# This program calculates the average of a series of exam scores. |print ("""Calculate the average of a bunch of exam scores. The scores can be integers or floats.""") print () list = [] # initialize variables sum 0.0 count 0.0 avg i = 0 SENTINEL VAL 9999 # input user data inputVal = float(input ("Enter a number. 9999 to quit: ")) # loop continues to iterate until the user enters 9999 while inputVal != 9999: inputVal = float(input("Enter a number. 9999 to quit: ")) if inputVal > 0 and inputVal < 100: list.append (inputVal) else: print ("Score is not in range. Please re-enter.") for i in list: sum = sum + i count = count + 1 # calculating average avg = sum / count # print result print ("These %d scores average as: %.1f" %(count, avg))
Write a
You need to use a while loop to allow the user to enter numbers, one at a time, until some numeric sentinel value is entered. I recommend having a sentinel like 9999, something unlikely to be confused with an exam score. If the user enters a score < 0 or > 100 that is not the sentinel value then that score is to be rejected. Each time a legit score is entered, however, it should be added (appended) to a list.
Once the user has entered all the numbers they want, calculate and display the average of the scores rounded to 1 decimal place.
I attached my solution. I can not fix two problems: 1. It does not count right the number of iterations and avg. 2. After I type 9999(sentinel value) it gives me output: Score is not in range. Please re-enter. It supose just quit and calculate the avg. Thank you!
![# This program calculates the average of a series of exam scores.
|print ("""Calculate the average of a bunch of exam scores.
The scores can be integers or floats.""")
print ()
list = []
# initialize variables
sum
0.0
count
0.0
avg
i = 0
SENTINEL VAL
9999
# input user data
inputVal = float(input ("Enter a number. 9999 to quit: "))
# loop continues to iterate until the user enters 9999
while inputVal != 9999:
inputVal = float(input("Enter a number. 9999 to quit: "))
if inputVal > 0 and inputVal < 100:
list.append (inputVal)
else:
print ("Score is not in range. Please re-enter.")
for i in list:
sum = sum + i
count = count + 1
# calculating average
avg = sum / count
# print result
print ("These %d scores average as: %.1f" %(count, avg))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e48e6c9-94b1-4280-8976-982aad03c6dd%2Fc2a9bc8b-1173-41aa-944f-3bbc584a8de8%2Fy1z9h6e.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

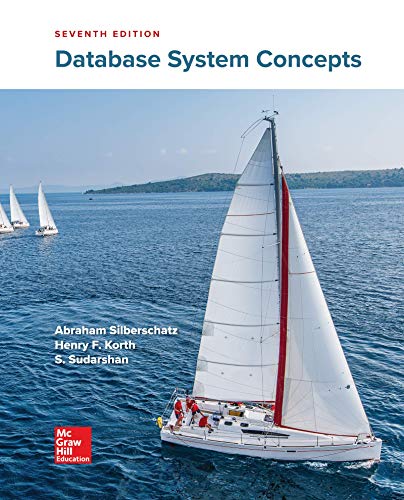
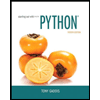
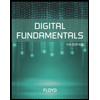
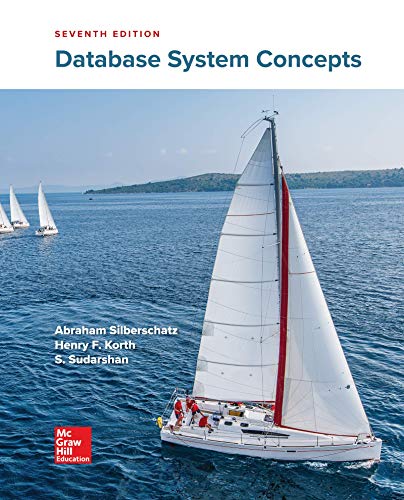
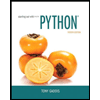
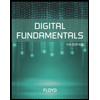
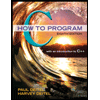
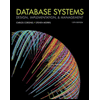
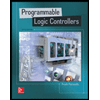