This function is used to create an user accounts dictionary and another login dictionary. The given argument is the filename to load. Every line in the file should be in the following format: username - password The key is a username and the value is a password. If the username and password fulfills the requirements, add the username and password into the user accounts dictionary. To make sure that the password fulfills these requirements, be sure to use the signup function that you wrote above. For the login dictionary, the key is the username, and its value indicates whether the user is logged in, or not. Initially, all users are not logged in. What you will do: - Create an empty user accounts dictionary and an empty login dictionary. - Read in the file. - If the username and password fulfills the requirements, adds the username and password into the user accounts dictionary, and updates the login dictionary. - You should also handle the following cases: -- When the password is missing. If so, ignore that line and don't update the dictionaries. -- When there is whitespace at the beginning or end of a line and/or between the name and password on a line. You should trim any and all whitespace. - Return both the user accounts dictionary and login dictionary from this function. For example, here's how your code should handle some specific lines in the file: The 1st line in the file has a name and password: Brandon - brandon123ABC Your code will process this line, and using the signup function, will add the extracted information to the dictionaries. After it does, the dictionaries will look like this: user_accounts = {"Brandon": "brandon123ABC"} log_in = {"Brandon": False} The 2nd line in the file has a name but missing password: Jack Your code will ignore this line. The dictionaries will still look like this: user_accounts = {"Brandon": "brandon123ABC"} log_in = {"Brandon": False} The 3rd line in the file has a name and password: Jack - jac123 Your code will process this line, and using the signup function, will not add the extracted information to the dictionaries because the password is invalid. The dictionaries will still look like this: user_accounts = {"Brandon": "brandon123ABC"} log_in = {"Brandon": False} The 4th line in the file has a name and password: Jack - jack123POU Your code will process this line, and using the signup function, will add the extracted information to the dictionaries. After it does, the dictionaries will look like this: user_accounts = {"Brandon": "brandon123ABC, "Jack": "jack123POU"} log_in = {"Brandon": False, "Jack": False} After processing every line in the file, the dictionaries will look like this: user_accounts = {"Brandon": "brandon123ABC, "Jack": "jack123POU", "James": "100jamesABD", "Sarah": "sd896ssfJJH"} log_in = {"Brandon": False, "Jack": False, "James": False, "Sarah": False} Return the dictionaries from this function.
This function is used to create an user accounts dictionary and another login dictionary. The given argument is the
filename to load.
Every line in the file should be in the following format:
username - password
The key is a username and the value is a password. If the username and password fulfills the requirements,
add the username and password into the user accounts dictionary. To make sure that the password fulfills these
requirements, be sure to use the signup function that you wrote above.
For the login dictionary, the key is the username, and its value indicates whether the user is logged in, or not.
Initially, all users are not logged in.
What you will do:
- Create an empty user accounts dictionary and an empty login dictionary.
- Read in the file.
- If the username and password fulfills the requirements, adds the username and password
into the user accounts dictionary, and updates the login dictionary.
- You should also handle the following cases:
-- When the password is missing. If so, ignore that line and don't update the dictionaries.
-- When there is whitespace at the beginning or end of a line and/or between the name and password on a line. You
should trim any and all whitespace.
- Return both the user accounts dictionary and login dictionary from this function.
For example, here's how your code should handle some specific lines in the file:
The 1st line in the file has a name and password:
Brandon - brandon123ABC
Your code will process this line, and using the signup function, will add the extracted information to the
dictionaries. After it does, the dictionaries will look like this:
user_accounts = {"Brandon": "brandon123ABC"}
log_in = {"Brandon": False}
The 2nd line in the file has a name but missing password:
Jack
Your code will ignore this line. The dictionaries will still look like this:
user_accounts = {"Brandon": "brandon123ABC"}
log_in = {"Brandon": False}
The 3rd line in the file has a name and password:
Jack - jac123
Your code will process this line, and using the signup function, will not add the extracted information to the
dictionaries because the password is invalid. The dictionaries will still look like this:
user_accounts = {"Brandon": "brandon123ABC"}
log_in = {"Brandon": False}
The 4th line in the file has a name and password:
Jack - jack123POU
Your code will process this line, and using the signup function, will add the extracted information to the
dictionaries. After it does, the dictionaries will look like this:
user_accounts = {"Brandon": "brandon123ABC, "Jack": "jack123POU"}
log_in = {"Brandon": False, "Jack": False}
After processing every line in the file, the dictionaries will look like this:
user_accounts = {"Brandon": "brandon123ABC, "Jack": "jack123POU", "James": "100jamesABD", "Sarah": "sd896ssfJJH"}
log_in = {"Brandon": False, "Jack": False, "James": False, "Sarah": False}
Return the dictionaries from this function.
THINGS IN BOLD ARE INSTRUCTIONS. PLEASE READ BEFORE STARTING
function is defined below:
def import_and_create_accounts(filename):

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

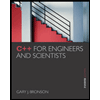
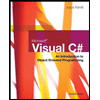
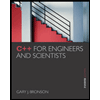
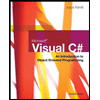