The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a String rather than an Integer. At FIXME in the code, add a try/catch statement to catch java.util.Input MismatchException, and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33. -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34
The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a String rather than an Integer. At FIXME in the code, add a try/catch statement to catch java.util.Input MismatchException, and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33. -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter14: Exception Handling
Section: Chapter Questions
Problem 1PE
Related questions
Question
import java.util.Scanner;
import java.util.InputMismatchException;
public class NameAgeChecker {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String inputName;
int age;
inputName = scnr.next();
while (!inputName.equals("-1")) {
// FIXME: The following line will throw an InputMismatchException.
// Insert a try/catch statement to catch the exception.
age = scnr.nextInt();
System.out.println(inputName + " " + (age + 1));
inputName = scnr.next();
}
}
}

Transcribed Image Text:The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The
program fails and throws an exception if the second input on a line is a String rather than an Integer. At FIXME in the code, add a try/catch
statement to catch java.util.InputMismatch Exception, and output 0 for the age.
Ex: If the input is:
Lee 18
Lua 21
Mary Beth 19.
Stu 33
-1
then the output is:
Lee 19
Lua 22
Mary 0
Stu 34
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
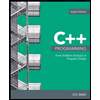
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
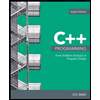
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning