1.addStudent("Juan Conde");
course1.addStudent("Mark Mendon");
course2.addStudent("Peter Jones");
course2.addStudent("Steve Smith");
/** Print student list and # of students in the first course */
System.out.println("Number of students in course1: "
+ course1.getNumberOfStudents());
String[] students = course1.getStudents();
for (int i = 0; i < students.length; i++)
System.out.print(students[i] + ", ");
/** Print student list and # of students in the Second course */
System.out.println();
System.out.print("Number of students in course2: "
+ course2.getNumberOfStudents() + "\n");
students = course2.getStudents();
for (int i = 0; i < students.length; i++)
System.out.print(students[i] + ", ");
System.out.println();
System.out.println("\n" + " ... Dropping Students from Course 1");
/** Drop a student named S1 from course 1*/
course1.dropStudent("Cindy Shay");
/** Print # of students and Show new class list */
System.out.println("Number of students in course1: "
+ course1.getNumberOfStudents());
students = course1.getStudents();
for (int i = 0; i < course1.getNumberOfStudents(); i++)
System.out.print(students[i] + (i < course1.getNumberOfStudents() - 1 ? ", " : " "));
/** Clear all students from course #2 */
System.out.println("\n\n" + " ... Clearing All Students from Course 2");
course2.clear();
System.out.println("\nNumber of students in course 2: "
+ course2.getNumberOfStudents());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

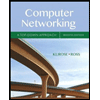
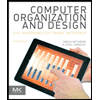
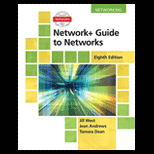
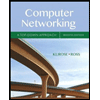
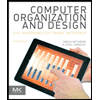
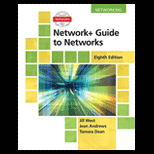
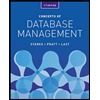
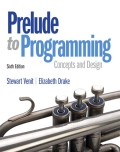
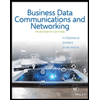