This is the final version of the Picross code development and your task is to produce a program that will allow the user to solve the puzzle. Feel free to take provided solutions from previous labs and assignments to incorporate into this final product. In addition to the functionalities required in the previous working version of the code, add the following: 1. Class PicrossPuzzle 1. In addition to the solution 2D array, a second 2D array (call it entered) needs to become part of a PicrossPuzzle object. solution holds the key or the secret picture the user is trying to solve; entered holds the current set of inputs entered by the user. solution is filled by information read from a data file, while entered is initially all Os (i.e., user has not clicked on any button yet). Scan through the existing methods and constructors and update any of these where the entered array may need to be initialized or updated. 2. A void toggleCell (int i, int j) method which flips the value in the entered array at row i, column j. That is, if entered[i] j) currently holds a 0, this method turns it into a 1, and vice versa. 3. Aboolean puzzleSolved () method which returns true if the puzzle has been solved, that is, the solution and the entered arrays contain the same exact values at each row and column. If they differ by at least one element, it returns false. 4. Aint[][] getEntered () getter method which returns the entered 2D array; this could be used in the Controller class to collect the entered array and display the puzzle board as game progresses. 5. A void resetEntered () setter method which initializes the entered array back to all 0s.This method will be handy when a user has solved a game, or when the user gives up and chooses to play a new game before the current one is solved. 6. Any additional private and public methods needed. 2. Class PicrossPuzzlePool • No changes needed from the most previous working version (Lab 5). 3. GUI and Controller class 1. Add an event handler so that when one of the grid pane buttons is clicked, the cell changes from white to black and black to white; and also updates(toggles) the array element in the PicrossPuzzle object. 2. Every time a button is clicked, check whether the puzzle has been solved or not. If the puzzle is solved, notify the user using an Alert box with an appropriate message. Displaying the filename when the puzzle is solved is optional. If the puzzle is not solved, the GUI waits for the next button click from the user. 3. Add a new button, Start Over, to allow the user to refresh the entries back to their original state, i.e., resets the game. Note that this does not present a new puzzle but
This is the final version of the Picross code development and your task is to produce a program that will allow the user to solve the puzzle. Feel free to take provided solutions from previous labs and assignments to incorporate into this final product. In addition to the functionalities required in the previous working version of the code, add the following: 1. Class PicrossPuzzle 1. In addition to the solution 2D array, a second 2D array (call it entered) needs to become part of a PicrossPuzzle object. solution holds the key or the secret picture the user is trying to solve; entered holds the current set of inputs entered by the user. solution is filled by information read from a data file, while entered is initially all Os (i.e., user has not clicked on any button yet). Scan through the existing methods and constructors and update any of these where the entered array may need to be initialized or updated. 2. A void toggleCell (int i, int j) method which flips the value in the entered array at row i, column j. That is, if entered[i] j) currently holds a 0, this method turns it into a 1, and vice versa. 3. Aboolean puzzleSolved () method which returns true if the puzzle has been solved, that is, the solution and the entered arrays contain the same exact values at each row and column. If they differ by at least one element, it returns false. 4. Aint[][] getEntered () getter method which returns the entered 2D array; this could be used in the Controller class to collect the entered array and display the puzzle board as game progresses. 5. A void resetEntered () setter method which initializes the entered array back to all 0s.This method will be handy when a user has solved a game, or when the user gives up and chooses to play a new game before the current one is solved. 6. Any additional private and public methods needed. 2. Class PicrossPuzzlePool • No changes needed from the most previous working version (Lab 5). 3. GUI and Controller class 1. Add an event handler so that when one of the grid pane buttons is clicked, the cell changes from white to black and black to white; and also updates(toggles) the array element in the PicrossPuzzle object. 2. Every time a button is clicked, check whether the puzzle has been solved or not. If the puzzle is solved, notify the user using an Alert box with an appropriate message. Displaying the filename when the puzzle is solved is optional. If the puzzle is not solved, the GUI waits for the next button click from the user. 3. Add a new button, Start Over, to allow the user to refresh the entries back to their original state, i.e., resets the game. Note that this does not present a new puzzle but
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
JAVA GUI using scene builder
Share a screenshot of everything
![Instructions Picross 3.0
This is the final version of the Picross code development and your task is to produce a program that will allow the user to solve the puzzle. Feel free to take provided solutions from
previous labs and assignments to incorporate into this final product. In addition to the functionalities required in the previous working version of the code, add the following:
1. Class PicrossPuzzle
1. In addition to the solution 2D array, a second 2D array (call it entered) needs to become part of a PicrossPuzzle object. solution holds the key or the secret
picture the user is trying to solve; entered holds the current set of inputs entered by the user. solution is filled by information read from a data file, while entered
is initially all Os (i.e., user has not clicked on any button yet). Scan through the existing methods and constructors and update any of these where the entered array
may need to be initialized or updated.
2. A void toggleCell(int i, int j) method which flips the value in the entered array at row i, column j. That is, if entered[i][j] currently holds a 0, this
method turns it into a 1, and vice versa.
3. Aboolean puzzleSolved () method which returns true if the puzzle has been solved, that is, the solution and the entered arrays contain the same exact
values at each row and column. If they differ by at least one element, it returns false.
4. Aint [] [] getEntered () getter method which returns the entered 2D array; this could be used in the Controller class to collect the entered array and
display the puzzle board as game progresses.
5. A void resetEntered () setter method which initializes the entered array back to all Os.This method will be handy when a user has solved a game, or when the
user gives up and chooses to play a new game before the current one is solved.
6. Any additional private and public methods needed.
2. Class PicrossPuzzlePool
o No changes needed from the most previous working version (Lab 5).
3. GUI and Controller class
1. Add an event handler so that when one of the grid pane buttons is clicked, the cell changes from white to black and black to white; and also updates(toggles) the array
element in the PicrossPuzzle object.
2. Every time a button is clicked, check whether the puzzle has been solved or not. If the puzzle is solved, notify the user using an Alert box with an appropriate
message. Displaying the filename when the puzzle is solved is optional. If the puzzle is not solved, the GUI waits for the next button click from the user.
3. Add a new button, Start Over, to allow the user to refresh the entries back to their original state, i.e., resets the game. Note that this does not present a new puzzle but
keeps the current puzzle blanking out all previous entries, if any. Add a method to handle this button being clicked.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F01516ebb-d37a-4d91-9729-e5203361ec2a%2F4fbf465d-abad-42d5-abd4-ff309ef62661%2Fm0yiwal_processed.png&w=3840&q=75)
Transcribed Image Text:Instructions Picross 3.0
This is the final version of the Picross code development and your task is to produce a program that will allow the user to solve the puzzle. Feel free to take provided solutions from
previous labs and assignments to incorporate into this final product. In addition to the functionalities required in the previous working version of the code, add the following:
1. Class PicrossPuzzle
1. In addition to the solution 2D array, a second 2D array (call it entered) needs to become part of a PicrossPuzzle object. solution holds the key or the secret
picture the user is trying to solve; entered holds the current set of inputs entered by the user. solution is filled by information read from a data file, while entered
is initially all Os (i.e., user has not clicked on any button yet). Scan through the existing methods and constructors and update any of these where the entered array
may need to be initialized or updated.
2. A void toggleCell(int i, int j) method which flips the value in the entered array at row i, column j. That is, if entered[i][j] currently holds a 0, this
method turns it into a 1, and vice versa.
3. Aboolean puzzleSolved () method which returns true if the puzzle has been solved, that is, the solution and the entered arrays contain the same exact
values at each row and column. If they differ by at least one element, it returns false.
4. Aint [] [] getEntered () getter method which returns the entered 2D array; this could be used in the Controller class to collect the entered array and
display the puzzle board as game progresses.
5. A void resetEntered () setter method which initializes the entered array back to all Os.This method will be handy when a user has solved a game, or when the
user gives up and chooses to play a new game before the current one is solved.
6. Any additional private and public methods needed.
2. Class PicrossPuzzlePool
o No changes needed from the most previous working version (Lab 5).
3. GUI and Controller class
1. Add an event handler so that when one of the grid pane buttons is clicked, the cell changes from white to black and black to white; and also updates(toggles) the array
element in the PicrossPuzzle object.
2. Every time a button is clicked, check whether the puzzle has been solved or not. If the puzzle is solved, notify the user using an Alert box with an appropriate
message. Displaying the filename when the puzzle is solved is optional. If the puzzle is not solved, the GUI waits for the next button click from the user.
3. Add a new button, Start Over, to allow the user to refresh the entries back to their original state, i.e., resets the game. Note that this does not present a new puzzle but
keeps the current puzzle blanking out all previous entries, if any. Add a method to handle this button being clicked.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Recommended textbooks for you
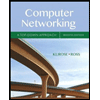
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
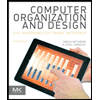
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
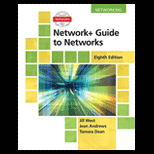
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
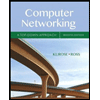
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
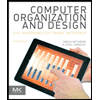
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
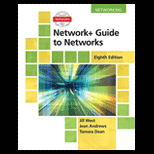
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
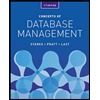
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
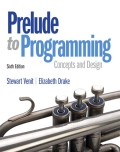
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
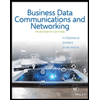
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY