The file text.txt contains a sentence text written in all small letters and does not have any punctuation marks, no numbers, no symbols except for spaces. You are required to write a program which accepts an operation code, and zero to two inputs depending on the operation code. Based on the operation code, you will call three versions of the overlaoded function textProcessing as follows: • Operation 0: The function removes all vowels from the ORIGINAL sentence. The vowels considered are the small letters (a, e, i, o, u y, and w). • Operation 1: The code further asks for one lowercase letter to be input by the user. If the character is not a lower case letter, the code outputs Invalid.The function returns the distance (difference) between the letter first occurence and last occurence in the text. If the letter does not occur in the sentence, it returns 0. • Operation 2: The code asks for two lowercase letters to be input by the user. If the characters are not lower case letters, the code outputs Invalid.The function replaces the original letter by the new letter in the ORIGINAL string. • Any other opcode: the code outputs Invalid. 1/0 Program Input: • A single line that takes in an operation code. • Zero or one or two lower case letters depending on the operation code Program Output: • Depends on the function called and as specified above
The file text.txt contains a sentence text written in all small letters and does not have any punctuation marks, no numbers, no symbols except for spaces. You are required to write a program which accepts an operation code, and zero to two inputs depending on the operation code. Based on the operation code, you will call three versions of the overlaoded function textProcessing as follows: • Operation 0: The function removes all vowels from the ORIGINAL sentence. The vowels considered are the small letters (a, e, i, o, u y, and w). • Operation 1: The code further asks for one lowercase letter to be input by the user. If the character is not a lower case letter, the code outputs Invalid.The function returns the distance (difference) between the letter first occurence and last occurence in the text. If the letter does not occur in the sentence, it returns 0. • Operation 2: The code asks for two lowercase letters to be input by the user. If the characters are not lower case letters, the code outputs Invalid.The function replaces the original letter by the new letter in the ORIGINAL string. • Any other opcode: the code outputs Invalid. 1/0 Program Input: • A single line that takes in an operation code. • Zero or one or two lower case letters depending on the operation code Program Output: • Depends on the function called and as specified above
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.3: Random File Access
Problem 6E
Related questions
Question
c++ language, pleaseeeeeee
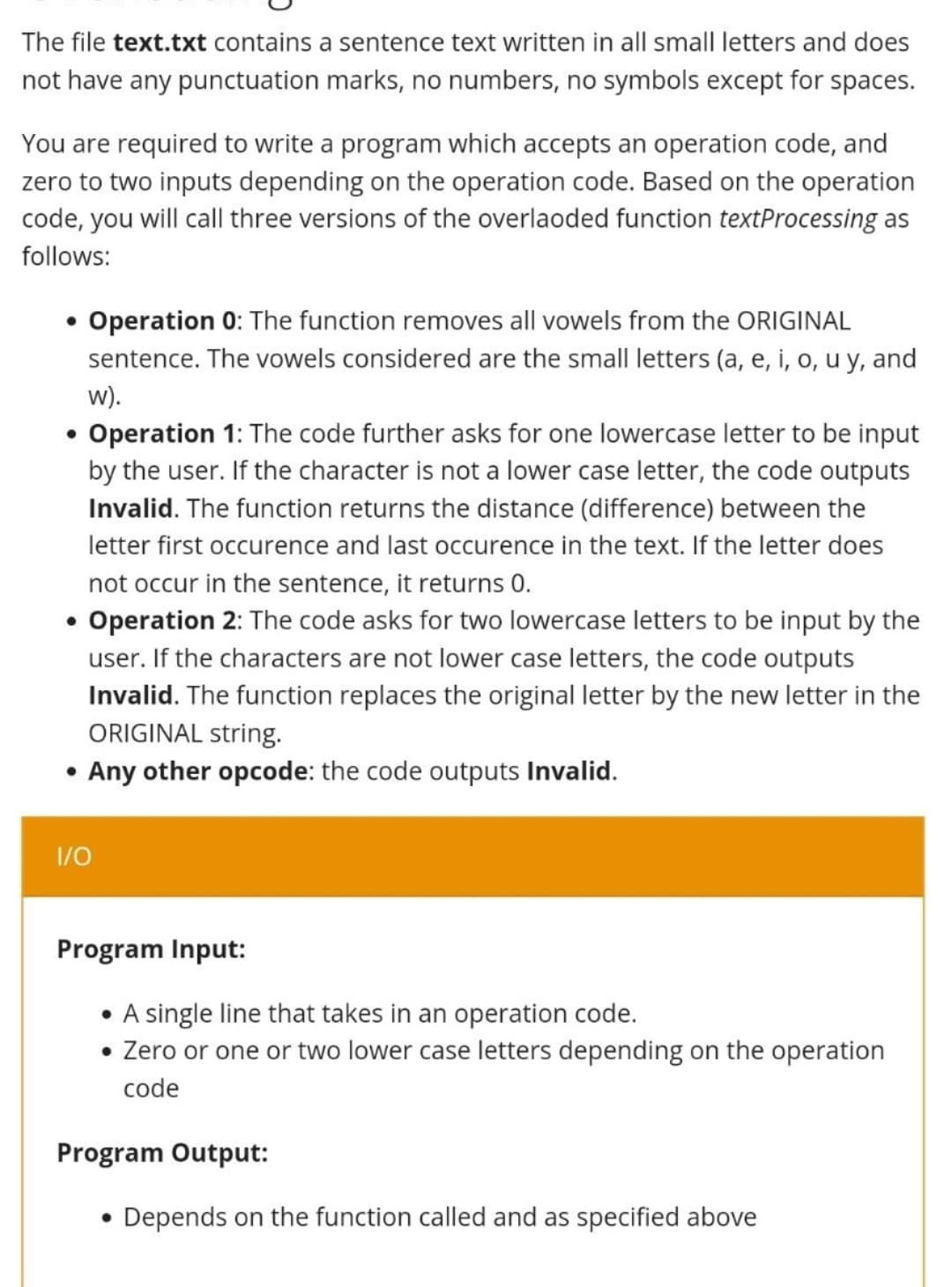
Transcribed Image Text:The file text.txt contains a sentence text written in all small letters and does
not have any punctuation marks, no numbers, no symbols except for spaces.
You are required to write a program which accepts an operation code, and
zero to two inputs depending on the operation code. Based on the operation
code, you will call three versions of the overlaoded function textProcessing as
follows:
●
Operation 0: The function removes all vowels from the ORIGINAL
sentence. The vowels considered are the small letters (a, e, i, o, u y, and
w).
• Operation 1: The code further asks for one lowercase letter to be input
by the user. If the character is not a lower case letter, the code outputs
Invalid.The function returns the distance (difference) between the
letter first occurence and last occurence in the text. If the letter does
not occur in the sentence, it returns 0.
• Operation 2: The code asks for two lowercase letters to be input by the
user. If the characters are not lower case letters, the code outputs
Invalid.The function replaces the original letter by the new letter in the
ORIGINAL string.
• Any other opcode: the code outputs Invalid.
1/0
Program Input:
• A single line that takes in an operation code.
• Zero or one or two lower case letters depending on the operation
code
Program Output:
• Depends on the function called and as specified above
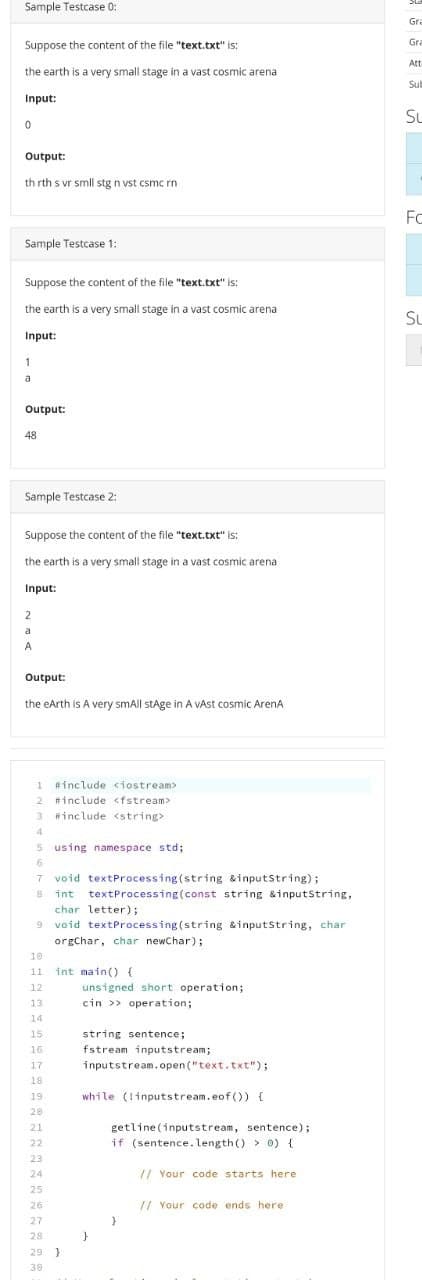
Transcribed Image Text:Sample Testcase 0:
Suppose the content of the file "text.txt" is:
the earth is a very small stage in a vast cosmic arena
Input:
0
Output:
th rth s vr smll stg n vst csmc rn
Sample Testcase 1:
Suppose the content of the file "text.txt" is:
the earth is a very small stage in a vast cosmic arena
Input:
1
a
Output:
48
Sample Testcase 2:
Suppose the content of the file "text.txt" is:
the earth is a very small stage in a vast cosmic arena
Input:
2
a
A
Output:
the earth is A very small stage in A vAst cosmic ArenA
1 #include <iostream>
2 #include <fstream>
3 #include <string>
4
5 using namespace std;
6
7 void textProcessing (string &inputString);
8 int textProcessing (const string &inputString,
char
letter);
9 void textProcessing (string &inputString, char
orgChar, char newChar);
10
11
int main() {
12
unsigned short operation;
13
cin >> operation;
14
14
15
string sentence;
16
16
fstream inputstream;
17
inputstream.open("text.txt");
18
19
while (!inputstream.eof()) {
28
21
22
23
24
25
26
27
28
29 }
30
}
getline (inputstream, sentence);
if (sentence.length() > 0) {
// Your code starts here.
// Your code ends here
}
Gra
Gra
Att
Sul
Su
FC
Su
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
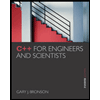
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
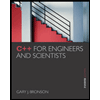
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr