This programming problem deals with circularly-linked queues (CLQueue). You must implement from scratch header file code (CLQueue.h) and .cpp definition code (CLQueue.cpp) to accommodate the circularly linked list representation of a queue which provides efficient enqueueing and dequeueing operations with a linked-list structure. Define and create class declaration file CLQueue.h and CLQueue.cpp with the following guidelines and required operations: You must use the standard, singly linked representation for nodes: struct LNode { int item; //note: do not use a template class for this implementation! LNode* next; }; You may want to revisit the LLUList.h and LLUList.cpp files for examples of how LNode is used. Your actual queue structure should have a single pointer to a LNode, and needs no other elements: class CLQueue { private: LNode* QRear; }; Your queue must be circular. More specifically, QRear should only be NULL if the queue is empty, or otherwise its next pointer must reference the beginning of the queue – permitting fast access to both ends of the queue. You must implement all of the following queue operations for the CLQueue: Enqueue, Dequeue, IsEmpty, MakeEmpty, and appropriate constructor/destructors. It is highly advised that you build your own driver file for testing purposes
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
This
Define and create class declaration file CLQueue.h and CLQueue.cpp with the following guidelines and required operations:
- You must use the standard, singly linked representation for nodes:
struct LNode {
int item; //note: do not use a template class for this implementation!
LNode* next;
};
You may want to revisit the LLUList.h and LLUList.cpp files for examples of how LNode is used. - Your actual queue structure should have a single pointer to a LNode, and needs no other elements:
class CLQueue {
private:
LNode* QRear;
}; - Your queue must be circular. More specifically, QRear should only be NULL if the queue is empty, or otherwise its next pointer must reference the beginning of the queue – permitting fast access to both ends of the queue.
- You must implement all of the following queue operations for the CLQueue: Enqueue, Dequeue, IsEmpty, MakeEmpty, and appropriate constructor/destructors.
- It is highly advised that you build your own driver file for testing purposes

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

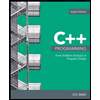
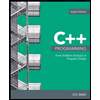