There are two logic errors in setting up the global constants. Locate and fix those errors.
There are two logic errors in setting up the global constants. Locate and fix those errors.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This the original js code unmodified and the complete question.
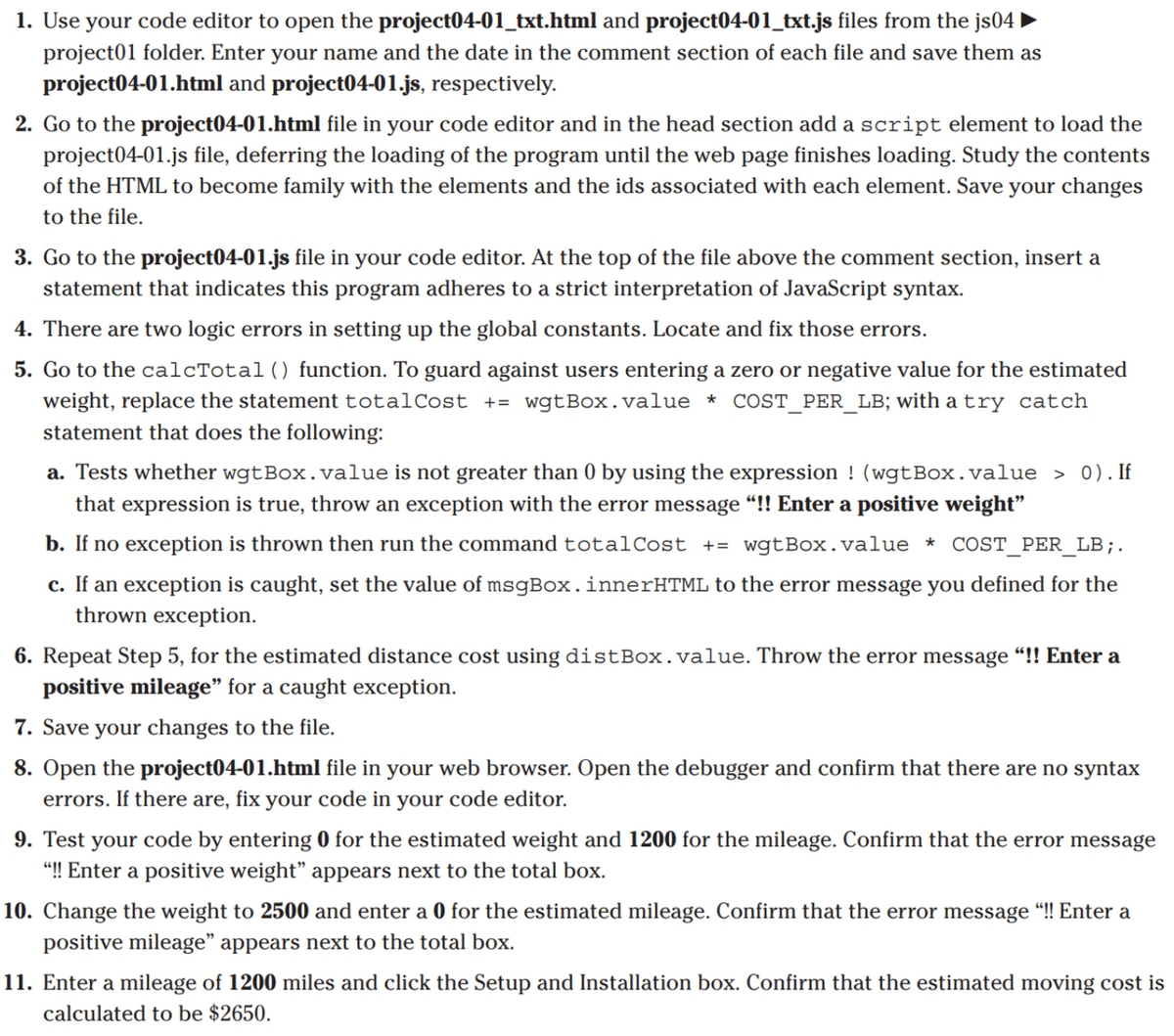
Transcribed Image Text:1. Use your code editor to open the project04-01_txt.html and project04-01_txt.js files from the js04 |
project01 folder. Enter your name and the date in the comment section of each file and save them as
project04-01.html and project04-01.js, respectively.
2. Go to the project04-01.html file in your code editor and in the head section add a script element to load the
project04-01.js file, deferring the loading of the program until the web page finishes loading. Study the contents
of the HTML to become family with the elements and the ids associated with each element. Save your changes
to the file.
3. Go to the project04-01.js file in your code editor. At the top of the file above the comment section, insert a
statement that indicates this program adheres to a strict interpretation of JavaScript syntax.
4. There are two logic errors in setting up the global constants. Locate and fix those errors.
5. Go to the calcTotal() function. To guard against users entering a zero or negative value for the estimated
weight, replace the statement totalCost += wgtBox.value * COST_PER_LB; with a try catch
statement that does the following:
a. Tests whether wgtBox.value is not greater than 0 by using the expression ! (wgtBox.value > 0). If
that expression is true, throw an exception with the error message “!! Enter a positive weight"
b. If no exception is thrown then run the command totalCost += wgtBox.value * COST_PER_LB;.
c. If an exception is caught, set the value of msgBox.innerHTML to the error message you defined for the
thrown exception.
6. Repeat Step 5, for the estimated distance cost using distBox. value. Throw the error message “!! Enter a
positive mileage" for a caught exception.
7. Save your changes to the file.
8. Open the project04-01.html file in your web browser. Open the debugger and confirm that there are no syntax
errors. If there are, fix your code in your code editor.
9. Test your code by entering 0 for the estimated weight and 1200 for the mileage. Confirm that the error message
"! Enter a positive weight" appears next to the total box.
10. Change the weight to 2500 and enter a 0 for the estimated mileage. Confirm that the error message “! Enter a
positive mileage" appears next to the total box.
11. Enter a mileage of 1200 miles and click the Setup and Installation box. Confirm that the estimated moving cost is
calculated to be $2650.
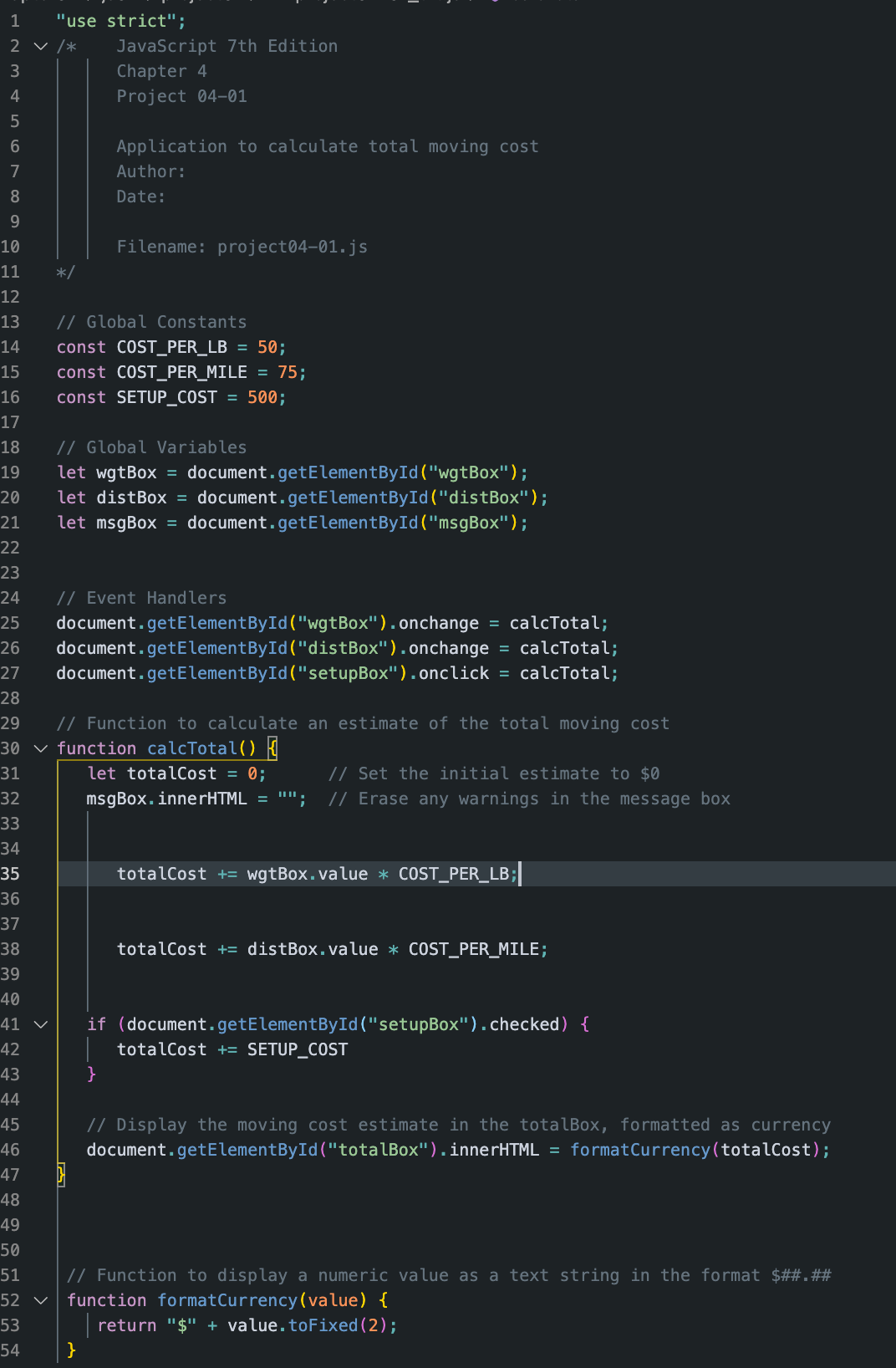
Transcribed Image Text:1
"use strict";
2 v /*
JavaScript 7th Edition
3
Chapter 4
4
Project 04-01
Application to calculate total moving cost
7
Author:
8
Date:
9
10
Filename: project04-01.js
11
*/
12
13
// Global Constants
14
const COST_PER_LB
= 50;
15
const COST_PER_MILE = 75;
16
const SETUP_COST = 500;
17
// Global Variables
let wgtBox = document.getElementById("wgtBox");
let distBox = document.getElementById("distBox");
let msgBox = document.getElementById("msgBox");
18
19
20
21
22
23
// Event Handlers
document.getElementById("wgtBox").onchange = calcTotal;
document.getElementById("distBox").onchange = calcTotal;
document.getElementById("setupBox").onclick = calcTotal;
24
25
26
27
28
// Function to calculate an estimate of the total moving cost
30 v function calcTotal()
29
31
let totalCost = 0;
// Set the initial estimate to $0
32
msgBox.innerHTML =
// Erase any warnings in the message box
33
34
35
totalCost += wgtBox.value * COST_PER_LB;
36
37
38
totalCost += distBox.value * COST_PER_MILE;
39
40
if (document.getElementById("setupBox").checked) {
|totalCost += SETUP_COST
41 v
42
43
}
44
45
// Display the moving cost estimate in the totalBox, formatted as currency
46
document.getElementById("totalBox").innerHTML = formatCurrency(totalCost);
47
48
49
50
// Function to display a numeric value as a text string in the format $##.##
function formatCurrency(value) {
51
52 v
53
return "$" + value.toFixed(2);
54
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
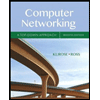
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
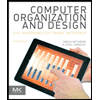
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
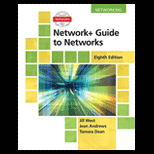
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
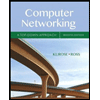
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
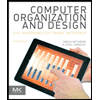
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
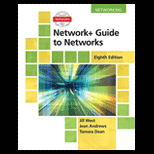
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
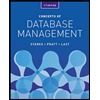
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
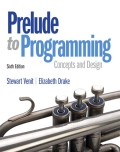
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
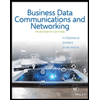
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY