this is insturction Write a program which prompts the user to enter up to 15 floating point numbers (terminated with a non-numeric), reads them into an array, then prints the following. The program must use an array (not a vector) to get credit. The array values in the original order, separated by commas, with no trailing comma at the last value. The array values where the order of each adjacent pair of elements is swapped, separated by commas (NOT every element swapped with its next neighbor, which would just move the first element to the end, but every original pair swapped only in that pair. For an odd element count, the last element should not change. See examples below.) The smallest number in the array. Example program outputs with user input shown in bold font: Enter up to 15 numbers, and a non-numeric to quit: 1 0 -1.001 # Original array: 1, 0, -1.001 The swapped array: 0, 1, -1.001 Smallest number: -1.001 Enter up to 15 numbers, and a non-numeric to quit: 1 0 -1.001 5 , Original array: 1, 0, -1.001, 5 The swapped array: 0, 1, 5, -1.001 Smallest number: -1.001 provide some comments for code and must done c++ I am getting errors and attached the picture. Could you check please and fix the error? thanks. this code #include using namespace std; int main() { ,const, const int maxSize = 15; double numbers[maxSize]; int count = 0; // Read input numbers until non-numeric input or reaching the maximum size of the array while (count < maxSize && cin >> numbers[count]) { count++; } cout << "Original array: "; // Print the original array values separated by commas for (int i = 0; i < count; i++) { cout << numbers[i]; if (i < count - 1) { cout << ", "; } } cout << endl << "The swapped array: "; // Print the array values where each adjacent pair of elements is swapped, except for the last element (if the count is odd) for (int i = 0; i < count - 1; i += 2) { cout << numbers[i + 1] << ", " << numbers[i]; if (i + 2 < count - 1) { cout << ", "; } } // Print the last element if the count is odd if (count % 2 != 0) { cout << ", " << numbers[count - 1]; } // Find the smallest number in the array double smallest = numbers[0]; for (int i = 1; i < count; i++) { if (numbers[i] < smallest) { smallest = numbers[i]; } } cout << endl << "Smallest number: " << smallest << endl; return 0;
this is insturction
Write a program which prompts the user to enter up to 15 floating point numbers (terminated with a non-numeric), reads them into an array, then prints the following. The program must use an array (not a
- The array values in the original order, separated by commas, with no trailing comma at the last value.
- The array values where the order of each adjacent pair of elements is swapped, separated by commas (NOT every element swapped with its next neighbor, which would just move the first element to the end, but every original pair swapped only in that pair. For an odd element count, the last element should not change. See examples below.)
- The smallest number in the array.
Example program outputs with user input shown in bold font:
Enter up to 15 numbers, and a non-numeric to quit:
1 0 -1.001 #
Original array: 1, 0, -1.001 The swapped array: 0, 1, -1.001 Smallest number: -1.001 Enter up to 15 numbers, and a non-numeric to quit:
1 0 -1.001 5 ,
Original array: 1, 0, -1.001, 5 The swapped array: 0, 1, 5, -1.001 Smallest number: -1.001
provide some comments for code and must done c++
I am getting errors and attached the picture. Could you check please and fix the error? thanks.
this code
#include <iostream>
using namespace std;
int main() {
,const, const int maxSize = 15;
double numbers[maxSize];
int count = 0;
// Read input numbers until non-numeric input or reaching the maximum size of the array
while (count < maxSize && cin >> numbers[count]) {
count++;
}
cout << "Original array: ";
// Print the original array values separated by commas
for (int i = 0; i < count; i++) {
cout << numbers[i];
if (i < count - 1) {
cout << ", ";
}
}
cout << endl << "The swapped array: ";
// Print the array values where each adjacent pair of elements is swapped, except for the last element (if the count is odd)
for (int i = 0; i < count - 1; i += 2) {
cout << numbers[i + 1] << ", " << numbers[i];
if (i + 2 < count - 1) {
cout << ", ";
}
}
// Print the last element if the count is odd
if (count % 2 != 0) {
cout << ", " << numbers[count - 1];
}
// Find the smallest number in the array
double smallest = numbers[0];
for (int i = 1; i < count; i++) {
if (numbers[i] < smallest) {
smallest = numbers[i];
}
}
cout << endl << "Smallest number: " << smallest << endl;
return 0;
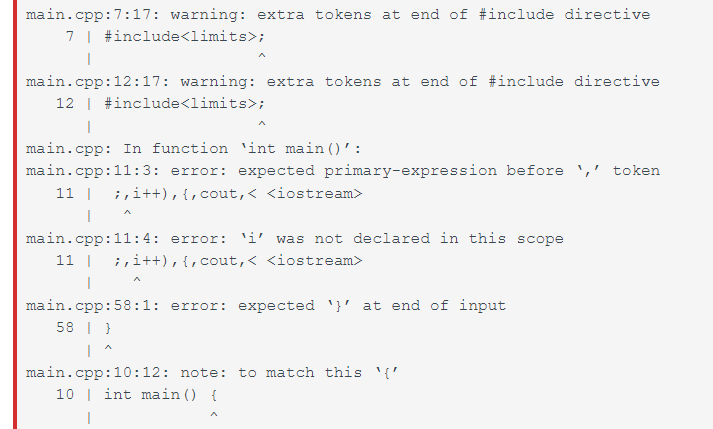

Step by step
Solved in 5 steps with 2 images

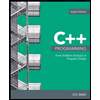
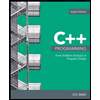