The Monte Carlo method is used in modeling a wide-range of physical systems at the forefront of scientific research today. Monte Carlo simulations are statistical models based on a series of random numbers. Let's consider the problem of estimating Pi by utilizing the Monte Carlo method. Suppose you have a circle inscribed in a square (as in the figure). The experiment simply consists of throwing darts on this figure completely at random (meaning that every point on the dartboard has an equal chance of being hit by the dart). How can we use this experiment to estimate Pi? The answer lies in discovering the relationship between the geometry of the figure and the statistical outcome of throwing the darts. Let's first look at the geometry of the figure. Let's assume the radius of the circle is R, then the Area of the circle = Pi * R^2 and the Area of the square = 4 * R^2. Now if we divide the area of the circle by the area of the square we get Pi / 4. But, how do we estimate Pi by simulation? In the simulation, you keep throwing darts at random onto the dartboard. All of the darts fall within the square, but not all of them fall within the circle. Here's the key. If you throw darts completely at random, this experiment estimates the ratio of the area of the circle to the area of the square, by counting the number of darts within each area. Our study of the geometry tells us this ratio is Pi/4. So, now we can estimate Pi as Pi = 4 x (Number of Darts in Circle) / (Number of Darts in Square) 3 #include 4 #include 5 #include 7 using namespace std; 9 // given the coordinates of a point (x, y) computes if the point is inside or on the circle 10 // centered at origin with radius r. Returns 'true' if it is inside or on the circle, 'false' otherwise. 11 // DO NOT use the sqrt function here. Just compare to r squared. 12 bool isInside(double x, double y, double r) { 13 14 } 15 16 // given s, the size of the side of a square that is centered at the origin, 17 // chooses a random coordinates inside the square, and calls isInside function 18 // to test if the point is inside the circle or not. 19 bool throwDart(int s) { 20 int x, y; 21 // assign x and y to two random integers between -s/2 and s/2 22 23 //Call the isInside function and return its output. //You do not have to cast x & y to doubles, it is done automatically. 24 25 26 } 27 28 int main() { 29 srand(333); int side; // this is the side of the square and is also our resolution. int tries; // this is the number of tries. 30 31 32 33 //Ask the user for the size (integer) of a side of the square 34 35 //Get the users input using cin 36 37 //Ask the user for the number of tries using cout. 38 39 //Get the users input using cin. 40 41 42 int inCount = 0; //counter to track number of throws that fall inside the circle 43 for(int i = 0; i < tries; ++i) { //throw a dart using throwDart method and increment the counter depending on its output. 44 45 46 47 48 49 //Compute and display the estimated value of PI. Make sure you are not using integer division. 50 51 52 return 0;
The Monte Carlo method is used in modeling a wide-range of physical systems at the forefront of scientific research today. Monte Carlo simulations are statistical models based on a series of random numbers. Let's consider the problem of estimating Pi by utilizing the Monte Carlo method. Suppose you have a circle inscribed in a square (as in the figure). The experiment simply consists of throwing darts on this figure completely at random (meaning that every point on the dartboard has an equal chance of being hit by the dart). How can we use this experiment to estimate Pi? The answer lies in discovering the relationship between the geometry of the figure and the statistical outcome of throwing the darts. Let's first look at the geometry of the figure. Let's assume the radius of the circle is R, then the Area of the circle = Pi * R^2 and the Area of the square = 4 * R^2. Now if we divide the area of the circle by the area of the square we get Pi / 4. But, how do we estimate Pi by simulation? In the simulation, you keep throwing darts at random onto the dartboard. All of the darts fall within the square, but not all of them fall within the circle. Here's the key. If you throw darts completely at random, this experiment estimates the ratio of the area of the circle to the area of the square, by counting the number of darts within each area. Our study of the geometry tells us this ratio is Pi/4. So, now we can estimate Pi as Pi = 4 x (Number of Darts in Circle) / (Number of Darts in Square) 3 #include 4 #include 5 #include 7 using namespace std; 9 // given the coordinates of a point (x, y) computes if the point is inside or on the circle 10 // centered at origin with radius r. Returns 'true' if it is inside or on the circle, 'false' otherwise. 11 // DO NOT use the sqrt function here. Just compare to r squared. 12 bool isInside(double x, double y, double r) { 13 14 } 15 16 // given s, the size of the side of a square that is centered at the origin, 17 // chooses a random coordinates inside the square, and calls isInside function 18 // to test if the point is inside the circle or not. 19 bool throwDart(int s) { 20 int x, y; 21 // assign x and y to two random integers between -s/2 and s/2 22 23 //Call the isInside function and return its output. //You do not have to cast x & y to doubles, it is done automatically. 24 25 26 } 27 28 int main() { 29 srand(333); int side; // this is the side of the square and is also our resolution. int tries; // this is the number of tries. 30 31 32 33 //Ask the user for the size (integer) of a side of the square 34 35 //Get the users input using cin 36 37 //Ask the user for the number of tries using cout. 38 39 //Get the users input using cin. 40 41 42 int inCount = 0; //counter to track number of throws that fall inside the circle 43 for(int i = 0; i < tries; ++i) { //throw a dart using throwDart method and increment the counter depending on its output. 44 45 46 47 48 49 //Compute and display the estimated value of PI. Make sure you are not using integer division. 50 51 52 return 0;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
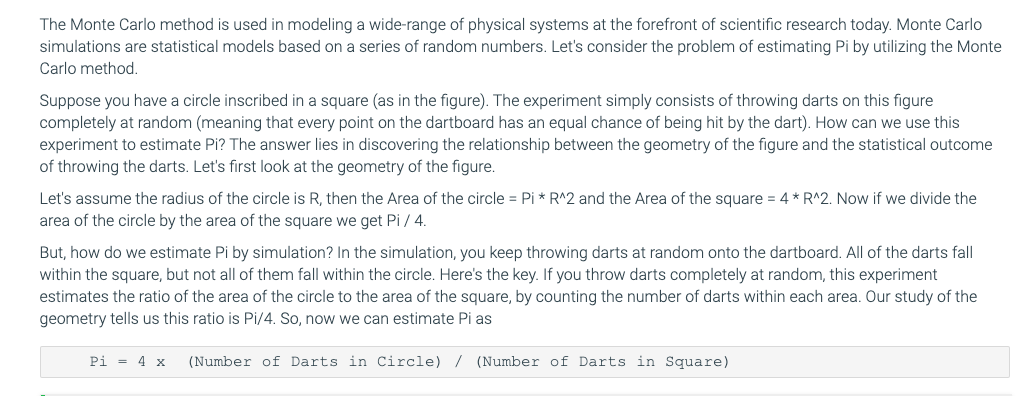
Transcribed Image Text:The Monte Carlo method is used in modeling a wide-range of physical systems at the forefront of scientific research today. Monte Carlo
simulations are statistical models based on a series of random numbers. Let's consider the problem of estimating Pi by utilizing the Monte
Carlo method.
Suppose you have a circle inscribed in a square (as in the figure). The experiment simply consists of throwing darts on this figure
completely at random (meaning that every point on the dartboard has an equal chance of being hit by the dart). How can we use this
experiment to estimate Pi? The answer lies in discovering the relationship between the geometry of the figure and the statistical outcome
of throwing the darts. Let's first look at the geometry of the figure.
Let's assume the radius of the circle is R, then the Area of the circle = Pi * R^2 and the Area of the square = 4 * R^2. Now if we divide the
area of the circle by the area of the square we get Pi / 4.
But, how do we estimate Pi by simulation? In the simulation, you keep throwing darts at random onto the dartboard. All of the darts fall
within the square, but not all of them fall within the circle. Here's the key. If you throw darts completely at random, this experiment
estimates the ratio of the area of the circle to the area of the square, by counting the number of darts within each area. Our study of the
geometry tells us this ratio is Pi/4. So, now we can estimate Pi as
Pi = 4 x
(Number of Darts in Circle) / (Number of Darts in Square)
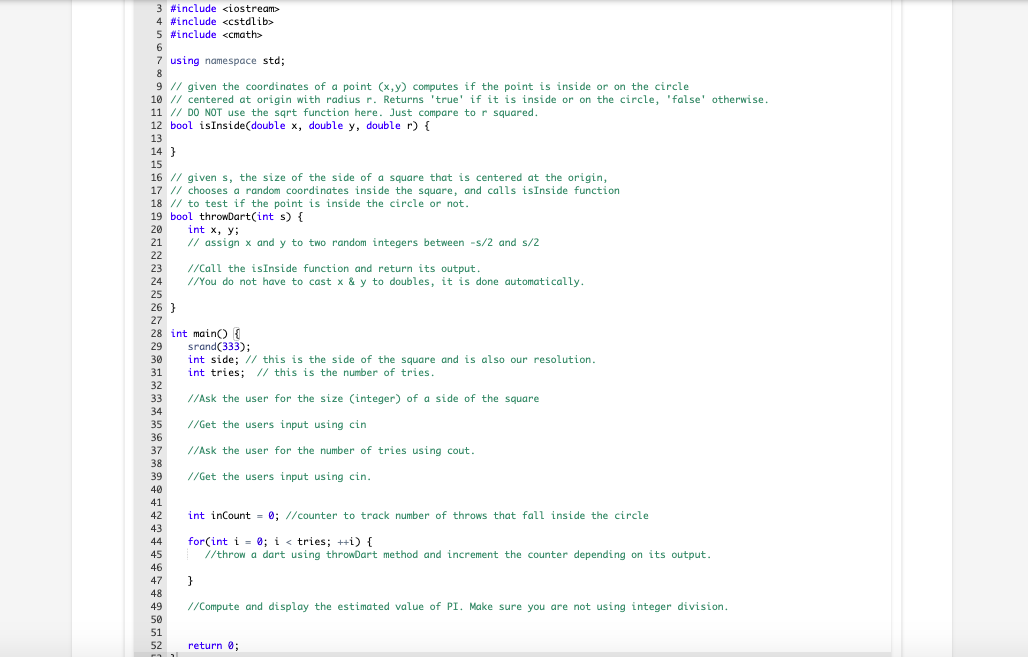
Transcribed Image Text:3 #include <iostream>
4 #include <cstdlib>
5 #include <cmath>
7 using namespace std;
9 // given the coordinates of a point (x, y) computes if the point is inside or on the circle
10 // centered at origin with radius r. Returns 'true' if it is inside or on the circle, 'false' otherwise.
11 // DO NOT use the sqrt function here. Just compare to r squared.
12 bool isInside(double x, double y, double r) {
13
14 }
15
16 // given s, the size of the side of a square that is centered at the origin,
17 // chooses a random coordinates inside the square, and calls isInside function
18 // to test if the point is inside the circle or not.
19 bool throwDart(int s) {
20
int x, y;
21
// assign x and y to two random integers between -s/2 and s/2
22
23
//Call the isInside function and return its output.
//You do not have to cast x & y to doubles, it is done automatically.
24
25
26 }
27
28 int main() {
29
srand(333);
int side; // this is the side of the square and is also our resolution.
int tries; // this is the number of tries.
30
31
32
33
//Ask the user for the size (integer) of a side of the square
34
35
//Get the users input using cin
36
37
//Ask the user for the number of tries using cout.
38
39
//Get the users input using cin.
40
41
42
int inCount = 0; //counter to track number of throws that fall inside the circle
43
for(int i = 0; i < tries; ++i) {
//throw a dart using throwDart method and increment the counter depending on its output.
44
45
46
47
48
49
//Compute and display the estimated value of PI. Make sure you are not using integer division.
50
51
52
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
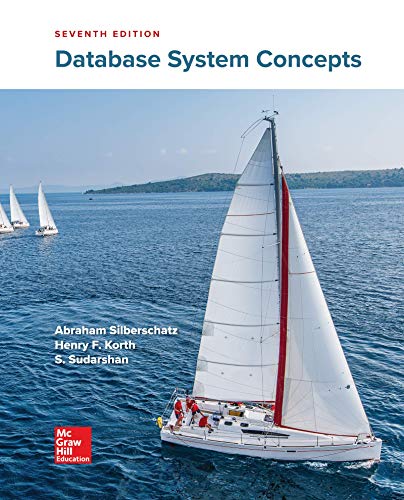
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
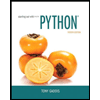
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
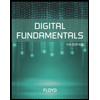
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
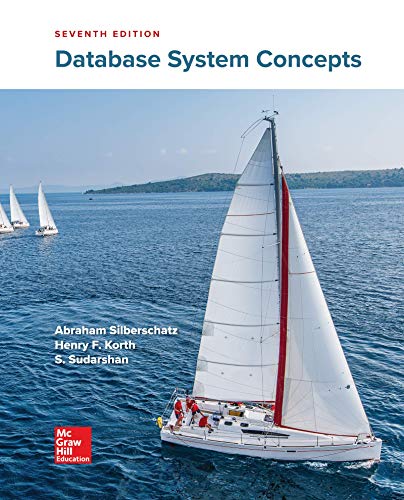
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
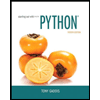
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
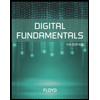
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
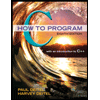
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
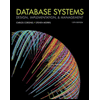
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
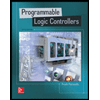
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education