Node.java import java.util.HashMap; import java.util.Map; import java.util.Properties; public abstract class Node { private String type; private Map properties = new HashMap<>(); public Node(String type) { this.type = type; }
Node.java import java.util.HashMap; import java.util.Map; import java.util.Properties; public abstract class Node { private String type; private Map properties = new HashMap<>(); public Node(String type) { this.type = type; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Below is Node.java and ProgramNode.java. There is an error in ProgramNode.java, so make sure to fix that error and show the fixed code with the screenshot of the working code.
Node.java
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
public abstract class Node {
private String type;
private Map<String, String> properties = new HashMap<>();
public Node(String type) {
this.type = type;
}
public String getType() {
return type;
}
public void addProperty(String key, String value) {
properties.put(key, value);
}
public String getProperty(String key) {
return properties.get(key);
}
public String ToString() {
return null;
}
}
ProgramNode.java
import javax.swing.ActionMap;
public class ProgramNode extends Node {
private String programName;
private int programId;
public ProgramNode(String programName, int programId) {
this.programName = programName;
this.programId = programId;
}
@Override
public String ToString() {
return "ProgramNode{" +
"programName='" + programName + '\'' +
", programId=" + programId +
'}';
}
}
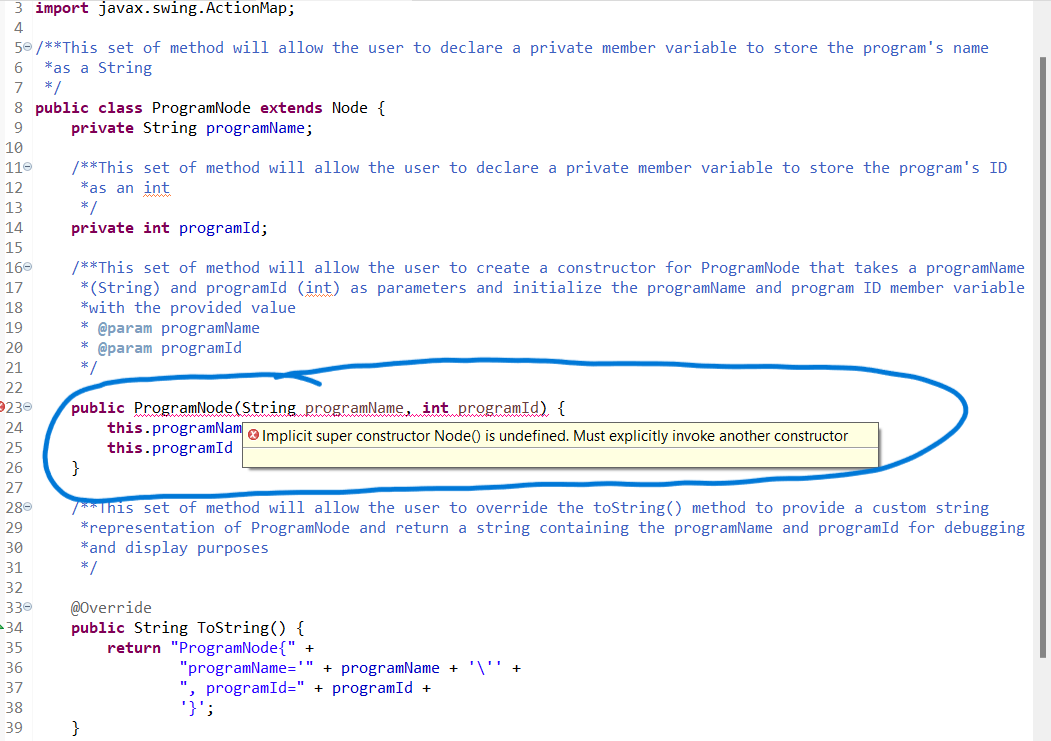
Transcribed Image Text:3 import javax.swing.ActionMap;
4
50 /**This set of method will allow the user to declare a private member variable to store the program's name
6 *as a String
7
*/
8 public class ProgramNode extends Node {
9
private String programName;
10
110
12
13
14
15
160
17
18
19
20
21
22
9230
24
25
26
27
280
29
30
31
32
330
34
35
36
37
38
39
/**This set of method will allow the user to declare a private member variable to store the program's ID
*as an int
*/
private int programId;
/**This set of method will allow the user to create a constructor for ProgramNode that takes a programName
*(String) and programId (int) as parameters and initialize the programName and program ID member variable
*with the provided value
* @param programName
* @param programId
*/
public ProgramNode(String programName, int programId) {
}
this.programNam
this.programId
> Implicit super constructor Node() is undefined. Must explicitly invoke another constructor
/**This set of method will allow the user to override the toString() method to provide a custom string
*representation of ProgramNode and return a string containing the programName and programId for debugging
*and display purposes
*/
}
@Override
public String ToString() {
return "ProgramNode{" +
"programName="" + programName +
", programId=" + programId +
'}';
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
There is an error for type in ProgramNode.java. Please fix the error and show the fixed code for ProgramNode.java with the screenshot of the working code.
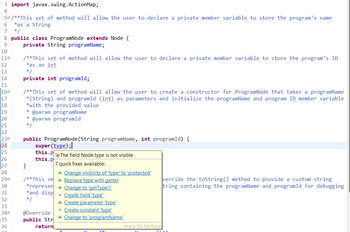
Transcribed Image Text:3 import javax.swing. ActionMap;
4
50 /**This set of method will allow the user to declare a private member variable to store the program's name
6 *as a String
7
*/
8 public class ProgramNode extends Node {
9
private String programName;
10
110
12
13
14
15
160
17
18
19
20
21
22
230
24
25
26
27
28
290
30
31
32
33
340
-35
36
/**This set of method will allow the user to declare a private member variable to store the program's ID
*as an int
*/
private int programId;
/**This set of method will allow the user to create a constructor for ProgramNode that takes a programName
*(String) and programId (int) as parameters and initialize the programName and program ID member variable
*with the provided value
* @param programName
@param programId
*/
public ProgramNode (String programName, int programId) {
super (type);
this.p The field Node is not visible
this.p
7 quick fixes available:
}
/**This se
*represen
*and disp
*/
@Override
public Str
return
Change visibility of 'type' to 'protected'
Replace type with getter
Change to 'getType()'
Create field 'type'
Create parameter 'type'
• Create constant 'type'
Change to 'programName'
Press 'F2' for focus
verride the toString() method to provide a custom string
tring containing the programName and programId for debugging
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
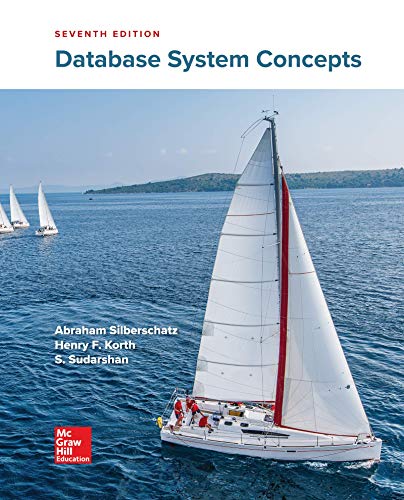
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
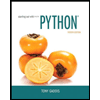
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
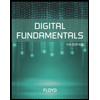
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
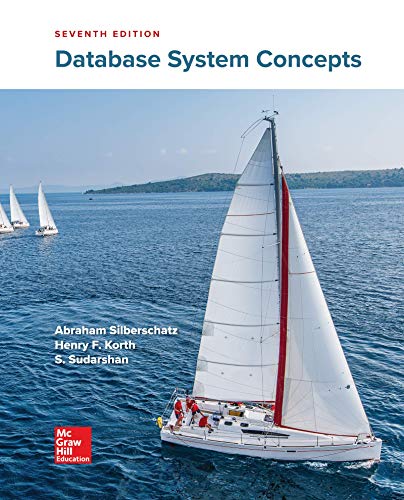
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
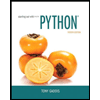
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
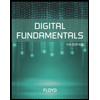
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
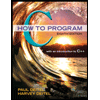
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
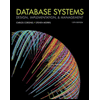
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
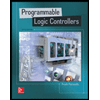
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education