This question involves computing the greatest common factor between two positive integers and using greatest common factors to reduce fractions. You will write two methods in the NumberSystem class that follows. public class NumberSystem { /** Precondition: a and b are positive integers. *Returns the greatest common factor of a and b, as described in part (a). public static int gcf(int a, int b) { / to be implemented in part (a) */ } /** Precondition: numerator and denominator are positive integers. *Reduces the fraction numerator / denominator * and prints the result, as described in part (b). */ public static void reduceFraction(int numerator, int denominator) { / to be implemented in part (b) */ } } The greatest common factor (GCF) of two integers a and b is the largest integer that divides evenly into both a and b. For example, the GCF of 8 and 12 is 4. The greatest common factor can be computed using the following rules. Case I: If a is evenly divisible by b, then the GCF is b. Case II: If a is not evenly divisible by b, then the GCF of a and b is equal to the GCF of b and the remainder when a is divided by b. If the rule in case II is repeatedly applied, it is guaranteed to eventually result in case I. Consider the following examples. Example 1 In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. Therefore, the GCF of 30 and 3 is 3. Example 2 In determining the GCF of 3 and 30, case Il applies because 3 is not evenly divisible by 30. The GCF of 3 and 30 will be equal to the GCF of 30 and the remainder when 3 is divided by 30, or 3. In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. The GCF of 30 and 3 is 3, and therefore the GCF of 3 and 30 is also 3. Example 3 In determining the GCF of 24 and 9, case Il applies because 24 is not evenly divisible by 9. The GCF of 24 and 9 will be equal to the GCF of 9 and the remainder when 24 is divided by 9, or 6. In determining the GCF of 9 and 6, case Il applies because 9 is not evenly divisible by 6. The GCF of 9 and 6 will be equal to the GCF of 6 and the remainder when 9 is divided by 6, or 3. In determining the GCF of 6 and 3, case I applies because 6 is evenly divisible by 3. The GCF of 6 and 3 is 3, and therefore the GCF of 24 and 9 is also 3. Example 4 In determining the GCF of 7 and 3, case Il applies because 7 is not evenly divisible by 3. The GCF of 7 and 3 will be equal to the GCF of 3 and the remainder when 7 is divided by 3, or 1. In determining the GCF of 3 and 1, case I applies because 3 is evenly divisible by 1. The GCF of 3 and 1 is 1, and therefore the GCF of 7 and 3 is also 1. (a) The gcf method returns the greatest common factor of parameters a and b, as determined by case I and case II. Write the gcf method below. You are encouraged to implement this method recursively. /* Precondition: a and b are positive integers. * Returns the greatest common factor of a and b, as described in part (a). */ public static int gcf(int a, int b)
This question involves computing the greatest common factor between two positive integers and using greatest common factors to reduce fractions. You will write two methods in the NumberSystem class that follows. public class NumberSystem { /** Precondition: a and b are positive integers. *Returns the greatest common factor of a and b, as described in part (a). public static int gcf(int a, int b) { / to be implemented in part (a) */ } /** Precondition: numerator and denominator are positive integers. *Reduces the fraction numerator / denominator * and prints the result, as described in part (b). */ public static void reduceFraction(int numerator, int denominator) { / to be implemented in part (b) */ } } The greatest common factor (GCF) of two integers a and b is the largest integer that divides evenly into both a and b. For example, the GCF of 8 and 12 is 4. The greatest common factor can be computed using the following rules. Case I: If a is evenly divisible by b, then the GCF is b. Case II: If a is not evenly divisible by b, then the GCF of a and b is equal to the GCF of b and the remainder when a is divided by b. If the rule in case II is repeatedly applied, it is guaranteed to eventually result in case I. Consider the following examples. Example 1 In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. Therefore, the GCF of 30 and 3 is 3. Example 2 In determining the GCF of 3 and 30, case Il applies because 3 is not evenly divisible by 30. The GCF of 3 and 30 will be equal to the GCF of 30 and the remainder when 3 is divided by 30, or 3. In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. The GCF of 30 and 3 is 3, and therefore the GCF of 3 and 30 is also 3. Example 3 In determining the GCF of 24 and 9, case Il applies because 24 is not evenly divisible by 9. The GCF of 24 and 9 will be equal to the GCF of 9 and the remainder when 24 is divided by 9, or 6. In determining the GCF of 9 and 6, case Il applies because 9 is not evenly divisible by 6. The GCF of 9 and 6 will be equal to the GCF of 6 and the remainder when 9 is divided by 6, or 3. In determining the GCF of 6 and 3, case I applies because 6 is evenly divisible by 3. The GCF of 6 and 3 is 3, and therefore the GCF of 24 and 9 is also 3. Example 4 In determining the GCF of 7 and 3, case Il applies because 7 is not evenly divisible by 3. The GCF of 7 and 3 will be equal to the GCF of 3 and the remainder when 7 is divided by 3, or 1. In determining the GCF of 3 and 1, case I applies because 3 is evenly divisible by 1. The GCF of 3 and 1 is 1, and therefore the GCF of 7 and 3 is also 1. (a) The gcf method returns the greatest common factor of parameters a and b, as determined by case I and case II. Write the gcf method below. You are encouraged to implement this method recursively. /* Precondition: a and b are positive integers. * Returns the greatest common factor of a and b, as described in part (a). */ public static int gcf(int a, int b)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Questions are in the attached pictures!
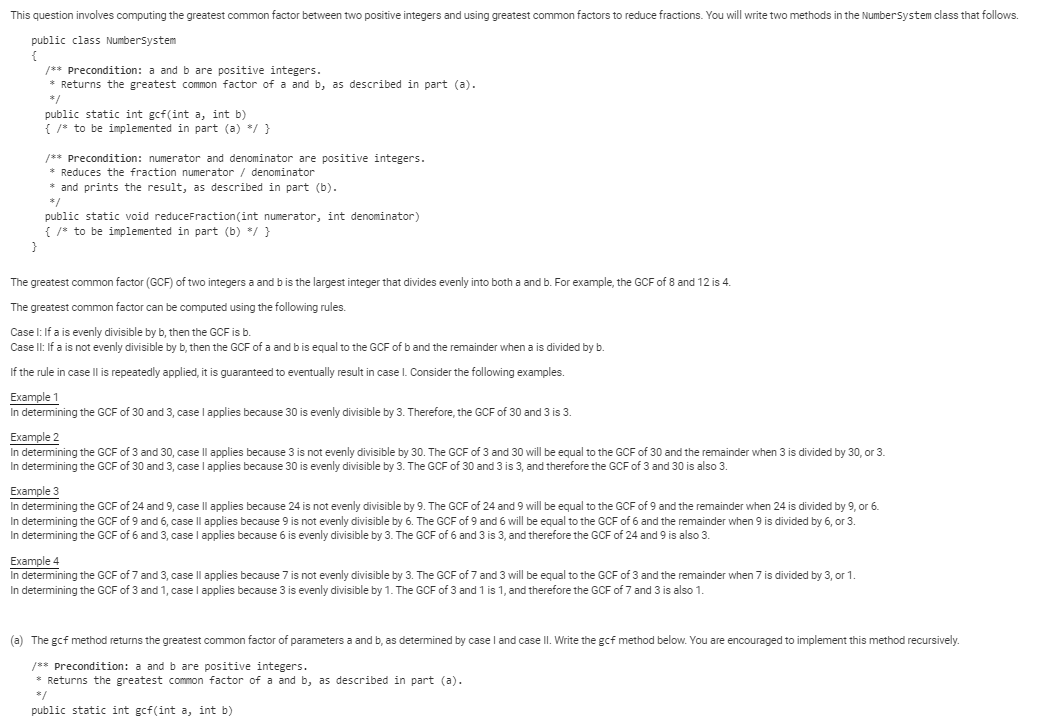
Transcribed Image Text:This question involves computing the greatest common factor between two positive integers and using greatest common factors to reduce fractions. You will write two methods in the NumberSystem class that follows.
public class NumberSystem
{
/** Precondition: a and b are positive integers.
* Returns the greatest common factor of a and b, as described in part (a).
*/
public static int gcf(int a, int b)
{ /* to be implemented in part (a) */ }
/** Precondition: numerator and denominator are positive integers.
* Reduces the fraction numerator / denominator
* and prints the result, as described in part (b).
public static void reduceFraction(int numerator, int denominator)
{ /* to be implemented in part (b) */ }
}
The greatest common factor (GCF) of two integers a and b is the largest integer that divides evenly into both a and b. For example, the GCF of 8 and 12 is 4.
The greatest common factor can be computed using the following rules.
Case I: If a is evenly divisible by b, then the GCF is b.
Case II: If a is not evenly divisible by b, then the GCF of a and b is equal to the GCF of b and the remainder when a is divided by b.
If the rule in case II is repeatedly applied, it is guaranteed to eventually result in case I. Consider the following examples.
Example 1
In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. Therefore, the GCF of 30 and 3 is 3.
Example 2
In determining the GCF of 3 and 30, case II applies because 3 is not evenly divisible by 30. The GCF of 3 and 30 will be equal to the GCF of 30 and the remainder when 3 is divided by 30, or 3.
In determining the GCF of 30 and 3, case I applies because 30 is evenly divisible by 3. The GCF of 30 and 3 is 3, and therefore the GCF of 3 and 30 is also 3.
Example 3
In determining the GCF of 24 and 9, case II applies because 24 is not evenly divisible by 9. The GCF of 24 and 9 will be equal to the GCF of 9 and the remainder when 24 is divided by 9, or 6.
In determining the GCF of 9 and 6, case Il applies because 9 is not evenly divisible by 6. The GCF of 9 and 6 will be equal to the GCF of 6 and the remainder when 9 is divided by 6, or 3.
In determining the GCF of 6 and 3, case I applies because 6 is evenly divisible by 3. The GCF of 6 and 3 is 3, and therefore the GCF of 24 and 9 is also 3.
Example 4
In determining the GCF of 7 and 3, case II applies because 7 is not evenly divisible by 3. The GCF of 7 and 3 will be equal to the GCF of 3 and the remainder when 7 is divided by 3, or 1.
In determining the GCF of 3 and 1, case I applies because 3 is evenly divisible by 1. The GCF of 3 and 1 is 1, and therefore the GCF of 7 and 3 is also 1.
(a) The gcf method returns the greatest common factor of parameters a and b, as determined by case I and case II. Write the gcf method below. You are encouraged to implement this method recursively.
/** Precondition: a and b are positive integers.
* Returns the greatest common factor of a and b, as described in part (a).
3/
public static int gcf(int a, int b)
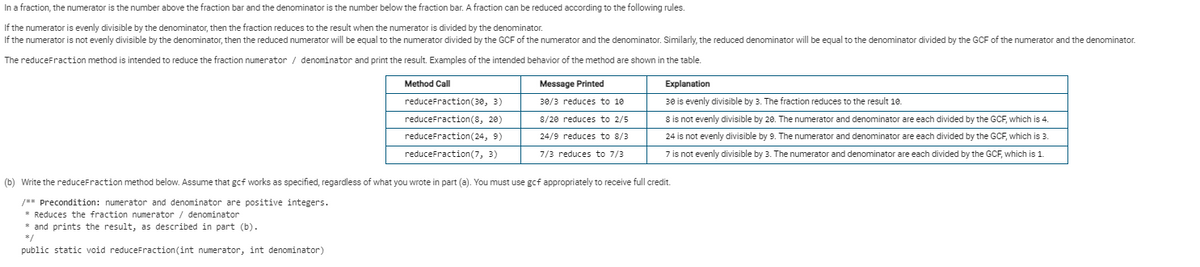
Transcribed Image Text:In a fraction, the numerator is the number above the fraction bar and the denominator is the number below the fraction bar. A fraction can be reduced according to the following rules.
If the numerator is evenly divisible by the denominator, then the fraction reduces to the result when the numerator is divided by the denominator.
If the numerator is not evenly divisible by the denominator, then the reduced numerator will be equal to the numerator divided by the GCF of the numerator and the denominator. Similarly, the reduced denominator will be equal to the denominator divided by the GCF of the numerator and the denominator.
The reduceFraction method is intended to reduce the fraction numerator / denominator and print the result. Examples of the intended behavior of the method are shown in the table.
Method Call
reduceFraction (30, 3)
reduceFraction (8, 20)
reduceFraction (24, 9)
reduceFraction (7,3)
* and prints the result, as described in part (b).
3/
public static void reduceFraction(int numerator, int denominator)
Message Printed
30/3 reduces to 10
8/20 reduces to 2/5
24/9 reduces to 8/3
7/3 reduces to 7/3
Explanation
30 is evenly divisible by 3. The fraction reduces to the result 10.
8 is not evenly divisible by 20. The numerator and denominator are each divided by the GCF, which is 4.
24 is not evenly divisible by 9. The numerator and denominator are each divided by the GCF, which is 3.
7 is not evenly divisible by 3. The numerator and denominator are each divided by the GCF, which is 1.
(b) Write the reduceFraction method below. Assume that gcf works as specified, regardless of what you wrote in part (a). You must use gcf appropriately to receive full credit.
/** Precondition: numerator and denominator are positive integers.
* Reduces the fraction numerator / denominator
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 4 images

Recommended textbooks for you
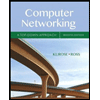
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
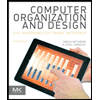
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
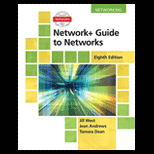
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
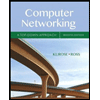
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
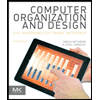
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
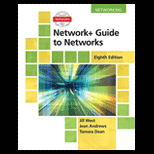
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
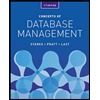
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
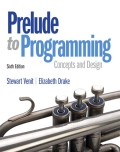
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
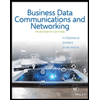
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY