This question is about vectors. In this question you will write a program with two functions. You must also write the #include statements. In your main() function you will create two vectors which will store integers and strings respectively. After that your program must read user inputs from the console. If the input is a string you should append it to the string vector. If the input is an integer, you should append it to the integer vector. You cannot use the strutils library. You cannot use the prompt library. The range of the integers is [-10, 10]. If the user input exceeds this range, you should warn the user and skip that input. There are no restrictions on the string inputs. Your program should stop collecting user inputs, when the user inputs the word STOP (case-sensitive). After that point you should send your int vector as a reference parameter to a non-void function that will find the biggest sum within the vector. If the vector is empty, your function should return 0. Else if the vector is not empty, your function should return the sum of all positive values: If vector: [-5, -1, -3], return: 0 If vector: [-3, 2, 8], return: 10 If vector: [0, 4, 1], return: 5 Once this value is returned to the main() function, your program should call a second void function, which will take the string vector and the biggest sum value as parameters and find the words that are longer than the biggest sum (say bigSum). All words that are longer than bigSum should be printed onto the console on separate lines. If the string vector is empty your function should print an error message stating that the vector is empty. If the string vector has strings that are shorter than bigSum, then your function should print an error message stating that no strings were found longer than the biggest sum value. Below you can find sample runs:
This question is about
In your main() function you will create two vectors which will store integers and strings respectively. After that your program must read user inputs from the console.
- If the input is a string you should append it to the string vector.
- If the input is an integer, you should append it to the integer vector.
- You cannot use the strutils library.
- You cannot use the prompt library.
The range of the integers is [-10, 10]. If the user input exceeds this range, you should warn the user and skip that input.
There are no restrictions on the string inputs.
Your program should stop collecting user inputs, when the user inputs the word STOP (case-sensitive).
After that point you should send your int vector as a reference parameter to a non-void function that will find the biggest sum within the vector.
- If the vector is empty, your function should return 0.
- Else if the vector is not empty, your function should return the sum of all positive values:
- If vector: [-5, -1, -3], return: 0
- If vector: [-3, 2, 8], return: 10
- If vector: [0, 4, 1], return: 5
Once this value is returned to the main() function, your program should call a second void function, which will take the string vector and the biggest sum value as parameters and find the words that are longer than the biggest sum (say bigSum). All words that are longer than bigSum should be printed onto the console on separate lines.
- If the string vector is empty your function should print an error message stating that the vector is empty.
- If the string vector has strings that are shorter than bigSum, then your function should print an error message stating that no strings were found longer than the biggest sum value.
Below you can find sample runs:
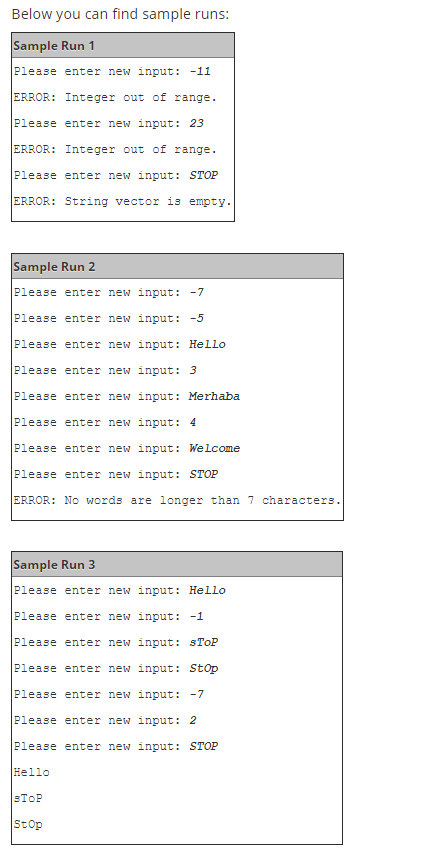

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

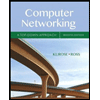
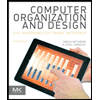
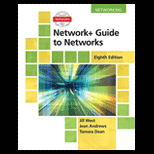
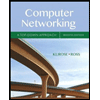
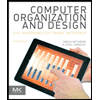
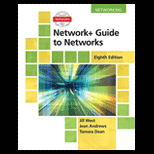
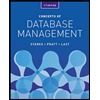
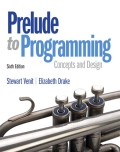
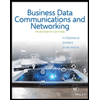