this is my code, but after automatically check it, it shows smt went wrong(image one) image two is the spec for nextTweet and below is my code, since it's the system automatically check the code, I dont really have a textcases. plz debug it and fix it. show the screenshot of the code when u finish. thanks Below is my whole code for TweetBot import java.util.*; import java.io.*; public class TweetBot { private List tweets; private int index; public TweetBot(List tweets){ if(tweets.size() < 1){ throw new IllegalArgumentException("need contain at least one tweet!"); } this.tweets = new ArrayList<>(tweets); } public int numTweets(){ return tweets.size(); } public void addTweet(String tweet) { tweets.add(tweet); if (tweets.size() == 1) { // if the tweet was the first one added, set the index to 0 index = tweets.size() - 1; } } public String nextTweet() { if (tweets.size() == 1) { index = 0; return tweets.get(0); } else { if (index == tweets.size()) { index = 0; } String tweet = tweets.get(index); index++; return tweet; } } public void removeTweet(String tweet) { int tweetIndex = tweets.indexOf(tweet); if (tweetIndex == -1) { // Tweet was not found in the list, so the state of the TweetBot should be unchanged return; } tweets.remove(tweetIndex); if (index > tweetIndex) { // If the removed tweet was before the current index, shift the index back by 1 index--; } else if (index == tweets.size()) { // If the current index is now out of bounds, set it to the last index in the list index = tweets.size() - 1; } } public void reset(){ index = 0; } } and here is the main class method (ignore the most frequent word) import java.util.*; import java.io.*; public class TwitterMain { public static void main(String[] args) throws FileNotFoundException { Scanner input = new Scanner(new File("tweets.txt")); // Make Scanner over tweet file List tweets = new ArrayList<>(); while (input.hasNextLine()) { // Add each tweet in file to List tweets.add(input.nextLine()); } TweetBot bot = new TweetBot(tweets); // Create TweetBot object with list of tweets System.out.println(bot.nextTweet()); // should print "tweet about something controversial" System.out.println(bot.nextTweet()); // should print "Remember to vote!" System.out.println(bot.nextTweet()); // should print "Look at this meme:0" System.out.println(bot.nextTweet()); // should print "tweet about something controversial" (because the index has wrapped around) bot.addTweet("This is a new tweet!"); System.out.println(bot.nextTweet()); // should print "This is a new tweet!" bot.addTweet("Hii, How are everyone?"); System.out.println(bot.nextTweet()); // should print "Hii, How are everyone?" TwitterTrends trends = new TwitterTrends(bot); // Create TwitterTrends object // TODO: Call and display results from getMostFrequentWord and your // creative method here String mostFrequentWord = trends.getMostFrequentWord(); System.out.println("The most frequent word is: " + mostFrequentWord); } }
this is my code, but after automatically check it, it shows smt went wrong(image one)
image two is the spec for nextTweet and below is my code, since it's the system automatically check the code, I dont really have a textcases. plz debug it and fix it. show the screenshot of the code when u finish. thanks
Below is my whole code for TweetBot
import java.util.*;
import java.io.*;
public class TweetBot {
private List<String> tweets;
private int index;
public TweetBot(List<String> tweets){
if(tweets.size() < 1){
throw new IllegalArgumentException("need contain at least one tweet!");
}
this.tweets = new ArrayList<>(tweets);
}
public int numTweets(){
return tweets.size();
}
public void addTweet(String tweet) {
tweets.add(tweet);
if (tweets.size() == 1) {
// if the tweet was the first one added, set the index to 0
index = tweets.size() - 1;
}
}
public String nextTweet() {
if (tweets.size() == 1) {
index = 0;
return tweets.get(0);
} else {
if (index == tweets.size()) {
index = 0;
}
String tweet = tweets.get(index);
index++;
return tweet;
}
}
public void removeTweet(String tweet) {
int tweetIndex = tweets.indexOf(tweet);
if (tweetIndex == -1) {
// Tweet was not found in the list, so the state of the TweetBot should be unchanged
return;
}
tweets.remove(tweetIndex);
if (index > tweetIndex) {
// If the removed tweet was before the current index, shift the index back by 1
index--;
} else if (index == tweets.size()) {
// If the current index is now out of bounds, set it to the last index in the list
index = tweets.size() - 1;
}
}
public void reset(){
index = 0;
}
}
and here is the main class method (ignore the most frequent word)
import java.util.*;
import java.io.*;
public class TwitterMain {
public static void main(String[] args) throws FileNotFoundException {
Scanner input = new Scanner(new File("tweets.txt")); // Make Scanner over tweet file
List<String> tweets = new ArrayList<>();
while (input.hasNextLine()) { // Add each tweet in file to List
tweets.add(input.nextLine());
}
TweetBot bot = new TweetBot(tweets); // Create TweetBot object with list of tweets
System.out.println(bot.nextTweet()); // should print "tweet about something controversial"
System.out.println(bot.nextTweet()); // should print "Remember to vote!"
System.out.println(bot.nextTweet()); // should print "Look at this meme:0"
System.out.println(bot.nextTweet()); // should print "tweet about something controversial" (because the index has wrapped around)
bot.addTweet("This is a new tweet!");
System.out.println(bot.nextTweet()); // should print "This is a new tweet!"
bot.addTweet("Hii, How are everyone?");
System.out.println(bot.nextTweet()); // should print "Hii, How are everyone?"
TwitterTrends trends = new TwitterTrends(bot); // Create TwitterTrends object
// TODO: Call and display results from getMostFrequentWord and your
// creative method here
String mostFrequentWord = trends.getMostFrequentWord();
System.out.println("The most frequent word is: " + mostFrequentWord);
}
}
![TESTCASES
Constructor throws exception correctly
numTweets works as intended
12/14 passed
addTweet works correctly
Constructor creates a copy of the tweets list rather than switching references (addTweet
must work)
numTweets updates after tweets are added
NumTweets updates after tweets are removed
nextTweet correctly iterates through a freshly constructed bot
nextTweet works correctly even after tweets are added
Failed: Make sure that nextTweet returns the most recently added tweet if there was previously only a
single tweet in the bot. ex. If bot=[t1], nextTweet would return t1. If addTweet(t1), then nextTweet
should return t2, not t1 again ==> expected: <lol hi XD> but was: <Does this work>
Show stacktrace
nextTweets works correctly when tweets are removed
nextTweets works correctly when tweets are removed at the location of next tweet
nextTweets works correctly when tweets are added and removed simultaneously
removeTweet does nothing in the case that the tweet is not in the bot
The reset method resets the state of the TwitterBot correctly
>
> X](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c680976-d041-43f6-b2b7-59630778639d%2Fbc5cb0f7-bc16-4ac6-b82b-361ace877cc9%2Fkm9qe5_processed.png&w=3840&q=75)
![public int numTweets()
Returns the number of tweets currently in the tweet list.
public void addTweet(String tweet)
Adds the given tweet to the end of the tweet list.
You may assume that the given tweet does not already exist in the TweetBot 's list of tweets.
public String next Tweet()
Returns the next tweet from the tweet list. If all the tweets in the list are exhausted, cycle around to the start of the
list.
For example, the following code snippet should produce the output in the comments. Suppose that tweets stores
a list of Tweets ["A tweet about something controversial", "Remember to vote!", "Look at this meme
:0"].
TweetBot bot = new TweetBot (tweets);
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
// A tweet about something controversial
// Remember to vote!
// Look at this meme :0
// A tweet about something controversial
iThink about how to get the next tweet from the list of tweets, and how you can cycle around to the start once you've gone
through all the tweets!
One Special Case!
As you're working on your TweetBot and testing your implementation, you'll likely start thinking about different
cases that your TweetBot will need to handle. There is one specific special case whose behavior is particularly
tricky, so we wanted to talk about it more in depth.
Consider the case where your TweetBot is storing a single tweet (referred to as tweet1), and you call nextTweet()
on your TweetBot. Then, you immediately afterwards add in another tweet (referred to as tweet2). On the next
call to next Tweet (), your TweetBot should return tweet2 (the tweet that was just added in) -- NOT tweet1.
i Note that the case described here is a tricky one - really there are couple of different ways that a TweetBot could reasonably
handle this situation. But, since you'll be writing code to pass tests that we provide, we're telling you how you should handle
this situation for this assignment!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c680976-d041-43f6-b2b7-59630778639d%2Fbc5cb0f7-bc16-4ac6-b82b-361ace877cc9%2Fk5inkbd_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

but on ur full java program's nextTweet method... u didnt change it.
and after I fixed the code like u said: here is my (wrong) output:
A tweet about something controversial
Remember to vote!
A tweet about something controversial
Remember to vote!
Look at this meme :O
This is a new tweet!
missing: Hii, How are everyone?
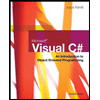
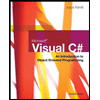