This code by C to : 1- Create a sorted linked list using tenStudent array (copy from array into the linked list will be done). 2-Append an element to the end of a list and Details in the picture: >>>>>>>>>>>>>>>>>>>>>>> I want to update the code to : 1- Delete the last element from a list. 2- Delete the nth element from a list. The code: #include struct student { int TC; char F_name[12]; char L_name[12]; int age; char gender[2]; }; struct Linked_list_node { struct student info; struct node* next; }; struct student tenStudent[10] = {
This code by C to :
1- Create a sorted linked list using tenStudent array (copy from array into the linked list will be done).
2-Append an element to the end of a list
and Details in the picture:
>>>>>>>>>>>>>>>>>>>>>>>
I want to update the code to :
1- Delete the last element from a list.
2- Delete the nth element from a list.
The code:
#include<stdio.h>
struct student
{
int TC;
char F_name[12];
char L_name[12];
int age;
char gender[2];
};
struct Linked_list_node
{
struct student info;
struct node* next;
};
struct student tenStudent[10] = {
123,"X1","Y1",21,"M",
234,"X2","Y2",26,"F",
128,"X3","Y3",18,"M",
432,"X4","Y4",27,"M",
287,"X5","Y5",34,"F",
423,"X6","Y6",21,"M",
634,"X7","Y7",16,"F",
828,"X8","Y8",15,"M",
252,"X9","Y9",27,"F",
887,"X10","Y10",34,"F",
};
int main()
{
//create a head node for linked list
struct Linked_list_node* head = (struct Linked_list_node*)malloc(sizeof(struct Linked_list_node));
//add first student to the linked list
head->info = tenStudent[0];
//set next pointer to null
head->next = NULL;
//assign a temporary pointer for linked list
struct Linked_list_node* ptr = head;
int i = 1;
//for each student in the given array, add it to the linked list
while (i < 10)
{
//create a new node of linked list
struct Linked_list_node* n = (struct Linked_list_node*)malloc(sizeof(struct Linked_list_node));
n->info = tenStudent[i];
n->next = NULL;
//add it to the linked list
ptr->next = n;
ptr = ptr->next;
i++;
}
struct Linked_list_node* ptr1 = head;
struct Linked_list_node* ptr2 = head;
//sort the linked list on the basis of TC number
while (ptr1 != NULL)
{
ptr2 = ptr1->next;
while (ptr2 != NULL)
{
if (ptr1->info.TC > ptr2->info.TC)
{
struct student temp = ptr1->info;
ptr1->info = ptr2->info;
ptr2->info = temp;
}
ptr2 = ptr2->next;
}
ptr1 = ptr1->next;
}
//print the linked list
ptr = head;
printf("Sorted linked list\n");
while (ptr != NULL)
{
printf("%d\t", ptr->info.TC);
printf("%s\t", ptr->info.F_name);
printf("%s\t", ptr->info.L_name);
printf("%d\t", ptr->info.age);
printf("%s\n", ptr->info.gender);
ptr = ptr->next;
}
//create a new student node
struct student new_stu = { 600,"X11","Y11",56,"M" };
struct Linked_list_node* n = (struct Linked_list_node*)malloc(sizeof(struct Linked_list_node));
n->info = new_stu;
n->next = NULL;
ptr = head;
//take the pointer to the end of linked list
while (ptr->next != NULL)
{
ptr = ptr->next;
}
//add the new node at the end of linked list
ptr->next = n;
ptr = head;
//again print the linked list
printf("Linked list after adding new node at the end\n");
while (ptr != NULL)
{
printf("%d\t", ptr->info.TC);
printf("%s\t", ptr->info.F_name);
printf("%s\t", ptr->info.L_name);
printf("%d\t", ptr->info.age);
printf("%s\n", ptr->info.gender);
ptr = ptr->next;
}
}
![struct student
{
int TC;
char F_name[12];
char L_name[12];
int age;
char gender[2];/*M=male,F=Female*/
};
struct Linked_list_node
{
struct student info;
struct node *next;
};
struct Tree_node
{
struct node *left ;
struct person info ;
struct node *right;
};
sruct student tenStudent[10]={
123,"X1", "Y1", 21, "M",
234,"X2","Ү2", 26, "F",
128,"ХЗ","ҮЗ",18,"М",
432,"X4", "Y4", 27,"M",
287,"Х5","Ү5",34,"F",
423,"Х6", "Y6", 21, "M",
634, "X7", "Ү7", 16, "F",
828,"х8", "Y8", 15,"M",
252,"X9" ,"Y9",27,"F",
887,"X10" ,"Y10",34,"F"
};](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F469ef60b-3a4b-4208-81a9-0ddda0b04d6e%2F5b9825e3-1ca6-4801-9c91-8f3b5b2c1ca2%2Fbc0fk8l_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

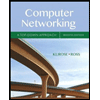
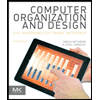
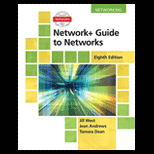
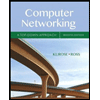
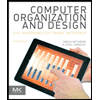
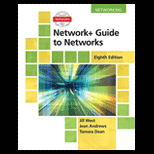
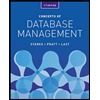
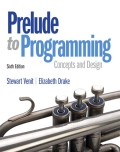
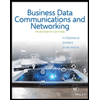