This exercise is about the bit-wise operators in C. Complete each function skeleton using only straight-line code (i.e., no loops, conditionals, or function calls) and limited of C arithmetic and logical C operators. Specifically, you are only allowed to use the following eight operators: ! ∼, &,ˆ|, + <<>>. For more details on the Bit-Level Integer Coding Rules on p. 128/129 of the text. A specific problem may restrict the list further: For example, to write a function to compute the bitwise xor of x and y, only using & |, ∼ int bitXor(int x, int y) { return ((x&∼y) | (∼x & y));} (a) /* copyLSbit:Set all bits of result to least significant bit of x * Example: copyLSB(5) = 0xFFFFFFFF, copyLSB(6) = 0x00000000 * Legal ops: ! ∼ & ˆ| + <<>> */ int copyLSbit(int x) { return 2;} (b) /* negate - return -x * Example: negate(1) = -1. * Legal ops:! ∼ & ˆ| + <<>> */ int negate(int x) { return 2; } (c) /* isEqual - return 1 if x == y, and 0 otherwise * Examples: isEqual(5,5) = 1, isEqual(4,5) = 0 * Legal ops: ! ∼ & ˆ| + <<>> */ int isEqual(int x, int y) { return 2; } (d) /* twoCmax: return maximum two’s complement integer Legal ops: ! ∼ & ˆ| + <<>>int twoCmax(void) { return 2; }
This exercise is about the bit-wise operators in C. Complete each function
skeleton using only straight-line code (i.e., no loops, conditionals, or function
calls) and limited of C arithmetic and logical C operators. Specifically, you
are only allowed to use the following eight operators: ! ∼, &,ˆ|, + <<>>.
For more details on the Bit-Level Integer Coding Rules on p. 128/129 of the
text. A specific problem may restrict the list further: For example, to write
a function to compute the bitwise xor of x and y, only using & |, ∼
int bitXor(int x, int y)
{ return ((x&∼y) | (∼x & y));}
(a) /*
copyLSbit:Set all bits of result to least significant bit of x
* Example: copyLSB(5) = 0xFFFFFFFF, copyLSB(6) = 0x00000000
* Legal ops: ! ∼ & ˆ| + <<>>
*/
int copyLSbit(int x) {
return 2;}
(b) /* negate - return -x
* Example: negate(1) = -1.
* Legal ops:! ∼ & ˆ| + <<>>
*/ int negate(int x) { return 2; }
(c) /* isEqual - return 1 if x == y, and 0 otherwise
* Examples: isEqual(5,5) = 1, isEqual(4,5) = 0
* Legal ops: ! ∼ & ˆ| + <<>>
*/ int isEqual(int x, int y) { return 2; }
(d) /* twoCmax: return maximum two’s complement integer
Legal ops: ! ∼ & ˆ| + <<>>int twoCmax(void)
{ return 2; }
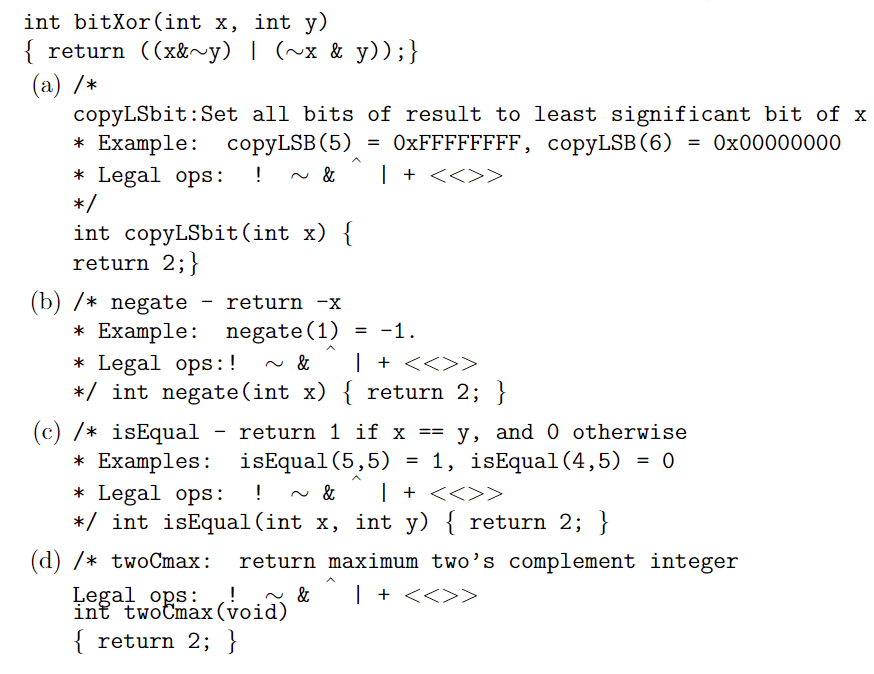

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

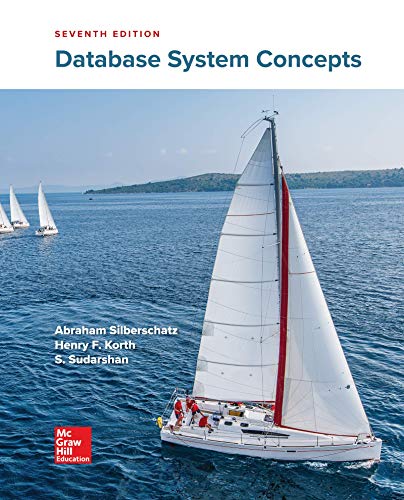
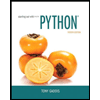
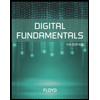
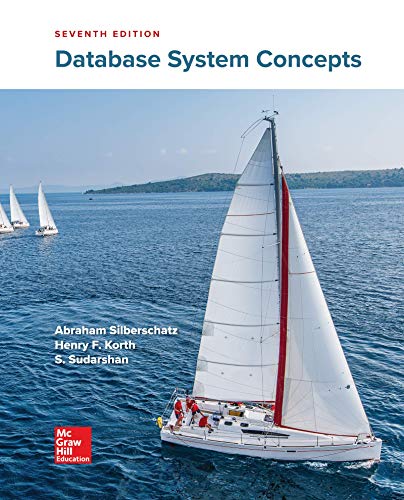
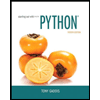
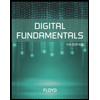
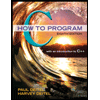
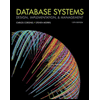
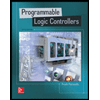