The office hours for Professor X in Spring 2021 are as follows: Monday 3:00pm - 5:00pm Thursday 3:30pm - 5:00pm Friday 3:00pm - 4:30pm Note that all office hours are in the late afternoon. a program that will take the day of the week and a time (XX:XXam/pm) as inputs, and will output whether the professor is available during that time on that day. Start NetBeans. new project called Lab4 with a main class called YournameLab4 with your name. Declare a String variable to represent the day of the week. Prompt the user for the day of the week and store it in the variable from step 3. The result should look like this: Enter the day of the week: Monday Declare a String variable to represent the time of day. Prompt the user for the time of day in the format XX:XXam/pm (e.g., 10:45am, 3:30pm), and store it in the variable from step 5. The result should look like this: Enter a time (XX:XXam/pm): 4:15pm Declare two int variables to represent the hour and minute, and a String variable to represent a value of "am" or "pm". Use the String class length, indexOf, and substring methods to process time to extract the hour, minute, and am/pm information. Store the results in the three variables from step 7. You will need to use the Integer.parseInt method to convert a String to an int. int number = Integer.parseInt("42"); // converts "42" to 42 Use if-else statements to display the entered day of the week in the following format: Day of the week: Monday Use the String class equalsIgnoreCase method to identify the entered input in different format (e.g. "Mon", "mon", "MON", "Monday", "monday", "MONDAY"). Use the logical OR operator || to build the Boolean expressions. For each day when the professor does not have office hour (Tuesday, Wednesday, Saturday, Sunday), add an additional statement that displays "Not Available". For days, when the professor has office hours (Monday, Thursday, and Friday), write nested if-else statements within the respective if-else statements described in step 9 to check whether the professor is available at that particular time. There are 3 cases to consider. If the time entered is AM, display "Not Available" since the professor's office hours are in the late afternoon. If the time entered is PM, and it's within the office hour time, then display "Available" If the time entered is PM, but it's not within the office hour time, then display "Not Available". Use the String class equals method to check whether the entered time is "am" or "pm". To check the whether the entered time is during the office hours, use Java comparison and logical operators. The output from your program should look like the following Sample 1 Enter the day of the week: MON Enter a time (XX:XXam/pm): 10:30am Day of the week: MON Not available Sample 2 Enter the day of the week: Monday Enter a time (XX:XXam/pm): 3:45pm Day of the week: Monday Available Sample 3 Enter the day of the week: Friday Enter a time (XX:XXam/pm): 04:45pm Day of the week: Friday Not Available Sample 4 Enter the day of the week: thu Enter a time (XX:XXam/pm): 2:00pm Day of the week: thu Not Available Sample 5 Enter the day of the week: wed Enter a time (XX:XXam/pm): 9:00am Day of the week: wed Not Available
The office hours for Professor X in Spring 2021 are as follows: Monday 3:00pm - 5:00pm Thursday 3:30pm - 5:00pm Friday 3:00pm - 4:30pm Note that all office hours are in the late afternoon. a program that will take the day of the week and a time (XX:XXam/pm) as inputs, and will output whether the professor is available during that time on that day. Start NetBeans. new project called Lab4 with a main class called YournameLab4 with your name. Declare a String variable to represent the day of the week. Prompt the user for the day of the week and store it in the variable from step 3. The result should look like this: Enter the day of the week: Monday Declare a String variable to represent the time of day. Prompt the user for the time of day in the format XX:XXam/pm (e.g., 10:45am, 3:30pm), and store it in the variable from step 5. The result should look like this: Enter a time (XX:XXam/pm): 4:15pm Declare two int variables to represent the hour and minute, and a String variable to represent a value of "am" or "pm". Use the String class length, indexOf, and substring methods to process time to extract the hour, minute, and am/pm information. Store the results in the three variables from step 7. You will need to use the Integer.parseInt method to convert a String to an int. int number = Integer.parseInt("42"); // converts "42" to 42 Use if-else statements to display the entered day of the week in the following format: Day of the week: Monday Use the String class equalsIgnoreCase method to identify the entered input in different format (e.g. "Mon", "mon", "MON", "Monday", "monday", "MONDAY"). Use the logical OR operator || to build the Boolean expressions. For each day when the professor does not have office hour (Tuesday, Wednesday, Saturday, Sunday), add an additional statement that displays "Not Available". For days, when the professor has office hours (Monday, Thursday, and Friday), write nested if-else statements within the respective if-else statements described in step 9 to check whether the professor is available at that particular time. There are 3 cases to consider. If the time entered is AM, display "Not Available" since the professor's office hours are in the late afternoon. If the time entered is PM, and it's within the office hour time, then display "Available" If the time entered is PM, but it's not within the office hour time, then display "Not Available". Use the String class equals method to check whether the entered time is "am" or "pm". To check the whether the entered time is during the office hours, use Java comparison and logical operators. The output from your program should look like the following Sample 1 Enter the day of the week: MON Enter a time (XX:XXam/pm): 10:30am Day of the week: MON Not available Sample 2 Enter the day of the week: Monday Enter a time (XX:XXam/pm): 3:45pm Day of the week: Monday Available Sample 3 Enter the day of the week: Friday Enter a time (XX:XXam/pm): 04:45pm Day of the week: Friday Not Available Sample 4 Enter the day of the week: thu Enter a time (XX:XXam/pm): 2:00pm Day of the week: thu Not Available Sample 5 Enter the day of the week: wed Enter a time (XX:XXam/pm): 9:00am Day of the week: wed Not Available
Chapter5: Making Decisions
Section: Chapter Questions
Problem 4PE
Related questions
Question
The office hours for Professor X in Spring 2021 are as follows:
- Monday 3:00pm - 5:00pm
- Thursday 3:30pm - 5:00pm
- Friday 3:00pm - 4:30pm
Note that all office hours are in the late afternoon. a
- Start NetBeans.
- new project called Lab4 with a main class called YournameLab4 with your name.
- Declare a String variable to represent the day of the week.
- Prompt the user for the day of the week and store it in the variable from step 3. The result should look like this:
- Enter the day of the week: Monday
- Declare a String variable to represent the time of day.
- Prompt the user for the time of day in the format XX:XXam/pm (e.g., 10:45am, 3:30pm), and store it in the variable from step 5. The result should look like this:
- Enter a time (XX:XXam/pm): 4:15pm
- Declare two int variables to represent the hour and minute, and a String variable to represent a value of "am" or "pm".
- Use the String class length, indexOf, and substring methods to process time to extract the hour, minute, and am/pm information. Store the results in the three variables from step 7. You will need to use the Integer.parseInt method to convert a String to an int.
- int number = Integer.parseInt("42"); // converts "42" to 42
- Use if-else statements to display the entered day of the week in the following format:
- Day of the week: Monday
- Use the String class equalsIgnoreCase method to identify the entered input in different format (e.g. "Mon", "mon", "MON", "Monday", "monday", "MONDAY"). Use the logical OR operator || to build the Boolean expressions.
- For each day when the professor does not have office hour (Tuesday, Wednesday, Saturday, Sunday), add an additional statement that displays "Not Available".
- For days, when the professor has office hours (Monday, Thursday, and Friday), write nested if-else statements within the respective if-else statements described in step 9 to check whether the professor is available at that particular time. There are 3 cases to consider.
- If the time entered is AM, display "Not Available" since the professor's office hours are in the late afternoon.
- If the time entered is PM, and it's within the office hour time, then display "Available"
- If the time entered is PM, but it's not within the office hour time, then display "Not Available".
- Use the String class equals method to check whether the entered time is "am" or "pm". To check the whether the entered time is during the office hours, use Java comparison and logical operators.
- The output from your program should look like the following
Sample 1
- Enter the day of the week:
MON
Enter a time (XX:XXam/pm):
10:30am
Day of the week: MON
Not available
Sample 2
- Enter the day of the week:
Monday
Enter a time (XX:XXam/pm):
3:45pm
Day of the week: Monday
Available
Sample 3
- Enter the day of the week:
Friday
Enter a time (XX:XXam/pm):
04:45pm
Day of the week: Friday
Not Available
Sample 4
- Enter the day of the week:
thu
Enter a time (XX:XXam/pm):
2:00pm
Day of the week: thu
Not Available
Sample 5
- Enter the day of the week:
wed
Enter a time (XX:XXam/pm):
9:00am
Day of the week: wed
Not Available
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
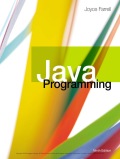
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
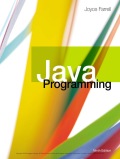
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT