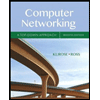
Need help with part B the decode method AND the getChoice method using try/catch
The program with the main method should be called ‘LetterCode.java’ The program with the class for performing the operations should be called LetterCodeLogic.java. The class should have two static methods: Encode and Decode (both of which receive a string parameter).
Part A: The part A program will be the Encode function
Part B: The Part B program will be the Decode function.
This is my code so far for LetterCode.java:
package lettercode;
import business.LetterCodeLogic;
import java.util.Scanner;
/**
*
* @author Sean Jeffries
*/
public class LetterCode {
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
int choice;
String msg="", result="";
System.out.println("welcome to the Letter Code Program!");
choice = getChoice();
while (choice != 0) {
switch (choice) {
case 1: //encode
System.out.print("Enter your message to be encoded: ");
msg = sc.nextLine();
result = LetterCodeLogic.Encode(msg);
System.out.println("Your encoded message is:");
System.out.println(result);
break;
case 2: //decode
System.out.print("Enter your numbers to be decoded (seperate with commas): ");
msg = sc.nextLine();
result = LetterCodeLogic.Decode(msg);
System.out.println("Your decoded message is: ");
System.out.println(result);
break;
default:
System.out.println("Unknown operation\n");
break;
}//end of switch
choice = getChoice();
}//end of while
}//end of main
public static int getChoice() {
//must be robust with data validation and error trapping
int c;
System.out.print("Choice? 1=Encode, 2=Decode, 0=Quit: ");
c = Integer.parseInt(sc.nextLine());
return c;
}
}
And this is my code so far for the LetterCodeLogic.java
package business;
/**
*
* @author Sean Jeffries
*/
public class LetterCodeLogic {
public static String Encode(String msg) {
String m = msg.toUpperCase();
String result="";
//convert characters to numbers using the ASCII coding scheme
// A=65, B=66, C=67, etc. (space = 32)
//also use the fact that characters and integers are equivalent
//based on this scheme...
int x;
char c; //single character (not string) - use apostrophe
//pull characters froim the input string m
for(int i=0; i < m.length(); i++) {
c = m.charAt(i); //take string value at position i and turn it into a char
x = c; //creates value x from char using ASCII scheme
if (x == 32) {
x = 0; //convert value of space to zero
} else {
x -= 64; //adjust x to 1,2,3 etc.
if (x < 1 || x > 26) {
x = 99;
}
}
//character at position i of string is now a value in x
//of 0 for space, 1-26 for letter, or 99 for unkown character
result += String.valueOf(x) + " ";
}
return result;
}
public static String Decode(String msg) {
String result = "";
//input string looks like (ex): "1,2,3,4,5,6"
//need to extract numbers 1 2 3 4 5 6
//use the split method of string
String[] nums = msg.split(",");
int x=0;
char c;
for (int i = 0; i < nums.length; i++) {
//first step: convert value in current position i of array to integer...
//process here is reverse of encode: take number returned by conversion
//change it to space (ASCII 32) or capital letter (ASCII 65 and following
x = Integer.parseInt(nums[i]);
c = (char)x;
if (c == 0) {
c = (char)32;
} else {
c -= (char)64;
if (c < 65 || c > 90) {
c = (char)35;
}
}
result += String.valueOf(x) + " ";
}
return result;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- basic java please Write a void method named emptyBox()that accepts two parameters: the first parameter should be the height of a box to be drawn, and the second parameter will be the width. The method should then draw an empty box of the correct size. For example, the call emptyBox(8, 5) should produce the following output: ***** * * * * * * * * * * * * ***** The width of the box is 5. The middle lines have 3 spaces in the middle. The height of the box is 8. The middle columns have 6 spacesarrow_forwardin Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forwardDon't use AI.arrow_forward
- Student name: CIS 232 Introduction to Programming Homework Assignment 10 Due Date: 11/23/2020 Instructor: Dr. Lomako Problem Statement: Write a Java program with the main method and a multiplication method (must be created, not the library method). Create “HW10_lastname.java” program that: Prints a program title Creates two n x n square matrices A and B and initializes them with random values Displays the matrices A and B Calls the multiplication method that multiplies two square matrices and returns a matrix AB that is the result of the multiplication. Prints AB matrixarrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardCSCI 140/L Java Project: Menu-Driven System Part A Write a menu-driven program that will give the user the three choices: 1) Wage calculator, 2) Coupon Calculator, and 3) Exit. Class Name: PartA Wage Calculator: For the wage calculator, prompt for the name and hourly pay rate of an employee. Here the hourly pay rate is a floating-point number, such as $9.25. Then ask how many hours the employee worked in the past week. Be sure to accept fractional hours. Compute the pay. Any overtime work (over 40 hours per week) is paid at 150 percent of the regular wage (1.5 the hourly pay rate). Print the employee's name, regular hours worked, regular hours pay, overtime hours worked (do not show overtime hours, if there are none), overtime hours pay (do not show overtime pay if there is none), and total pay. [Do not prompt for overtime hours] Coupon Calculator: For the coupon calculator, the total coupon amount is calculated based on the type of items purchased. Ask for the shopper's total purchase…arrow_forward
- 18. Gas Prices In the student sample program files for this chapter, you will find a text file named Gas Prices.txt. The file contains the weekly average prices for a gallon of gas in the United States, beginning on April 5, 1993, and ending on August 26, 2013. Here is an example of the first few lines of the file's contents: 04-05-1993:1.068 04-12-1993:1.079 04-19-1993:1.079 04-26-1993:1.086 05-03-1993:1.086 and so on... Each line in the file contains the average price for a gallon of gas on a specific date. Each line is formatted in the following way: MM-DD-YYYY: Pricearrow_forwardT/F The body of a method may be emptyarrow_forwardConstant Assignment • The compiler will issue an error if you try to change the value of a constant • In Java, we use the final modifier to declare a constant final int x= 69; Add x= x+10 What is the result?arrow_forward
- PHYTONarrow_forwardThis code should be working at repl.itarrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
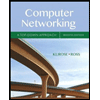
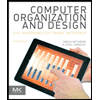
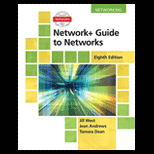
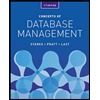
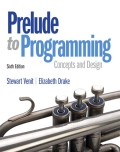
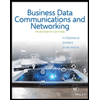