There is a problem with my program. I need the JOptionpane to pop up when I run the program and that I am unable to enter bets, the only option it gives me is to input y or no to play again or end the program. Java Language: import javax.swing.JOptionPane; import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.util.*; import java.io.*; import java.util.Random; public class Craps { double balance, startBalance, bet; int wins, loss, games; boolean win; boolean eligibletoPlay; public enum Status { CONTINUE, WON, LOST }; final static int SNAKE_EYES = 2; final static int TREY = 3; final static int SEVEN = 7; final static int ELEVEN = 11; final static int BOX_CARS = 12; final static double MIN_BALANCE = 200.00; final static double MIN_BET = 1.00; Scanner input; public static void main () { Craps game = new Craps(); game.NewGame(); } public void NewGame() { String answer; input = new Scanner(System.in); while (true) { System.out.print("Do you wish to play a new game? "); answer = input.next(); if (answer.toLowerCase().charAt(0) == 'y') { play(); } else if (answer.toLowerCase().charAt(0) == 'n') { printreport(); System.exit(5); } else System.out.println("Please type in either 'y' to play again or 'n' to quit"); } } public boolean play () { int point = 0; int sumOfDice = roll(); Status gameStatus; switch (sumOfDice) { case SEVEN: case ELEVEN: gameStatus = Status.WON; System.out.printf("Player rolls: %d\n", sumOfDice); break; case SNAKE_EYES: case TREY: case BOX_CARS: gameStatus = Status.LOST; System.out.printf("Player rolls: %d\n", sumOfDice); break; default: gameStatus = Status.CONTINUE; point = sumOfDice; System.out.printf("Player rolls: %d\n", point); } while ( gameStatus == Status.CONTINUE ) { sumOfDice = roll(); System.out.printf("Player rolls: %d\n", sumOfDice); if (sumOfDice == point) { gameStatus = Status.WON; } else if (sumOfDice == SEVEN) { gameStatus = Status.LOST; } } if ( gameStatus == Status.WON ) { System.out.printf("Congratulations, You win\n\n", sumOfDice); } else System.out.printf("Sorry, you've lost\n\n", sumOfDice, point); NewGame(); return play(); } public void AccountData() { String response; JOptionPane.showMessageDialog(null, "Hello, and Welcome to the Craps Game;"); while (true) { response = JOptionPane.showInputDialog(null, "Enter your starting account balance ($)"); try { startBalance = Double.parseDouble(response); } catch (NullPointerException e) { JOptionPane.showMessageDialog(null, "Error: The box cannot be empty!"); continue; } catch (NumberFormatException e) { JOptionPane.showMessageDialog(null, "Error: You must enter a numeric value in the box!"); continue; } if (startBalance >= MIN_BALANCE) break; System.out.printf("Please enter at least $%5.2f for a starting balance\n", MIN_BALANCE); } balance = startBalance; wins = 0; loss = 0; games = 0; play(); } public boolean update () { games++; if (win) { wins++; balance = balance - bet; } else { loss++; balance = balance - bet; } System.out.printf("Your total account balance is now: %4.2f", balance); if (balance < MIN_BET) { eligibletoPlay = false; System.out.println("Sadly, you are no longer eligible to play"); } return update(); } public void printreport() { if (games > 0) { double changed = balance - startBalance; double percentchanged = (changed/startBalance) * 100; double percentwins = (wins/(double)games) * 100; double percentlosses = (loss/(double)games) * 100; System.out.printf("Of the %d games you have played you have won %d (%4.2f) , lost %d (%4.2f)", games, wins, percentwins, loss, percentlosses); if (changed < 0 ) { System.out.printf("Your balanced has decreased by %4.2f (%-4.2f)\n", changed, percentchanged); System.out.printf("You have no more money left, bye\n"); } else if (changed > 0) { System.out.printf("Your balanced has increased by %4.2f (%4.2f)\n", changed, percentchanged); System.out.printf("Bye, enjoy your winnings"); } } } public static int roll () { int dice1; int dice2; dice1 = 1 + (int)(6.0*Math.random()); dice2 = 1 + (int)(6.0*Math.random()); return (dice1 + dice2); } }
There is a problem with my program. I need the JOptionpane to pop up when I run the program and that I am unable to enter bets, the only option it gives me is to input y or no to play again or end the program.
Java Language:
import javax.swing.JOptionPane;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
import java.io.*;
import java.util.Random;
public class Craps
{
double balance, startBalance, bet;
int wins, loss, games;
boolean win;
boolean eligibletoPlay;
public enum Status { CONTINUE, WON, LOST };
final static int SNAKE_EYES = 2;
final static int TREY = 3;
final static int SEVEN = 7;
final static int ELEVEN = 11;
final static int BOX_CARS = 12;
final static double MIN_BALANCE = 200.00;
final static double MIN_BET = 1.00;
Scanner input;
public static void main ()
{
Craps game = new Craps();
game.NewGame();
}
public void NewGame()
{
String answer;
input = new Scanner(System.in);
while (true)
{
System.out.print("Do you wish to play a new game? ");
answer = input.next();
if (answer.toLowerCase().charAt(0) == 'y')
{
play();
}
else if (answer.toLowerCase().charAt(0) == 'n')
{
printreport();
System.exit(5);
}
else
System.out.println("Please type in either 'y' to play again or 'n' to quit");
}
}
public boolean play ()
{
int point = 0;
int sumOfDice = roll();
Status gameStatus;
switch (sumOfDice)
{
case SEVEN:
case ELEVEN:
gameStatus = Status.WON;
System.out.printf("Player rolls: %d\n", sumOfDice);
break;
case SNAKE_EYES:
case TREY:
case BOX_CARS:
gameStatus = Status.LOST;
System.out.printf("Player rolls: %d\n", sumOfDice);
break;
default:
gameStatus = Status.CONTINUE;
point = sumOfDice;
System.out.printf("Player rolls: %d\n", point);
}
while ( gameStatus == Status.CONTINUE )
{
sumOfDice = roll();
System.out.printf("Player rolls: %d\n", sumOfDice);
if (sumOfDice == point)
{
gameStatus = Status.WON;
}
else if (sumOfDice == SEVEN)
{
gameStatus = Status.LOST;
}
}
if ( gameStatus == Status.WON )
{
System.out.printf("Congratulations, You win\n\n", sumOfDice);
}
else
System.out.printf("Sorry, you've lost\n\n", sumOfDice, point);
NewGame();
return play();
}
public void AccountData()
{
String response;
JOptionPane.showMessageDialog(null, "Hello, and Welcome to the Craps Game;");
while (true)
{
response = JOptionPane.showInputDialog(null, "Enter your starting account balance ($)");
try
{
startBalance = Double.parseDouble(response);
}
catch (NullPointerException e)
{
JOptionPane.showMessageDialog(null, "Error: The box cannot be empty!");
continue;
}
catch (NumberFormatException e)
{
JOptionPane.showMessageDialog(null, "Error: You must enter a numeric value in the box!");
continue;
}
if (startBalance >= MIN_BALANCE)
break;
System.out.printf("Please enter at least $%5.2f for a starting balance\n", MIN_BALANCE);
}
balance = startBalance;
wins = 0;
loss = 0;
games = 0;
play();
}
public boolean update ()
{
games++;
if (win)
{
wins++;
balance = balance - bet;
}
else
{
loss++;
balance = balance - bet;
}
System.out.printf("Your total account balance is now: %4.2f", balance);
if (balance < MIN_BET)
{
eligibletoPlay = false;
System.out.println("Sadly, you are no longer eligible to play");
}
return update();
}
public void printreport()
{
if (games > 0)
{
double changed = balance - startBalance;
double percentchanged = (changed/startBalance) * 100;
double percentwins = (wins/(double)games) * 100;
double percentlosses = (loss/(double)games) * 100;
System.out.printf("Of the %d games you have played you have won %d (%4.2f) , lost %d (%4.2f)", games, wins, percentwins, loss, percentlosses);
if (changed < 0 )
{
System.out.printf("Your balanced has decreased by %4.2f (%-4.2f)\n", changed, percentchanged);
System.out.printf("You have no more money left, bye\n");
}
else if (changed > 0)
{
System.out.printf("Your balanced has increased by %4.2f (%4.2f)\n", changed, percentchanged);
System.out.printf("Bye, enjoy your winnings");
}
}
}
public static int roll ()
{
int dice1;
int dice2;
dice1 = 1 + (int)(6.0*Math.random());
dice2 = 1 + (int)(6.0*Math.random());
return (dice1 + dice2);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

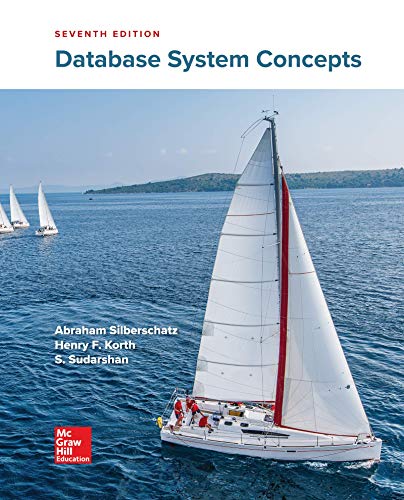
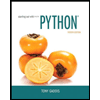
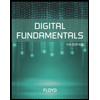
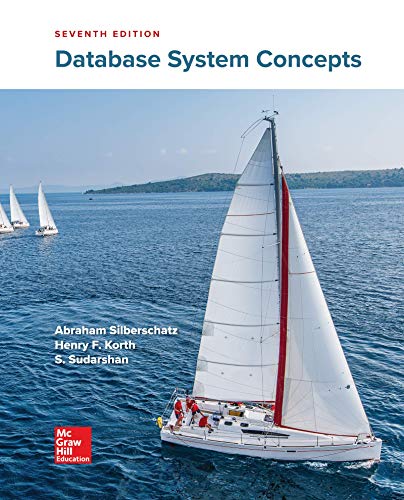
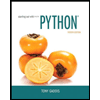
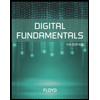
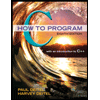
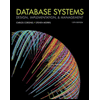
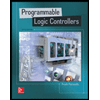