The equation of a line in standard form is ax + by = c , wherein both aand b cannot be zero, and a, b, and c are real numbers. If b≠0, then –a/b is the slope of the line. If a = 0, then it is a horizontal line, and if b = 0, then it is a vertical line. The slope of a vertical line is undefined. Two lines are parallel if they have the same slope or both are vertical lines. Two lines are perpendicular if either one of the lines is horizontal and the other is vertical or the product of their slopes is –1. Design the class lineType to store a line. To store a line, you need to store the values of a (coefficient of x), b (coefficient of y), and c. Your class must contain the following operations: If a line is nonvertical, then determine its slope. Determine if two lines are equal. (Two lines a₁x + b₁y = c₁ and a₂x + b₂y = c₂ are equal if either a₁ = a₂, b₁ = b₂, and c₁ = c₂, or a₁ = ka₂, b₁ = kb₂ and c₁ = kc₂, and for some real number k.) Determine if two lines are parallel. Determine if two lines are perpendicular. If two lines are not parallel, then print the point of intersection. The intersection method should indicate one of three options: The intersection point in the format: (x, y) . A message indicating Both lines are equal. A message indicating the Lines do not inters
The equation of a line in standard form is ax + by = c , wherein both aand b cannot be zero, and a, b, and c are real numbers. If b≠0, then –a/b is the slope of the line. If a = 0, then it is a horizontal line, and if b = 0, then it is a vertical line. The slope of a vertical line is undefined. Two lines are parallel if they have the same slope or both are vertical lines. Two lines are perpendicular if either one of the lines is horizontal and the other is vertical or the product of their slopes is –1. Design the class lineType to store a line. To store a line, you need to store the values of a (coefficient of x), b (coefficient of y), and c. Your class must contain the following operations:
- If a line is nonvertical, then determine its slope.
- Determine if two lines are equal. (Two lines a₁x + b₁y = c₁ and a₂x + b₂y = c₂ are equal if either a₁ = a₂, b₁ = b₂, and c₁ = c₂, or a₁ = ka₂, b₁ = kb₂ and c₁ = kc₂, and for some real number k.)
- Determine if two lines are parallel.
- Determine if two lines are perpendicular.
- If two lines are not parallel, then print the point of intersection. The intersection method should indicate one of three options: The intersection point in the format: (x, y) . A message indicating Both lines are equal. A message indicating the Lines do not intersect.
Add appropriate constructors to initialize variables of lineType. Also, write a program to test your class.

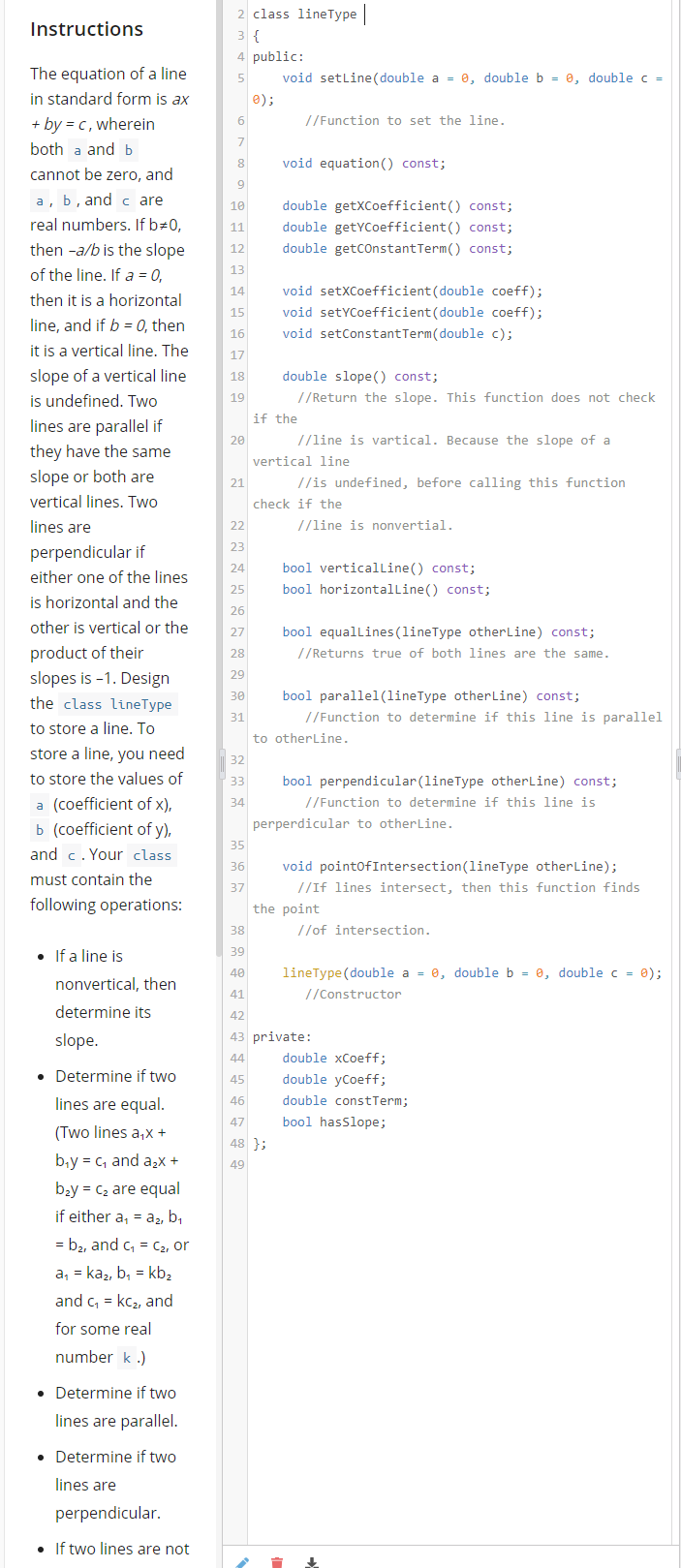

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

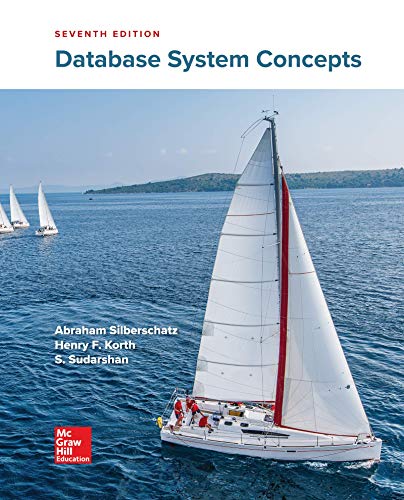
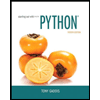
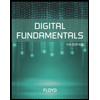
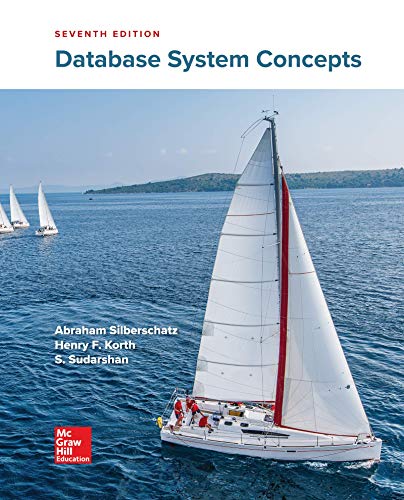
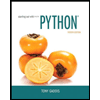
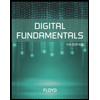
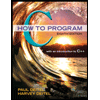
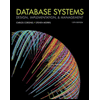
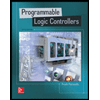