This program will find the standard deviation of a set of How many numbers will you be entering? numbers.
in java
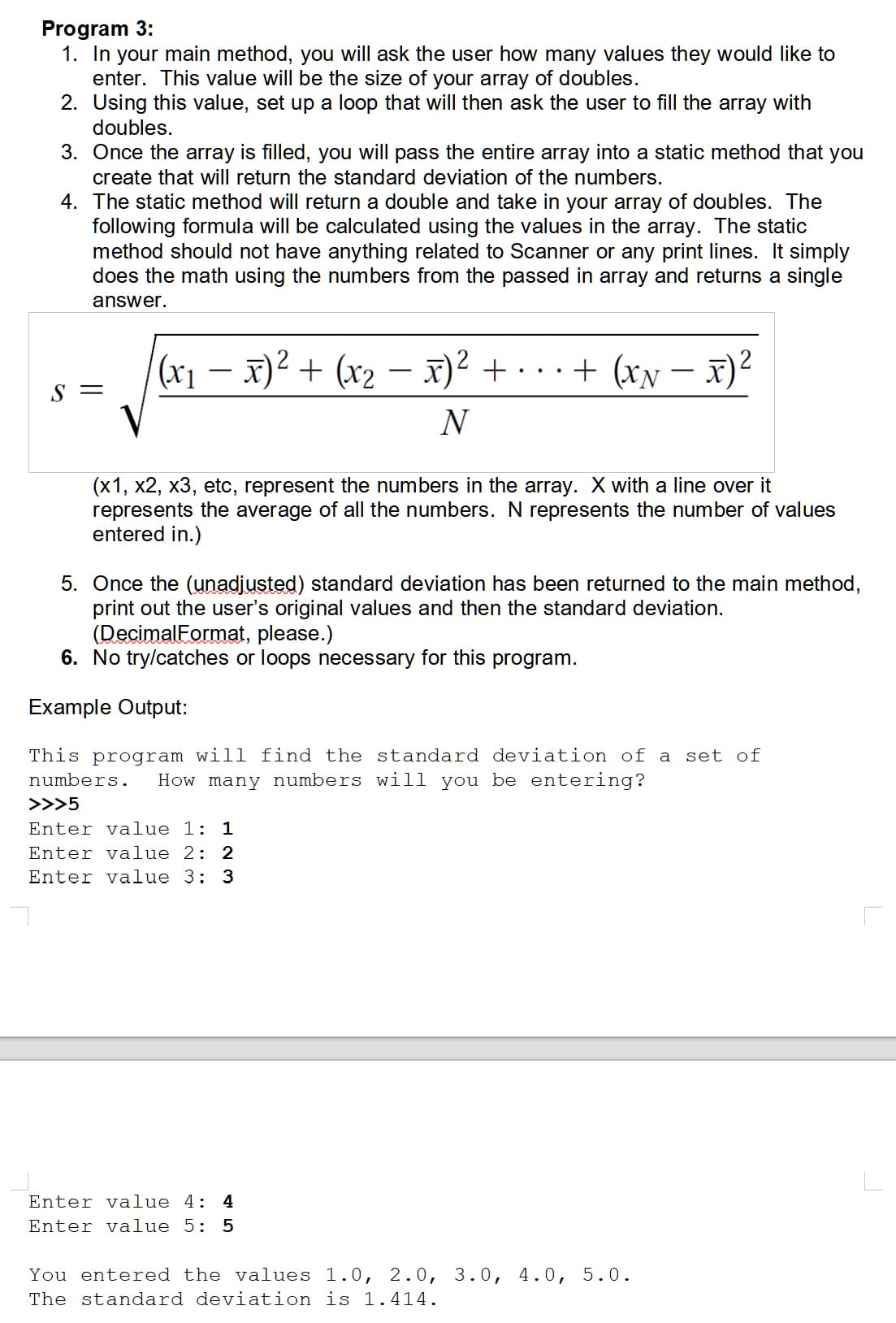

The program of to find standard deviation of entered values by user is below with output.
Program:
import java.util.Scanner; //for input
import java.text.DecimalFormat; //for formatting of decimal numbers
public class StandardDeviation
{
//method to find standard deviation that takes array argument
static double standardDeviation(double[] array){
int n = array.length; //length of the array
double sum = 0;
//loop to find sum of elements in the array
for (int i = 0; i < n; i++){
sum += array[i];
}
double mean = sum / n; //calculating average or mean of the elements of the array
sum = 0;
//loop to find sum of standard deviation elements
for (int i = 0; i < n; i++){
sum += (array[i] - mean) * (array[i] - mean);
}
double standrdDev = Math.sqrt(sum / n); //calculating standard deviation
return standrdDev; //return standard deviation
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); //for input
DecimalFormat format = new DecimalFormat("#.###"); //for formatting 3 decimal places
System.out.println("How many numbers will you be entering?");
int n = sc.nextInt(); //reading how many numbers
double[] values = new double[n]; //creating array
//loop to read number
for (int i = 0; i < n; i++){
System.out.print("Enter value " + (i+1) + ": ");
values[i] = sc.nextDouble(); //reading number by user
}
double standard_deviation = standardDeviation(values); //calling function to calculate standard deviation
System.out.print("\nYou eneterd the values ");
for (int i = 0; i < n; i++){
System.out.print(values[i] + " "); //print values
}
System.out.print("\nThe standard deviation is " + format.format(standard_deviation)); //print standard deviation
}
}
Step by step
Solved in 3 steps with 1 images

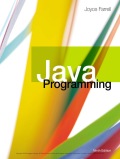
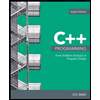
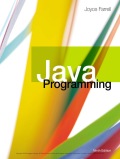
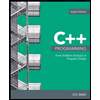