This project utilizes three new classes: · Word - an immutable class representing a word · Words - a class representing a list of Word objects · WordTester - a class used to test the Word and Words classes. WordTester (the application) is complete and only requires uncommenting to test the Word and Words classes as they are completed. The needed imports, class headings, method headings, and block comments are provided for the remaining classes. Javadocs are also provided for the Word and Words classes. Word The Word class represents a single word. It is immutable. You have been provided a skeleton for this class. Complete the Word class using the block comments, Javadocs, and the following instructions. 1. Add a String instance variable named word which will contain the word. 2. Complete the one parameter constructor to initialize the word instance variable. 3. Complete the getLength method to return the number of characters in the word. 4. Complete the getNumVowels method to return the number of vowels in the word. It should use the VOWELS class constant and String’s length, substring, and indexOf methods. Do not use more than one loop. Do not use any String methods that are not in the AP Subset. 5. Complete the getReverse method to return a Word with the letters of this reversed. 6. Complete the toString method to return the String word. 7. Uncomment the Word test code in WordTester and make sure that Word works correctly. Words The Words class represents a list of words. You have been provided a skeleton for this class. Complete the Words class using the block comments, Javadocs, and the following instructions. 1. Add a List instance variable named words which will contain the list of words. Be sure to specify the type of objects that will be stored in the List. 2. Complete the one parameter constructor to initialize the words instance variable. You will need to create the ArrayList and add each word to it. This would be a great place to use a for-each loop. Your code should not generate any warnings. 3. Complete the countWordsWithNumChars method to return the number of words with lengths of num characters. 4. Complete the removeWordsWithNumChars method to remove all words with lengths of num characters. 5. Complete the countWordsWithNumVowels method to return the number of words that have num vowels. 6. Complete the getReversedWords method to return an array of reversed words. 7. Complete the toString method to return the words as a String. You should utilize ArrayLists’s toString method. 8. Uncomment the Words test code in WordTester and make sure that Words works correctly. WORDS CLASS: import java.util.List; import java.util.ArrayList; /** * Words represents a list of Word objects. */ class Words { /** The Words */ /** * Constructs a Words object. * @param wordList the words for the Words object. */ public Words(String[] wordList) { } /** * Counts the number of words with num characters * @param num the number of characters * @return the number of words with num characters */ public int countWordsWithNumChars(int num) { return -999; // Replace } /** * Removes the words with num characters * from this * @param num the number of characters of words to be removed */ public void removeWordsWithNumChars(int num) { } /** * Counts the number of words with num vowels * @param num the number of vowels * @return the number of words with num vowels */ public int countWordsWithNumVowels(int num) { return -999; // Replace } /** * Makes an array containing reversed Word objects. * Each of the items in the returned array is the reverse of an * item in words. The items in the returned array * are in the same order as in words. * @return an array of the reverse objects of words. */ public Word[] getReversedWords() { return null; // Replace } /** * Gives the string representing the words. * @return the string representing the words. */ public String toString() { return null; // Replace } } WORD CLASS: public class Word { private static final String VOWELS = "AEIOUaeiou"; public Word(String w) { } public int getLength() { return -999; // Replace } public int getNumVowels() { return -999; // Replace } public Word getReverse() { return null; // Replace } public String toString() { return null; // Replace } }
This project utilizes three new classes:
· Word - an immutable class representing a word
· Words - a class representing a list of Word objects
· WordTester - a class used to test the Word and Words classes.
WordTester (the application) is complete and only requires uncommenting to test the Word and Words classes as they are completed. The needed imports, class headings, method headings, and block comments are provided for the remaining classes. Javadocs are also provided for the Word and Words classes.
Word
The Word class represents a single word. It is immutable. You have been provided a skeleton for this class. Complete the Word class using the block comments, Javadocs, and the following instructions.
1. Add a String instance variable named word which will contain the word.
2. Complete the one parameter constructor to initialize the word instance variable.
3. Complete the getLength method to return the number of characters in the word.
4. Complete the getNumVowels method to return the number of vowels in the word. It should use the VOWELS class constant and String’s length, substring, and indexOf methods. Do not use more than one loop. Do not use any String methods that are not in the AP Subset.
5. Complete the getReverse method to return a Word with the letters of this reversed.
6. Complete the toString method to return the String word.
7. Uncomment the Word test code in WordTester and make sure that Word works correctly.
Words
The Words class represents a list of words. You have been provided a skeleton for this class. Complete the Words class using the block comments, Javadocs, and the following instructions.
1. Add a List instance variable named words which will contain the list of words. Be sure to specify the type of objects that will be stored in the List.
2. Complete the one parameter constructor to initialize the words instance variable. You will need to create the ArrayList and add each word to it. This would be a great place to use a for-each loop. Your code should not generate any warnings.
