There's an error in the part that "function" was not declared in the code. Can you fix the code? Code: #include #include #include using namespace std; struct Process { int processId; int burstTime; int priority; }; // Function to calculate average waiting time and average turnaround time void calculateAvgTimes(vector& processes, vector& waitingTimes, vector& turnaroundTimes) { int totalProcesses = processes.size(); int totalWaitingTime = 0; int totalTurnaroundTime = 0; for (int i = 0; i < totalProcesses; i++) { totalWaitingTime += waitingTimes[i]; totalTurnaroundTime += turnaroundTimes[i]; } float avgWaitingTime = (float)totalWaitingTime / totalProcesses; float avgTurnaroundTime = (float)totalTurnaroundTime / totalProcesses; cout << "Average Waiting Time: " << avgWaitingTime << endl; cout << "Average Turnaround Time: " << avgTurnaroundTime << endl; } // Function to perform Multilevel Queue Scheduling void multilevelQueueScheduling(vector& processes) { int totalProcesses = processes.size(); // Queues for different levels of scheduling queue fcfsQueue; queue rrQueue; priority_queue, function> priorityQueue( [](const Process& p1, const Process& p2) { return p1.priority > p2.priority; }); // Splitting the processes into different queues based on priority for (int i = 0; i < totalProcesses; i++) { if (processes[i].priority == 1) fcfsQueue.push(processes[i]); else if (processes[i].priority == 2) rrQueue.push(processes[i]); else if (processes[i].priority == 3) priorityQueue.push(processes[i]); } // Vector to store waiting times and turnaround times vector waitingTimes(totalProcesses, 0); vector turnaroundTimes(totalProcesses, 0); // FCFS Scheduling int time = 0; while (!fcfsQueue.empty()) { Process currentProcess = fcfsQueue.front(); fcfsQueue.pop(); waitingTimes[currentProcess.processId - 1] = time; time += currentProcess.burstTime; turnaroundTimes[currentProcess.processId - 1] = time; } // RR Scheduling while (!rrQueue.empty()) { Process currentProcess = rrQueue.front(); rrQueue.pop(); waitingTimes[currentProcess.processId - 1] = time; if (currentProcess.burstTime > 2) { currentProcess.burstTime -= 2; time += 2; rrQueue.push(currentProcess); } else { time += currentProcess.burstTime; turnaroundTimes[currentProcess.processId - 1] = time; } } // Priority Scheduling while (!priorityQueue.empty()) { Process currentProcess = priorityQueue.top(); priorityQueue.pop(); waitingTimes[currentProcess.processId - 1] = time; time += currentProcess.burstTime; turnaroundTimes[currentProcess.processId - 1] = time; } // Displaying Gantt Chart cout << "Gantt Chart:\n"; for (int i = 0; i < totalProcesses; i++) { cout << "P" << processes[i].processId << "\t"; } cout << endl; for (int i = 0; i < totalProcesses; i++) { cout << waitingTimes[i] << "\t"; } cout << endl; calculateAvgTimes(processes, waitingTimes, turnaroundTimes); } int main() { vector processes = { {1, 8, 4}, {2, 6, 1}, {3, 1, 2}, {4, 9, 2}, {5, 3, 3} }; multilevelQueueScheduling(processes); return 0;
There's an error in the part that "function" was not declared in the code. Can you fix the code?
Code:
#include <iostream>
#include <queue>
#include <
using namespace std;
struct Process {
int processId;
int burstTime;
int priority;
};
// Function to calculate average waiting time and average turnaround time
void calculateAvgTimes(vector<Process>& processes, vector<int>& waitingTimes, vector<int>& turnaroundTimes) {
int totalProcesses = processes.size();
int totalWaitingTime = 0;
int totalTurnaroundTime = 0;
for (int i = 0; i < totalProcesses; i++) {
totalWaitingTime += waitingTimes[i];
totalTurnaroundTime += turnaroundTimes[i];
}
float avgWaitingTime = (float)totalWaitingTime / totalProcesses;
float avgTurnaroundTime = (float)totalTurnaroundTime / totalProcesses;
cout << "Average Waiting Time: " << avgWaitingTime << endl;
cout << "Average Turnaround Time: " << avgTurnaroundTime << endl;
}
// Function to perform Multilevel Queue Scheduling
void multilevelQueueScheduling(vector<Process>& processes) {
int totalProcesses = processes.size();
// Queues for different levels of scheduling
queue<Process> fcfsQueue;
queue<Process> rrQueue;
priority_queue<Process, vector<Process>, function<bool(const Process&, const Process&)>> priorityQueue(
[](const Process& p1, const Process& p2) { return p1.priority > p2.priority; });
// Splitting the processes into different queues based on priority
for (int i = 0; i < totalProcesses; i++) {
if (processes[i].priority == 1)
fcfsQueue.push(processes[i]);
else if (processes[i].priority == 2)
rrQueue.push(processes[i]);
else if (processes[i].priority == 3)
priorityQueue.push(processes[i]);
}
// Vector to store waiting times and turnaround times
vector<int> waitingTimes(totalProcesses, 0);
vector<int> turnaroundTimes(totalProcesses, 0);
// FCFS Scheduling
int time = 0;
while (!fcfsQueue.empty()) {
Process currentProcess = fcfsQueue.front();
fcfsQueue.pop();
waitingTimes[currentProcess.processId - 1] = time;
time += currentProcess.burstTime;
turnaroundTimes[currentProcess.processId - 1] = time;
}
// RR Scheduling
while (!rrQueue.empty()) {
Process currentProcess = rrQueue.front();
rrQueue.pop();
waitingTimes[currentProcess.processId - 1] = time;
if (currentProcess.burstTime > 2) {
currentProcess.burstTime -= 2;
time += 2;
rrQueue.push(currentProcess);
} else {
time += currentProcess.burstTime;
turnaroundTimes[currentProcess.processId - 1] = time;
}
}
// Priority Scheduling
while (!priorityQueue.empty()) {
Process currentProcess = priorityQueue.top();
priorityQueue.pop();
waitingTimes[currentProcess.processId - 1] = time;
time += currentProcess.burstTime;
turnaroundTimes[currentProcess.processId - 1] = time;
}
// Displaying Gantt Chart
cout << "Gantt Chart:\n";
for (int i = 0; i < totalProcesses; i++) {
cout << "P" << processes[i].processId << "\t";
}
cout << endl;
for (int i = 0; i < totalProcesses; i++) {
cout << waitingTimes[i] << "\t";
}
cout << endl;
calculateAvgTimes(processes, waitingTimes, turnaroundTimes);
}
int main() {
vector<Process> processes = {
{1, 8, 4},
{2, 6, 1},
{3, 1, 2},
{4, 9, 2},
{5, 3, 3}
};
multilevelQueueScheduling(processes);
return 0;
}
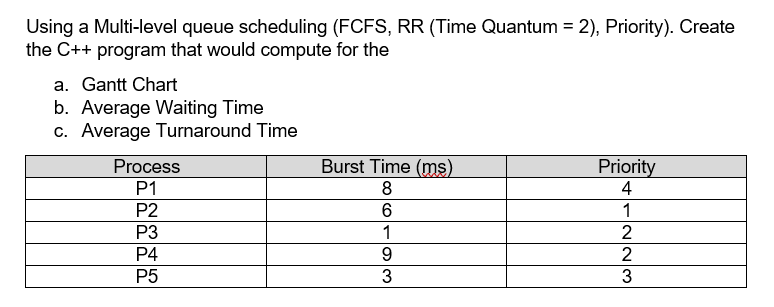

Step by step
Solved in 5 steps with 3 images

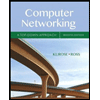
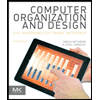
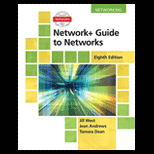
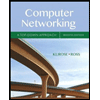
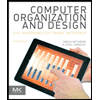
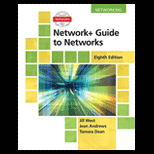
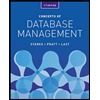
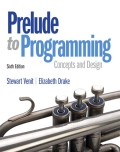
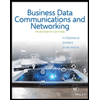