The homework is to re-write the previous program so that the user does not need to enter any freezing point information. Only the salinity of the water. Thefull question in the picture Code in C Previous Program #include #include int main(void) { /* Declare variables. */ double a, f_a, b, f_b, c, f_c; /* Get user input from the keyboard. */ printf("Use ppt for salinity values. \n"); printf("Use degrees F for temperatures. \n"); printf("Enter first salinity and freezing temperature: \n"); scanf("%lf %lf",&a,&f_a); printf("Enter second salinity and freezing temperature: \n"); scanf("%lf %lf",&c,&f_c); printf("Enter new salinity: \n"); scanf("%lf",&b); /* Check that salinity is between first two points. Note the order of a and c is not known, so I am checking for both orders (a>c and ab)&&(b>c)))) { printf("\nThe new salinity is not between the salinity" " of the two provided freezing temperatures."); return(1); } /* Use linear interpolation to compute new freezing temperature. */ f_b = f_a + (b-a)/(c-a)*(f_c - f_a); /* Print new freezing temperature. */ printf("New freezing temperature in degrees F: %0.1f \n",f_b); return 0;/* Exit program. */ } /*–––––––––––––––––––––––––––––––––––––––––––––––––––––––––––*/
The homework is to re-write the previous
Thefull question in the picture
Code in C
Previous Program
#include <stdio.h>
#include <math.h>
int main(void)
{
/* Declare variables. */
double a, f_a, b, f_b, c, f_c;
/* Get user input from the keyboard. */
printf("Use ppt for salinity values. \n");
printf("Use degrees F for temperatures. \n");
printf("Enter first salinity and freezing temperature: \n");
scanf("%lf %lf",&a,&f_a);
printf("Enter second salinity and freezing temperature: \n");
scanf("%lf %lf",&c,&f_c);
printf("Enter new salinity: \n");
scanf("%lf",&b);
/* Check that salinity is between first two points. Note the order of a and c is not known, so I am checking for both orders (a>c and a<c). I will exit the program with a code 1 if this check fails. */
if (!(((a<b)&&(b<c))||((a>b)&&(b>c))))
{
printf("\nThe new salinity is not between the salinity" " of the two provided freezing temperatures.");
return(1);
} /* Use linear interpolation to compute new freezing temperature. */
f_b = f_a + (b-a)/(c-a)*(f_c - f_a);
/* Print new freezing temperature. */
printf("New freezing temperature in degrees F: %0.1f \n",f_b);
return 0;/* Exit program. */
}
/*–––––––––––––––––––––––––––––––––––––––––––––––––––––––––––*/
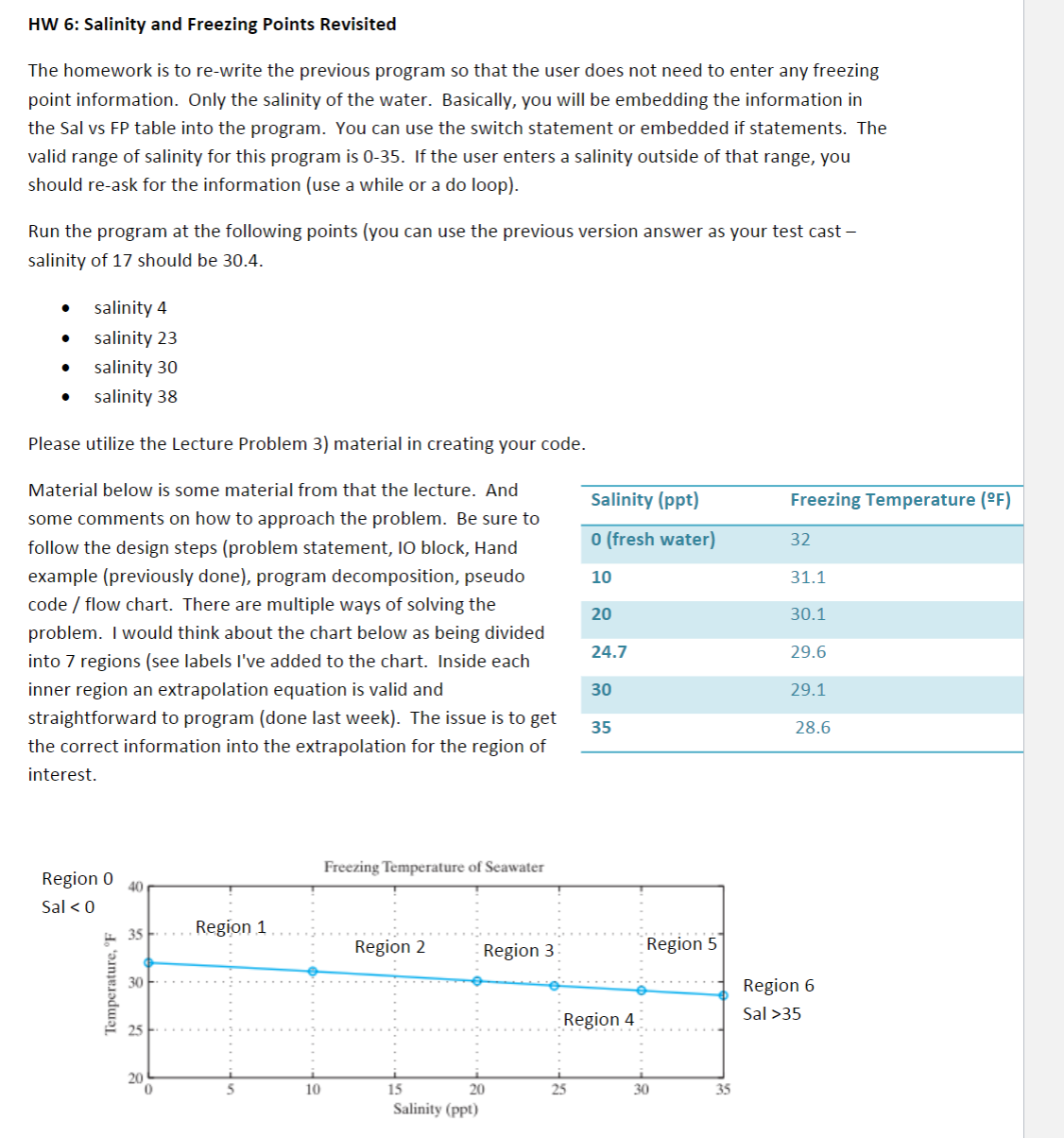

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

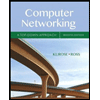
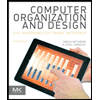
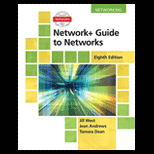
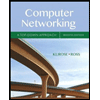
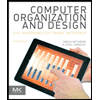
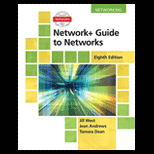
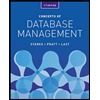
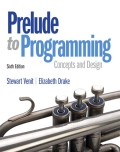
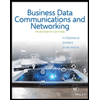