The perimeter P of the rectangle bounded by the two sides L and Wis P = 2(L+W) Write a piece of code that implements this equation. In other words, translate the above equation into code. This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and w. Python performs calculations on arrays element-by-element. #Taking input for the lengths and widths as arrays lengths [] widths = [] num_rectangles = int(input("Enter the number of rectangles: ")) for i in range(num_rectangles): length float (input (f"Enter the length of rectangle {i + 1}: ")) width = float (input (f"Enter the width of rectangle (i + 1}: ")) lengths.append(length) widths.append(width) #Calculating the perimeters for each rectangle perimeters = [2 * (L + W) for L, W in zip(lengths, widths)] #Printing the results for i, P in enumerate (perimeters): print (f"The perimeter of rectangle {i + 1} is:", P) # Let's see the perimeter: print ("perimeter: ", P) This calculation gave us a new array P. Each element is twice the sum of the corresponding elements in L and W. Now plot a histogram of P, using code similar to the one you used for L and W: Note: Do not redefine P. If your code below contains any line that starts with P =, you are doing it wrong. P was defined in the previous code cell and should be left untouched. #YOUR CODE HERE raise Not ImplementedError()
The perimeter P of the rectangle bounded by the two sides L and Wis P = 2(L+W) Write a piece of code that implements this equation. In other words, translate the above equation into code. This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and w. Python performs calculations on arrays element-by-element. #Taking input for the lengths and widths as arrays lengths [] widths = [] num_rectangles = int(input("Enter the number of rectangles: ")) for i in range(num_rectangles): length float (input (f"Enter the length of rectangle {i + 1}: ")) width = float (input (f"Enter the width of rectangle (i + 1}: ")) lengths.append(length) widths.append(width) #Calculating the perimeters for each rectangle perimeters = [2 * (L + W) for L, W in zip(lengths, widths)] #Printing the results for i, P in enumerate (perimeters): print (f"The perimeter of rectangle {i + 1} is:", P) # Let's see the perimeter: print ("perimeter: ", P) This calculation gave us a new array P. Each element is twice the sum of the corresponding elements in L and W. Now plot a histogram of P, using code similar to the one you used for L and W: Note: Do not redefine P. If your code below contains any line that starts with P =, you are doing it wrong. P was defined in the previous code cell and should be left untouched. #YOUR CODE HERE raise Not ImplementedError()
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.3: Declaring And Processing Two-dimensional Arrays
Problem 7E: (Electrical eng.) a. An engineer has constructed a two-dimensional array of real numbers with three...
Related questions
Question
![The perimeter P of the rectangle bounded by the two sides L and Wis
P=2(L + W)
Write a piece of code that implements this equation. In other words, translate the above equation into code.
This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and w. Python
performs calculations on arrays element-by-element.
▼ #Taking input for the lengths and widths as arrays
lengths
[]
widths []
num_rectangles int(input ("Enter the number of rectangles: "))
for i in range(num_rectangles):
length
width =
float (input (f"Enter the length of rectangle {i + 1}: "))
float (input(f"Enter the width of rectangle {i + 1}: "))
lengths.append(length)
widths.append(width)
=
# Calculating the perimeters for each rectangle
perimeters [2* (LW) for L, W in zip(lengths, widths)]
#Printing the results
for i, P in enumerate (perimeters):
print (f"The perimeter of rectangle {i + 1} is:", P)
#Let's see the perimeter:
print ("perimeter: ", P)
This calculation gave us a new array P. Each element is twice the sum of the corresponding elements in L and W.
Now plot a histogram of P, using code similar to the one you used for L and W:
Note: Do not redefine P. If your code below contains any line that starts with P =, you are doing it wrong. P was defined in the previous code cell
and should be left untouched.
#YOUR CODE HERE
raise Not ImplementedError()
Your histogram should look like another normal distribution, centered at Po
=
80 mm. (Why 80 mm? Because 2(25 + 15) = 80.)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7f470a0-93f2-43bf-9ed3-2f125d19f075%2Fb08af792-9304-4513-9eba-67db56577bb1%2Fm87928_processed.png&w=3840&q=75)
Transcribed Image Text:The perimeter P of the rectangle bounded by the two sides L and Wis
P=2(L + W)
Write a piece of code that implements this equation. In other words, translate the above equation into code.
This should be a simple one-liner! Do not use the np.random.normal() function. You must use the previously defined arrays L and w. Python
performs calculations on arrays element-by-element.
▼ #Taking input for the lengths and widths as arrays
lengths
[]
widths []
num_rectangles int(input ("Enter the number of rectangles: "))
for i in range(num_rectangles):
length
width =
float (input (f"Enter the length of rectangle {i + 1}: "))
float (input(f"Enter the width of rectangle {i + 1}: "))
lengths.append(length)
widths.append(width)
=
# Calculating the perimeters for each rectangle
perimeters [2* (LW) for L, W in zip(lengths, widths)]
#Printing the results
for i, P in enumerate (perimeters):
print (f"The perimeter of rectangle {i + 1} is:", P)
#Let's see the perimeter:
print ("perimeter: ", P)
This calculation gave us a new array P. Each element is twice the sum of the corresponding elements in L and W.
Now plot a histogram of P, using code similar to the one you used for L and W:
Note: Do not redefine P. If your code below contains any line that starts with P =, you are doing it wrong. P was defined in the previous code cell
and should be left untouched.
#YOUR CODE HERE
raise Not ImplementedError()
Your histogram should look like another normal distribution, centered at Po
=
80 mm. (Why 80 mm? Because 2(25 + 15) = 80.)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
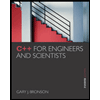
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
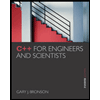
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage