This is a substitution cypher Each letter is replaced by another letter with no repetition Ignore spaces and punctuation You must account for spaces (I ignored them, but there are other legitimate responses.) The best program will also account for punctuation and other symbols. (Ignoring them is fine.) Your program can ignore these special characters when they occur, but it should not crash if it encounters a strange character. Manage Capitalization In most cryptographic applications, all letters are converted to uppercase. Please follow this convention or deal with potential case conversion problems in another way. Use Functions You may not make significant changes to the main function. Determine which functions are necessary and create
This is a substitution cypher
Each letter is replaced by another letter with no repetition
Ignore spaces and punctuation
You must account for spaces (I ignored them, but there are other legitimate responses.)
The best program will also account for punctuation and other symbols. (Ignoring them is fine.) Your program can ignore these special characters when they occur, but it should not crash if it encounters a strange character.
Manage Capitalization
In most cryptographic applications, all letters are converted to uppercase. Please follow this convention or deal with potential case conversion problems in another way.
Use Functions
You may not make significant changes to the main function. Determine which functions are necessary and create them. (The blackbelt project does allow for one slight modification, noted below.) Do not turn in a program with only a main() function!! Write the code necessary for Python to run the main function as expected
Pass data between functions
Your functions may require some input and return some output. Part of the task is to determine how the methods are to be created.
Using a key
There's several workable answers, but I used a string with all the alphabet characters and a second string with a "scrambled" version of the alphabet.
Useful String methods
This program is all about string manipulation, so you may want to use help("str") in your python shell to look up some useful techniques. Here are a few things you might need to know how to do:
determine the length of a string
convert a string to upper case
find the position of a character in a string
Determine the character at a certain position in a string



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

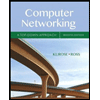
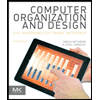
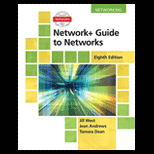
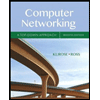
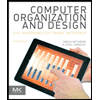
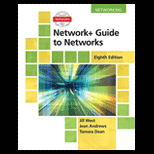
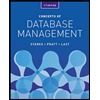
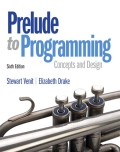
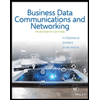