This is in C++ The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an int. At FIXME in the code, add a try/catch statement to catch ios_base::failure, and output 0 for the age. #include #include using namespace std; int main(int argc, char* argv[]) { string inputName; int age; // Set exception mask for cin stream cin.exceptions(ios::failbit); cin >> inputName; while(inputName != "-1") { // FIXME: The following line will throw an ios_base::failure. // Insert a try/catch statement to catch the exception. // Clear cin's failbit to put cin in a useable state. cin >> age; cout << inputName << " " << (age + 1) << endl; cin >> inputName; } return 0; } EX INPUT Lee 18 Lua 21 Mary Beth 19 Stu 33 -1 EX OUTPUT Lee 19 Lua 22 Mary 0 Stu 34 Thanks!!
This is in C++
The given
#include <string>
#include <iostream>
using namespace std;
int main(int argc, char* argv[]) {
string inputName;
int age;
// Set exception mask for cin stream
cin.exceptions(ios::failbit);
cin >> inputName;
while(inputName != "-1") {
// FIXME: The following line will throw an ios_base::failure.
// Insert a try/catch statement to catch the exception.
// Clear cin's failbit to put cin in a useable state.
cin >> age;
cout << inputName << " " << (age + 1) << endl;
cin >> inputName;
}
return 0;
}
EX INPUT
Lee 18
Lua 21
Mary Beth 19
Stu 33
-1
EX OUTPUT
Lee 19
Lua 22
Mary 0
Stu 34
Thanks!!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

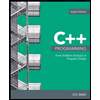
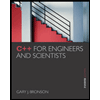
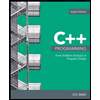
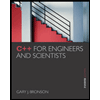