3. Complete the countWordsWithNumChars method to return the number of words with lengths of num characters.
4. Complete the removeWordsWithNumChars method to remove all words with lengths of num characters.
5. Complete the countWordsWithNumVowels method to return the number of words that have num vowels.
6. Complete the getReversedWords method to return an array of reversed words.
7. Complete the toString method to return the words as a String. You should utilize ArrayLists’s toString method.
8. Uncomment the Words test code in WordTester and make sure that Words works correctly.
WORDS CLASS:
![Words test2- new words(new String[] {
"fun", "fly", "four", "six", "times", "ten", "plus",
"eight"));
System.out.println("Words test2:+test2);
System.out.println("Number of words with 2 vowels: +
(2));
System.out.println("Number of words with 3 vowels: +
test2.countWordswithNumVowels (3));
System.out.println("Number of words with 4 vowels: *+
test2.countWordswithNumVowels (4));
test2.countWordswithNumVowels
System.out.println("Number of words with 2 chars: "+
test2.countwordskithNunChars (2));
System.out.println("Number of words with 4 chars: "+
test2.countWordswithNunChars (4));
System.out.println("Number of words with 5 chars: "
test2.countWordswithNunChars (5));
test2.removekordsWithNumChars (3);
System.out.println("\nwords test2 after removing words " +
with 3 chars:\"+test2);
System.out.println("\nReversed words: \n" +
System.out.println("\n\n");
Arrays.tostring(test2.getReversedwords()));
Words test3-new words(new String[] {
"alligator", "chicken", "dog", "cat", "pig", "buffalo"));
System.out.println("Words test3:+test);
System.out.println("Number of words with 2 vowels:+
test3.countWordswithNuVowels (2));
System.out.println("Number of words with 3 vowels: +
test3.countWordswithNumVowels (3));
System.out.println("Number of words with 4 vowels: +
test3.countwordswithNueVowels (4));
System.out.println("Number of words with 2 chars: "
test3.countWordswithChars (2));
System.out.println("Number of words with 4
test3.countWordswithNunChars (4));
System.out.println("Number of words with 9 chars:+
test3.countWordswithNunChars (9));
"/
chars: "+
test3.removebordswithusChars (3);
System.out.println("\nWords test3 after removing words " +
"with 3 chars:\n" + test3);
System.out.println("\nReversed words: \n" +
Arrays.tostring(test3.getReversedwords()));](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2711cc56-d6e8-4719-aa28-b183120c8097%2Fdd136944-17c6-4cd4-bdba-367c05bf246a%2Ffxrhsn8_processed.png&w=3840&q=75)
![import java.util.Arrays;
Application to test the <code>Mord</code> and
* <code>Words</code> classes.
"/
public class MordTester
(
public static void main(String[] args)
[
// Test Word
Word one new word("chicken");
System.out.println("Word one: + one);
System.out.println("Number
System.out.println("Number
System.out.println("Reverse:
System.out.println("\n\n");
of chars: "+one.getLength());
of vowels: +one.getNumVowels());
" + one.getReverse());
Word two new Word("alligator");
System.out.println("Word
System.out.println("Number
System.out.println("Number
two: " + two);
of chars:
of vowels:
two.getLength());
two-getNueVowels());
System.out.println("Reverse: "two-getReverse());
System.out.println("\n\n");
Word three new Word("elephant");
System.out.println("Word three: + three);
of chars:
of vowels:
System.out.println("Number
System.out.println("Number
System.out.println("Reverse:
System.out.println("\n\n");
" + three-getReverse());
three.getLength());
three.getNuVowels());
// Test Words
Words testi- new words(new String[] (
"one", "two", "three", "four", "Five", "six", "seven",
"alligator"));
System.out.println("Words testi: " + testi);
System.out.println("Number of words with 2 vowels: +
testi.countWordswithNumVowels (2));
System.out.println("Number of words with 3 vowels: *+
testi.countWordswithNumVowels (3));
System.out.println("Number of words with 4 vowels: +
testi.countWordswithNumVowels (4));
System.out.println("Number of words with 2 chars: a
testi.countWordswithNunChars (2));
System.out.println("Number of words with 4 chars:+
testi.countWordswithChars (4));
System.out.println("Number of words with 5 chars: " +
testi.countWordswithNunChars (5));
testi.removekordsWithNunChars (3);
System.out.println("\nWords testi after removing words +
with 3 chars:\"+testi);
System.out.println("\nReversed words: \n" +
System.out.println("\n\n");
Arrays.tostring(test1.getReversedwords()));](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2711cc56-d6e8-4719-aa28-b183120c8097%2Fdd136944-17c6-4cd4-bdba-367c05bf246a%2Fdtyxdt7_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

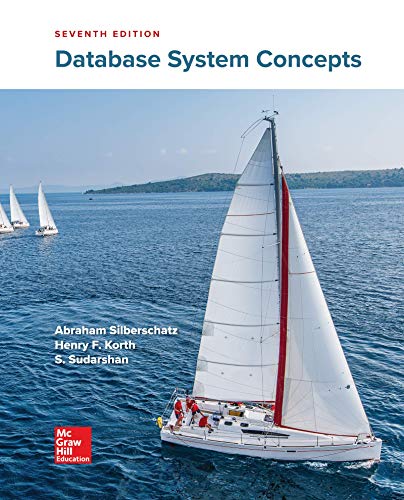
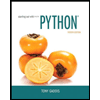
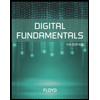
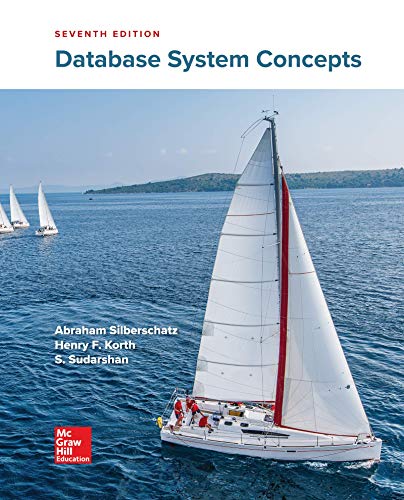
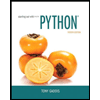
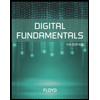
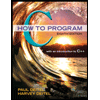
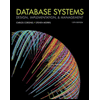
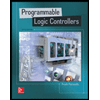